python 如何将str类型"[{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}]" 转为包含两个字典项的列表
时间: 2024-01-09 20:04:10 浏览: 67
可以使用`ast.literal_eval()`函数将字符串转换为列表。
示例代码如下:
```python
import ast
s = "[{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}]"
lst = ast.literal_eval(s)
print(lst)
```
输出结果为:
```
[{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}]
```
这样就将字符串转换为了包含两个字典项的列表。
相关问题
将结果显示为 [ {"name": "Alice", "age": 25}, {"name": "Bob", "age": 35}, {"name": "John", "age": 30} ]
要将结果显示为指定格式的多行字符串,可以使用 `json.dumps()` 方法并设置 `indent` 参数为指定的缩进值。
以下是一个示例代码,将结果显示为指定的格式:
```python
import json
sorted_data = [
{"name": "Alice", "age": 25},
{"name": "Bob", "age": 35},
{"name": "John", "age": 30}
]
json_str = json.dumps(sorted_data, indent=4)
print(json_str)
```
输出结果:
```json
[
{
"name": "Alice",
"age": 25
},
{
"name": "Bob",
"age": 35
},
{
"name": "John",
"age": 30
}
]
```
在上述示例中,我们将 `indent` 参数设置为 `4`,这样 JSON 字符串将会进行缩进,并且每个字典都会单独占据一行。这样打印出的 JSON 字符串将会以多行形式显示,且与你所期望的格式一致。
r循环遍历数据对象astr = 'hello'alist = [10, 20, 30]atuple = ('bob', 'tom', 'alice')adict = {'name': 'john', 'age': 23}for ch in astr: print(ch)
r循环遍历数据对象是指在Python中使用`for`循环逐个处理字符串、列表、元组和字典等不同类型的数据结构中的元素。在这个例子中:
```python
astr = 'hello'
alist = [10, 20, 30]
atuple = ('bob', 'tom', 'alice')
adict = {'name': 'john', 'age': 23}
# 对于字符串astr,我们按字符遍历
for ch in astr:
print(ch) # 输出每个字符,分别是'h', 'e', 'l', 'l', 'o'
# 对于列表alist,我们遍历的是元素的索引位置,可以访问到数值
for i in alist:
print(i) # 输出10, 20, 30
# 对于元组atuple,同样遍历元素
for item in atuple:
print(item) # 输出'bob', 'tom', 'alice'
# 对于字典adict,遍历的是键值对,这里使用items()方法
for key, value in adict.items():
print(f"{key}: {value}") # 输出'name: john', 'age: 23'
```
阅读全文
相关推荐
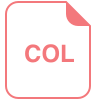
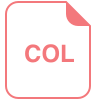
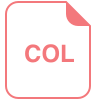
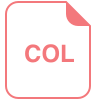
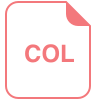
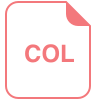
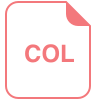
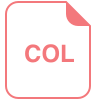
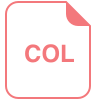
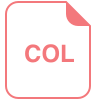
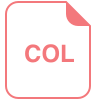
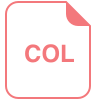
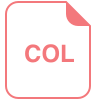
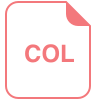