Reliability and Maintainability Simulation involves a large number of random variables. The use of dynamic arrays will greatly improve the efficiency of simulation and the scale of problem solving. Please design Vector. This problem requires the implementation of a vector class template, which can realize the storage and access of data. (1) [] operator can only access the existing elements. (2) The add method can automatically expand the internal storage space when accessing. Note that the behavior of this vector is different from that of std:: vector
时间: 2024-02-04 15:03:52 浏览: 21
Sure! Here's a possible implementation of a vector class template that meets your requirements:
```c++
template<typename T>
class Vector {
public:
Vector() : size_(0), capacity_(0), data_(nullptr) {}
Vector(const Vector& other) : size_(other.size_), capacity_(other.capacity_), data_(new T[capacity_]) {
for (size_t i = 0; i < size_; ++i) {
data_[i] = other.data_[i];
}
}
Vector& operator=(const Vector& other) {
if (this != &other) {
size_ = other.size_;
capacity_ = other.capacity_;
delete[] data_;
data_ = new T[capacity_];
for (size_t i = 0; i < size_; ++i) {
data_[i] = other.data_[i];
}
}
return *this;
}
~Vector() {
delete[] data_;
}
T& operator[](size_t i) {
if (i >= size_) {
throw std::out_of_range("Index out of range");
}
return data_[i];
}
const T& operator[](size_t i) const {
if (i >= size_) {
throw std::out_of_range("Index out of range");
}
return data_[i];
}
void add(const T& value) {
if (size_ == capacity_) {
if (capacity_ == 0) {
capacity_ = 1;
} else {
capacity_ *= 2;
}
T* new_data = new T[capacity_];
for (size_t i = 0; i < size_; ++i) {
new_data[i] = data_[i];
}
delete[] data_;
data_ = new_data;
}
data_[size_] = value;
++size_;
}
private:
size_t size_;
size_t capacity_;
T* data_;
};
```
Explanation:
- The `Vector` class template is parameterized by the type `T` of the elements it stores.
- The class has a default constructor that initializes the size, capacity, and data pointer to null, and a copy constructor that creates a new vector with the same size, capacity, and elements as another vector.
- The assignment operator is implemented using the copy-and-swap idiom to ensure strong exception safety.
- The destructor deallocates the memory used by the data pointer.
- The `operator[]` method is used to access elements by index. It checks if the index is within bounds and throws an `std::out_of_range` exception if not.
- The `add` method adds a new element to the end of the vector. If the vector is full, it doubles the capacity and allocates a new array with the new capacity, copies the existing elements, and replaces the old array with the new one.
相关推荐
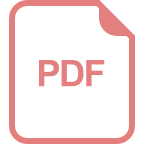
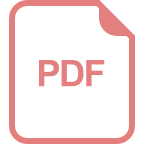
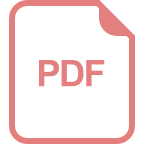















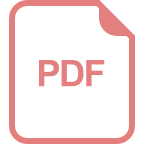