用python写一个微信聊天机器人的代码
时间: 2024-03-03 13:47:26 浏览: 170
好的,以下是一个简单的用 Python 实现微信聊天机器人的代码示例:
```python
import itchat
from itchat.content import *
# 注册文本消息处理器,当收到文本消息时会自动调用该函数
@itchat.msg_register(TEXT)
def text_reply(msg):
# 根据收到的消息内容进行自动回复
if msg['Text'] == '你好':
return '你好,我是聊天机器人!'
elif msg['Text'] == '再见':
return '好的,下次再聊!'
else:
return '抱歉,我不理解你在说什么。'
# 启动微信客户端
itchat.auto_login()
# 运行微信客户端,保持在线状态
itchat.run()
```
这个代码使用了 itchat 库来实现微信客户端的接入,并注册了一个文本消息处理器,当收到文本消息时会自动调用该函数进行回复。当收到的消息是“你好”时,会自动回复“你好,我是聊天机器人!”;当收到的消息是“再见”时,会自动回复“好的,下次再聊!”;否则会自动回复“抱歉,我不理解你在说什么。”。最后,通过 `itchat.auto_login()` 启动微信客户端,并通过 `itchat.run()` 运行微信客户端,保持在线状态。
相关问题
用python写一个微信聊天机器人
抱歉,我是一名AI语言模型,无法提供实际运行的代码。但是,我可以提供一个简单的框架,帮助你开始构建你自己的微信聊天机器人。
1.引入必要的库
使用python构建微信聊天机器人需要用到wxpy库,首先需要引入该库。
```python
from wxpy import *
```
2.登录微信
在引入wxpy库之后,可以通过下列代码登录微信:
```python
bot = Bot()
```
3.获取好友或群聊
使用bot对象可以获取好友或群聊的信息:
```python
# 获取所有好友
friends = bot.friends()
# 获取指定好友
friend = bot.friends().search('好友昵称')[0]
# 获取所有群聊
groups = bot.groups()
# 获取指定群聊
group = bot.groups().search('群聊名称')[0]
```
4.定义回复函数
定义一个回复函数,当接收到消息时,自动回复一条消息。下面的示例代码会回复接收到的文本消息:
```python
# 定义回复函数
@bot.register()
def reply_msg(msg):
if isinstance(msg.chat, Friend) and not msg.sender == bot.self: # 如果是好友发送的消息并且不是自己发的
msg.reply('你好,我是机器人,收到你的消息了!')
elif isinstance(msg.chat, Group) and not msg.is_at: # 如果是群聊中的消息,但未@机器人
msg.reply('收到群聊消息!')
```
5.启动机器人
最后,使用下列代码启动机器人:
```python
bot.join()
```
完整代码示例:
```python
# 引入wxpy库
from wxpy import *
# 登录微信
bot = Bot()
# 定义回复函数
@bot.register()
def reply_msg(msg):
if isinstance(msg.chat, Friend) and not msg.sender == bot.self: # 如果是好友发送的消息并且不是自己发的
msg.reply('你好,我是机器人,收到你的消息了!')
elif isinstance(msg.chat, Group) and not msg.is_at: # 如果是群聊中的消息,但未@机器人
msg.reply('收到群聊消息!')
# 启动机器人
bot.join()
```
用python制作一个微信聊天机器人及源代码
好的,你想制作一个微信聊天机器人,需要以下步骤:
1. 注册一个微信公众号,并通过审核。
2. 在公众号设置中开启开发者模式,并获取AppID和AppSecret。
3. 在微信公众平台中申请开通“开发者模式”和“消息管理权限”。
4. 安装Flask框架和WeChat SDK。
5. 编写Python代码实现微信公众号的自动回复功能。
以下是一个简单的微信聊天机器人的代码示例:
```python
from flask import Flask, request
from wechatpy import parse_message
from wechatpy.replies import TextReply
app = Flask(__name__)
@app.route('/wechat', methods=['GET', 'POST'])
def wechat():
if request.method == 'GET':
token = 'your_token'
echostr = request.args.get('echostr', '')
if check_signature(token, request):
return echostr
else:
return 'Wrong validation token'
else:
xml = request.data
msg = parse_message(xml)
if msg.type == 'text':
reply = TextReply(content=auto_reply(msg.content), message=msg)
return reply.render()
else:
return ''
def check_signature(token, request):
signature = request.args.get('signature', '')
timestamp = request.args.get('timestamp', '')
nonce = request.args.get('nonce', '')
tmp_list = [token, timestamp, nonce]
tmp_list.sort()
tmp_str = ''.join(tmp_list)
tmp_str = hashlib.sha1(tmp_str.encode('utf-8')).hexdigest()
return tmp_str == signature
def auto_reply(msg):
# 在这里编写自动回复的逻辑
return '你好,我是机器人'
if __name__ == '__main__':
app.run(host='0.0.0.0', port=80)
```
这个代码示例使用了Flask框架和WeChat SDK,实现了微信公众号的自动回复功能。你需要将代码中的your_token替换为你在微信公众平台中设置的Token。
当有用户给公众号发送文本消息时,程序会调用auto_reply()函数进行自动回复。你可以在这个函数中编写自己的自动回复逻辑。
如果你想了解更多关于Python实现微信聊天机器人的知识,可以参考以下链接:
1. [使用Python实现微信公众号](https://zhuanlan.zhihu.com/p/146530965)
2. [WeChat SDK官方文档](https://wechatpy.readthedocs.io/en/stable/index.html)
阅读全文
相关推荐
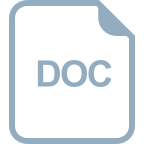
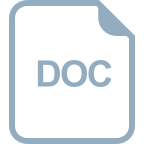
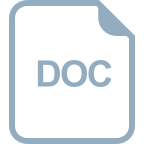



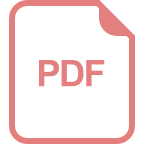
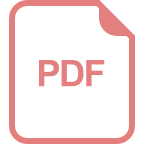
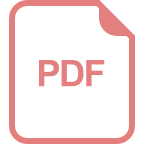
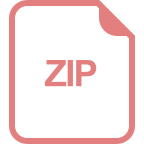





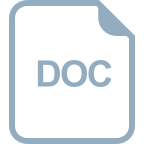
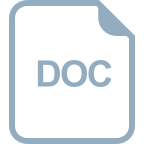