if URL(string: "https://www.google.com") != nil { import UIKit UIApplication.shared.open(url, options: [:]) { success in if success { print("The URL was delivered successfully.") } else { print("The URL failed to open.") } } } }报错Declaration is only valid at file scope
时间: 2023-04-03 10:04:16 浏览: 164
这个错误的意思是说,这个声明(代码块)只能在文件范围内有效。这通常是由于代码块被放在了一个错误的位置引起的,比如在函数内部或者其他语句块内部。
要解决这个问题,应该将代码块移到文件范围内,或者把它放到合适的函数内。如果你想在函数内部打开一个 URL,可以把这个代码块放到函数内部,并且确保函数已经被声明。
希望这能帮到你!
相关问题
openapp.jdmobile://virtual?params={"category":"jump","des":"m","url":"https://u.jd.com/nQo1Y5A","union_open":"union_cps"}
这个链接"openapp.jdmobile://virtual?params={"category":"jump","des":"m","url":"https://u.jd.com/nQo1Y5A","union_open":"union_cps"}"是用来在京东APP中打开指定商品的。
具体使用的代码如下:
```swift
static func goJD(_ url : String){
KeplerApiManager.sharedKPService()?.openKeplerPage(withURL: url, userInfo: nil, successCallback: {
debugPrint("open jd app success")
}, failedCallback: { code, msg in
debugPrint("open jd error code: ", code)
otherGoJD(url)
})
}
static func otherGoJD(_ url : String) {
let jdDict = ["category":"jump", "des":"getCoupon", "url":url ]
guard let jdStr = jdDict.formatJSON() else { return }
let urlStr = "openapp.jdmobile://virtual?params=\(jdStr)"
let utf8Str = urlStr.addingPercentEncoding(withAllowedCharacters: .urlQueryAllowed)
var openSuccess = false
if let jumpURL = URL(string: utf8Str ?? "") {
let isCanOpen = UIApplication.shared.canOpenURL(jumpURL)
if isCanOpen {
UIApplication.shared.open(jumpURL, options: [:], completionHandler: nil)
openSuccess = true
}
}
if (openSuccess == false) {
UIApplication.shared.open(URL(string: url)!, options: [:], completionHandler: nil)
}
}
```
通过调用goJD方法,传入京东商品的URL,即可在京东APP中打开该商品页面。如果无法打开京东APP,则会在浏览器中打开该商品链接。
对于京东的URL Scheme,可以在网上搜索到具体的参数传递方式。
func (c *cMatter) PutAdImage(r *ghttp.Request) { url := "https://api.oceanengine.com/open_api/2/file/image/ad/" token := "c1b4af1a34c3342ee5ad62e260f26ee330e4a30a" client := g.Client() client.SetHeader("Content-Type", "multipart/form-data") client.SetHeader("Accent-Token", token) uploadType := "UPLOAD_BY_FILE" imageSignature := gfile.Md5(r.GetUploadFile("image_file")) filename := r.Get("filename") response, err := client.PostMultipart(url, g.Map{ "advertiser_id": 1748895409387534, "upload_type": uploadType, "image_signature": imageSignature, "image_file": r.GetUploadFile("image_file"), "filename": filename, }) if err != nil { g.Log().Error(err) r.Response.WriteJson(g.Map{ "code": -1, "message": "Request failed", "data": nil, }) return } r.Response.Write(response.ReadAll()) }这段代码怎么重新优化,不使用Md5,PostMultipart,response.ReadAll()这些,请详细一点
好的,根据您的要求,下面是对代码进行优化的建议:
1. 不使用 `gfile.Md5` 方法:可以使用其他方法生成文件的唯一标识,例如使用文件的哈希值。您可以使用 `crypto/md5` 包来计算文件的 MD5 值。
```go
import (
"crypto/md5"
"encoding/hex"
"io"
"os"
)
// 计算文件的 MD5 值
func calculateMD5(filePath string) (string, error) {
file, err := os.Open(filePath)
if err != nil {
return "", err
}
defer file.Close()
hash := md5.New()
if _, err := io.Copy(hash, file); err != nil {
return "", err
}
md5Sum := hash.Sum(nil)
return hex.EncodeToString(md5Sum), nil
}
// 使用 calculateMD5 方法获取文件的唯一标识
imageSignature, err := calculateMD5(r.GetUploadFile("image_file"))
if err != nil {
// 处理错误
}
```
2. 不使用 `client.PostMultipart` 方法:可以使用 `client.Post` 方法发送带有文件的请求。首先,将文件读取为字节切片,然后将其作为请求体的一部分发送。
```go
import (
"bytes"
"io/ioutil"
)
// 读取上传的文件为字节切片
fileContent, err := ioutil.ReadAll(r.GetUploadFile("image_file"))
if err != nil {
// 处理错误
}
// 发送请求
response, err := client.Post(url, bytes.NewReader(fileContent), g.Map{
"Content-Type": "multipart/form-data",
"Accent-Token": token,
"advertiser_id": 1748895409387534,
"upload_type": uploadType,
"image_signature": imageSignature,
"filename": filename,
})
if err != nil {
// 处理错误
}
```
3. 不使用 `response.ReadAll` 方法:可以使用 `ioutil.ReadAll` 方法读取响应体内容。
```go
// 读取响应体内容
body, err := ioutil.ReadAll(response.Body)
if err != nil {
// 处理错误
}
// 将响应内容写入响应
r.Response.Write(body)
```
通过以上优化,您可以实现不使用 `gfile.Md5`、`client.PostMultipart` 和 `response.ReadAll` 方法的功能。请根据您的实际需求进行调整并处理错误。
阅读全文
相关推荐
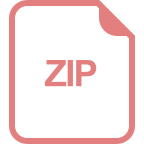
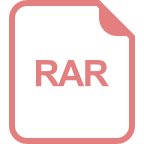
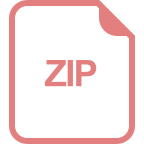

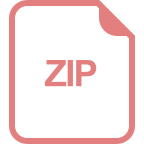




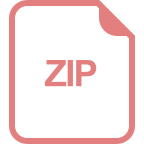
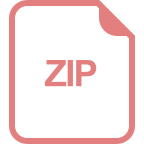
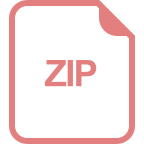
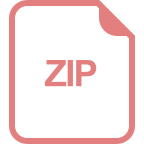
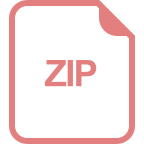