用java 设计一个文件管理系统 并可以完成剪切 复制和删除文件功能
时间: 2024-02-09 07:08:05 浏览: 80
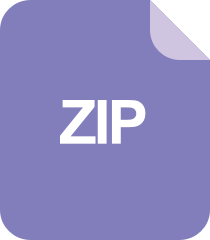
基于java安卓开发的文件管理器+源码+界面演示+项目文档(毕业设计&课程设计&项目开发)
好的,我可以为您提供一个简单的Java代码,用于实现文件管理系统。该系统可以执行剪切、复制和删除文件的功能。以下是代码:
```java
import java.io.*;
public class FileManager {
// 剪切文件
public static void cutFile(String sourceFilePath, String destinationFilePath) throws IOException {
File sourceFile = new File(sourceFilePath);
File destinationFile = new File(destinationFilePath);
if (sourceFile.exists()) {
InputStream in = new FileInputStream(sourceFile);
OutputStream out = new FileOutputStream(destinationFile);
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
sourceFile.delete();
System.out.println("文件剪切成功!");
} else {
System.out.println("文件不存在!");
}
}
// 复制文件
public static void copyFile(String sourceFilePath, String destinationFilePath) throws IOException {
File sourceFile = new File(sourceFilePath);
File destinationFile = new File(destinationFilePath);
if (sourceFile.exists()) {
InputStream in = new FileInputStream(sourceFile);
OutputStream out = new FileOutputStream(destinationFile);
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
System.out.println("文件复制成功!");
} else {
System.out.println("文件不存在!");
}
}
// 删除文件
public static void deleteFile(String filePath) {
File file = new File(filePath);
if (file.exists()) {
file.delete();
System.out.println("文件删除成功!");
} else {
System.out.println("文件不存在!");
}
}
}
```
上述代码使用Java的IO流操作,实现了剪切、复制和删除文件的功能。您只需要调用相应的方法并传入文件路径,即可完成对应的文件操作。
阅读全文
相关推荐
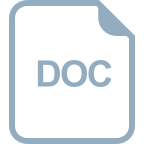
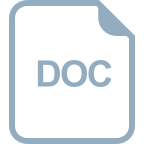
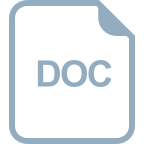

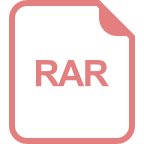
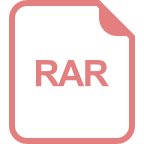
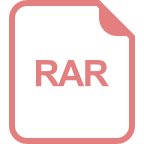
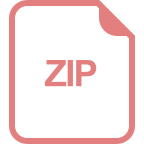
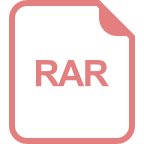
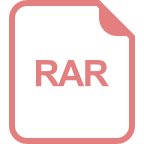
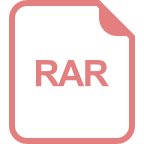
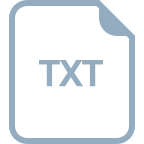
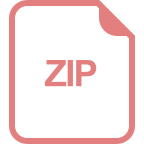
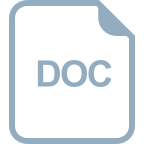
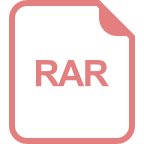
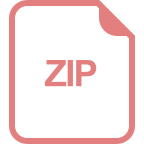
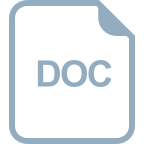
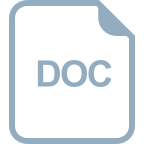