@objc func removeAllMeasurementReminder() { var removeIdentifiers = [String]() for i in 0...6 { for j in 0...9 { if #available(iOS 10.0, *) { removeIdentifiers.append(HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION + "\(i)" + "\(j)") } else { guard let allNotifications = UIApplication.shared.scheduledLocalNotifications else { return } for notification in allNotifications { let info = notification.userInfo as? [String: String] ?? ["":""] let identifier = info[HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION + "\(i)" + "\(j)"] if ((identifier?.hasPrefix(HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION)) != nil) { UIApplication.shared.cancelLocalNotification(notification) } } } } } if #available(iOS 10.0, *) { UNUserNotificationCenter.current().removePendingNotificationRequests(withIdentifiers: removeIdentifiers) } } 怎么放到子线程
时间: 2024-01-07 21:03:28 浏览: 25
可以使用GCD(Grand Central Dispatch)将代码块放到一个子线程中执行,代码如下:
```
DispatchQueue.global(qos: .background).async {
// 这里是需要在子线程中执行的代码
removeAllMeasurementReminder()
}
```
或者,您也可以使用OperationQueue来执行子线程中的代码块,代码如下:
```
let queue = OperationQueue()
queue.addOperation {
// 这里是需要在子线程中执行的代码
removeAllMeasurementReminder()
}
```
两种方法都可以将 `removeAllMeasurementReminder()` 方法放在一个子线程中执行。
相关问题
@objc func removeAllMeasurementReminder() { var removeIdentifiers = [String]() for i in 0...6 { for j in 0...9 { if #available(iOS 10.0, *) { removeIdentifiers.append(HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION + "\(i)" + "\(j)") } else { guard let allNotifications = UIApplication.shared.scheduledLocalNotifications else { return } for notification in allNotifications { let info = notification.userInfo as? [String: String] ?? ["":""] let identifier = info[HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION + "\(i)" + "\(j)"] if ((identifier?.hasPrefix(HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION)) != nil) { UIApplication.shared.cancelLocalNotification(notification) } } } } } if #available(iOS 10.0, *) { UNUserNotificationCenter.current().removePendingNotificationRequests(withIdentifiers: removeIdentifiers) } }函数内部操作移到子线程
可以将函数内部的操作移到一个异步的子线程中执行,避免在主线程中进行耗时操作导致界面卡顿。可以使用GCD的异步任务来实现:
```
@objc func removeAllMeasurementReminder() {
DispatchQueue.global(qos: .background).async {
var removeIdentifiers = [String]()
for i in 0...6 {
for j in 0...9 {
if #available(iOS 10.0, *) {
removeIdentifiers.append(HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION + "\(i)" + "\(j)")
} else {
guard let allNotifications = UIApplication.shared.scheduledLocalNotifications else {
return
}
for notification in allNotifications {
let info = notification.userInfo as? [String: String] ?? ["":""]
let identifier = info[HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION + "\(i)" + "\(j)"]
if ((identifier?.hasPrefix(HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION)) != nil) {
UIApplication.shared.cancelLocalNotification(notification)
}
}
}
}
}
if #available(iOS 10.0, *) {
UNUserNotificationCenter.current().removePendingNotificationRequests(withIdentifiers: removeIdentifiers)
}
}
}
```
此函数比较耗时,怎么优化一下 @objc func removeAllMeasurementReminder() { var removeIdentifiers = [String]() for i in 0...6 { for j in 0...9 { if #available(iOS 10.0, *) { removeIdentifiers.append(HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION + "\(i)" + "\(j)") } else { guard let allNotifications = UIApplication.shared.scheduledLocalNotifications else { return } for notification in allNotifications { let info = notification.userInfo as? [String: String] ?? ["":""] let identifier = info[HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION + "\(i)" + "\(j)"] if ((identifier?.hasPrefix(HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION)) != nil) { UIApplication.shared.cancelLocalNotification(notification) } } } } } if #available(iOS 10.0, *) { UNUserNotificationCenter.current().removePendingNotificationRequests(withIdentifiers: removeIdentifiers) } }
可以考虑对代码进行如下优化:
1. 将循环嵌套改为单层循环,用一个变量存储需要删除的 identifier,这样可以减少循环次数。
2. 对于 iOS 10 及以上版本,可以直接使用 `UNUserNotificationCenter.current().removeAllPendingNotificationRequests()` 方法来删除所有未触发的本地通知,这样不用遍历所有 identifier,可以减少时间复杂度。
3. 对于 iOS 9 及以下版本,可以将所有本地通知存储在一个数组中,然后遍历这个数组进行删除操作。
改进后的代码如下:
```
@objc func removeAllMeasurementReminder() {
var removeIdentifiers = [String]()
for i in 0..<7 {
for j in 0..<10 {
removeIdentifiers.append(HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION + "\(i)" + "\(j)")
}
}
if #available(iOS 10.0, *) {
UNUserNotificationCenter.current().removeAllPendingNotificationRequests()
} else {
guard let allNotifications = UIApplication.shared.scheduledLocalNotifications else {
return
}
for notification in allNotifications {
let info = notification.userInfo as? [String: String] ?? [:]
if let identifier = info[HW_BLOOD_PRESSURE_MEASUREMENT_NOTIFICATION], removeIdentifiers.contains(identifier) {
UIApplication.shared.cancelLocalNotification(notification)
}
}
}
}
```
这样,代码的执行效率应该会有所提高。
相关推荐
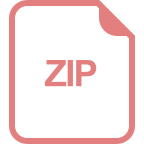
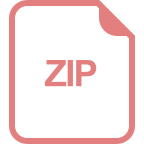
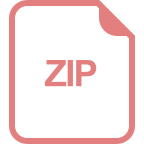







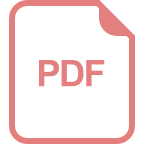
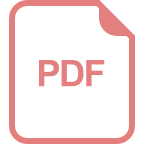
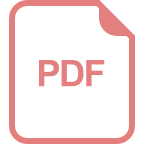
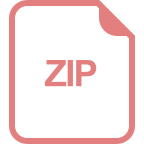