在Qt Creator中创建一个列表,每行有3列數據,每頁只能放10行,并实现翻页效果的代碼
时间: 2024-03-21 17:37:36 浏览: 93
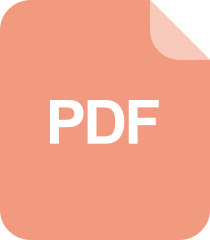
Qt 翻页效果实现~

以下是在Qt Creator中使用QListWidget实现翻页效果的代码:
```
// 定义全局变量
const int PAGE_SIZE = 10; // 每页显示的行数
int currentPage = 0; // 当前页码
// 创建列表控件
QListWidget *listWidget = new QListWidget(this);
listWidget->setViewMode(QListWidget::ListMode);
listWidget->setSelectionMode(QAbstractItemView::NoSelection);
listWidget->setResizeMode(QListWidget::Adjust);
// 添加数据
for (int i = 0; i < 30; i++) {
QString text = QString("Item %1").arg(i + 1);
QListWidgetItem *item = new QListWidgetItem(text);
item->setData(Qt::UserRole, i); // 存储数据,此处为行号
listWidget->addItem(item);
}
// 显示第一页
for (int i = 0; i < PAGE_SIZE; i++) {
listWidget->item(i)->setHidden(false);
}
currentPage = 1;
// 翻页按钮的槽函数
void on_btnNext_clicked() {
int totalPage = qCeil(listWidget->count() / (double)PAGE_SIZE); // 总页数
if (currentPage >= totalPage) {
return;
}
int start = currentPage * PAGE_SIZE;
int end = (currentPage + 1) * PAGE_SIZE - 1;
for (int i = start; i <= end; i++) {
listWidget->item(i)->setHidden(false);
}
start = (currentPage - 1) * PAGE_SIZE;
end = currentPage * PAGE_SIZE - 1;
for (int i = 0; i < start; i++) {
listWidget->item(i)->setHidden(true);
}
for (int i = end + 1; i < listWidget->count(); i++) {
listWidget->item(i)->setHidden(true);
}
currentPage++;
}
void on_btnPrev_clicked() {
if (currentPage <= 1) {
return;
}
int start = (currentPage - 2) * PAGE_SIZE;
int end = (currentPage - 1) * PAGE_SIZE - 1;
for (int i = start; i <= end; i++) {
listWidget->item(i)->setHidden(false);
}
start = (currentPage - 1) * PAGE_SIZE;
end = currentPage * PAGE_SIZE - 1;
for (int i = end + 1; i < listWidget->count(); i++) {
listWidget->item(i)->setHidden(true);
}
for (int i = 0; i < start; i++) {
listWidget->item(i)->setHidden(true);
}
currentPage--;
}
```
在代码中,我们使用QListWidget来创建列表控件,并且设置了其显示模式、选择模式和自动调整大小模式。然后,我们添加了30个条目,每个条目都存储一个数据(此处为行号)。
在翻页按钮的槽函数中,我们首先计算出总页数和当前页码。然后,根据当前页码计算出需要显示的条目的行号范围,并将这些条目设置为可见;同时,将不需要显示的条目设置为隐藏。
在上一页的槽函数中,我们使用与下一页类似的逻辑,只是将需要显示的条目的行号范围计算方式有所不同。
需要注意的是,我们使用了qCeil函数来计算总页数,这是因为当总条目数不能被每页显示的行数整除时,需要多出一页来显示剩余的条目。
阅读全文
相关推荐
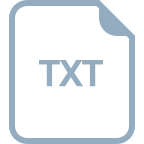
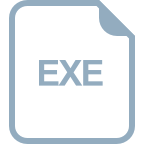
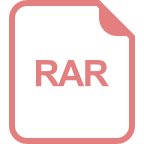
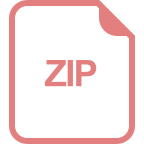
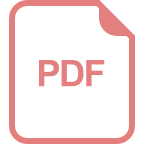
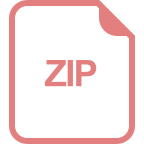
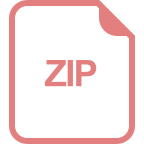
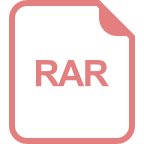
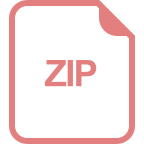
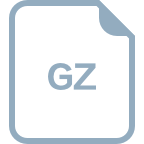
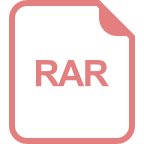
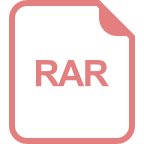
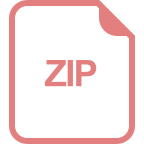
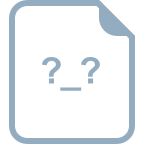
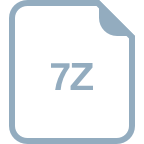