解释下列代码#include <iostream> #include <string> using namespace std; class Date { public: Date(int year, int month, int day) : year_(year), month_(month), day_(day) {}; friend ostream& operator<<(ostream& os, const Date& date) { os << date.year_ << "-" << date.month_ << "-" << date.day_; return os; } private: int year_; int month_; int day_; }; class Person { public: Person(const string& name, const Date& birthday) : name_(name), birthday_(birthday) {}; virtual ~Person() {}; protected: string name_; Date birthday_; }; class Teacher : public Person { public: Teacher(const string& id, const string& name, const Date& birthday, const string& major, const string& affiliation) : Person(name, birthday), id_(id), major_(major), affiliation_(affiliation) {}; void PrintInfo() const { cout << "Name: " << name_ << endl; cout << "Birthday: " << birthday_ << endl; cout << "Teacher ID: " << id_ << endl; cout << "Teaching Major: " << major_ << endl; cout << "Affiliation: " << affiliation_ << endl; cout << "The basic information: " << id_ << ' ' << name_ << ' ' << birthday_ << ' ' << major_ << ' ' << affiliation_ << endl; } private: string id_; string major_; string affiliation_; }; class Student : public Person { public: Student(const string& id, const string& name, int score, const Date& birthday) : Person(name, birthday), id_(id), score_(score) {}; void PrintInfo() const { cout << "Name: " << name_ << endl; cout << "Birthday: " << birthday_ << endl; cout << "Student ID: " << id_ << endl; cout << "Student Score: " << score_ << endl; cout << "The basic information: " << id_ << ' ' << name_ << ' ' << score_ << endl; cout << birthday_ << endl; } private: string id_; int score_; }; int main() { Date student_birthday(1976, 5, 27); //修改学生出生日期 Student student("2023007", "kxiong", 92, student_birthday); student.PrintInfo(); Date teacher_birthday(1998, 1, 7); //修改教师出生日期 Teacher teacher("20210058", "xsong", teacher_birthday, "Computer Science", "CTBu"); teacher.PrintInfo(); return 0; }
时间: 2024-03-11 07:44:42 浏览: 75
这段代码定义了四个类:Date、Person、Teacher和Student。其中,Date类表示日期,Person类表示人,Teacher和Student是Person的两个派生类,表示教师和学生。每个类都有一些成员变量和成员函数。
在主函数中,首先创建了一个学生对象,然后修改了学生的出生日期,并调用PrintInfo函数打印学生的信息。接着,创建了一个教师对象,修改了教师的出生日期,并调用PrintInfo函数打印教师的信息。
在Person类中,定义了一个纯虚析构函数,表示Person类是一个抽象类,不能直接创建Person对象,只能创建派生类的对象。在Teacher和Student类中,分别定义了PrintInfo函数,用于打印教师和学生的信息。在Date类中,重载了<<运算符,用于打印日期信息。
相关问题
请设计日期 DATE 类。它包含三个私有数据成员:year (年)、month (月) 和 day (天),均为整数。 #include <iostream> using namespace std
为了设计DATE类,我们可以创建一个名为DATE的结构体或者类,包含year、month和day三个私有成员变量。这里以类的形式展示,因为类更便于封装和操作:
```cpp
class DATE {
private:
int year;
int month;
int day;
public:
// 构造函数,用于初始化日期
DATE(int y = 0, int m = 0, int d = 0) : year(y), month(m), day(d) {}
// 获取和设置年份
int getYear() const { return year; }
void setYear(int new_year) { year = new_year; }
// 获取和设置月份
int getMonth() const { return month; }
void setMonth(int new_month) { month = new_month; }
// 获取和设置日期
int getDay() const { return day; }
void setDay(int new_day) { day = new_day; }
// 其他实用方法,如验证日期是否合法等
// 指定格式打印日期
void printDate() const {
cout << "Date: " << year << "/" << month << "/" << day << endl;
}
// 根据输入字符串解析日期
bool parseString(const string& date_str) {
char delimiter = '/';
size_t pos = 0;
if (!(pos = date_str.find(delimiter))) {
return false;
}
int parsed_year = stoi(date_str.substr(0, pos));
if (parsed_year < 1 || parsed_year > 9999) {
return false;
}
pos += delimiter == '-' ? 1 : 2; // Adjust for possible - instead of /
int parsed_month = stoi(date_str.substr(pos, pos + 2));
if (parsed_month < 1 || parsed_month > 12) {
return false;
}
pos += 2;
int parsed_day = stoi(date_str.substr(pos));
if (parsed_day < 1 || parsed_day > 31) {
return false;
}
setYear(parsed_year);
setMonth(parsed_month);
setDay(parsed_day);
return true;
}
// 其他可能的日期运算方法,例如比较、加减日期等
};
```
#include <iostream> using namespace std; class Date { public: int year,month,day; Date(int year=2000,int month=1,int day=1):year(year),month(month),day(day) {} }; class Cperson { private: string Name; string Num; Date brith; Cperson(const char *pName="张三", string Num="123456789123456789", int year=2000,int month=1,int day=1): Name(Name),Num(Num),brith(year,month,day) {} string GetName() { return Name; } string GetNum() { return Num; } int Getbrith() { return brith; } }; cass Cstudent: private Cperson { private: int StNum; }; class Cteacher:public Cperson { private: Date Time; }; int main() { cout << "手机编程APP"; return 0; }有什么错误
在类Cperson的构造函数中,初始化成员变量时,应该使用初始化列表中的参数,而不是使用类内部定义的变量。正确的构造函数应该是:
```
Cperson(const char *pName="张三",
string Num="123456789123456789",
int year=2000,int month=1,int day=1):
Name(pName),Num(Num),brith(year,month,day)
{}
```
此外,在类Cperson的成员函数Getbrith中,返回值应该是一个Date对象,而不是int类型。应该改为:
```
Date Getbrith()
{
return brith;
}
```
最后,在类Cstudent和Cteacher中,应该声明访问权限为public,否则外部无法访问这些类的成员。修正后的代码如下:
```
#include <iostream>
using namespace std;
class Date {
public:
int year,month,day;
Date(int year=2000,int month=1,int day=1):year(year),month(month),day(day)
{}
};
class Cperson {
public:
string Name;
string Num;
Date brith;
Cperson(const char *pName="张三",
string Num="123456789123456789",
int year=2000,int month=1,int day=1):
Name(pName),Num(Num),brith(year,month,day)
{}
string GetName()
{
return Name;
}
string GetNum()
{
return Num;
}
Date Getbrith()
{
return brith;
}
};
class Cstudent: public Cperson {
public:
int StNum;
};
class Cteacher:public Cperson {
private:
Date Time;
public:
// 省略构造函数
};
int main() {
cout << "手机编程APP";
return 0;
}
```
阅读全文
相关推荐
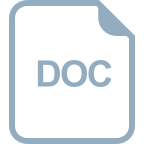
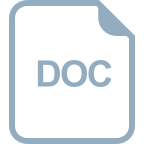
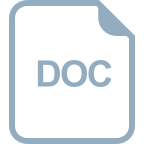













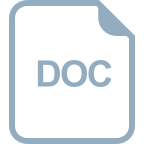