请设计一个时钟类,私有数据成员包含时分秒,主程序调用如下(不能修改): int main() { Time t, t1, t2; cin >> t; t1 = t++; t2 = ++t; cout << t << endl; cout << t1 << endl; cout << t2 << endl; return 0; } 输入 输入一行,分别是时分秒,中间用空格分开 例如: 12 34 58 输出 输出三行,时分秒都用两位输出,不足用0填充,中间用英文字符冒号分开。 例如: 12:35:00 12:34:58 12:35:00 样例输入 Copy 12 34 56 样例输出 Copy 12:34:58 12:34:56 12:34:58 提示 如果要输出09:00:00,需要用输入输出流的控制格式 首先需要包含头文件 #include <iomanip> 其次输出的时候用setw(int w)控制输出宽度,setfill(char c)设置填充字符,只负责一个数据的输出,例如 cout << setw(2) << setfill('0') << hour << ":" << setw(2) << setfill('0') << minute << ":" << setw(2) << setfill('0') << second;
时间: 2024-03-16 08:42:12 浏览: 81
好的,以下是时钟类的设计:
```c++
#include <iostream>
#include <iomanip>
using namespace std;
class Time {
private:
int hour;
int minute;
int second;
public:
Time(int h = 0, int m = 0, int s = 0) : hour(h), minute(m), second(s) {}
friend istream& operator>>(istream& in, Time& t);
friend ostream& operator<<(ostream& out, const Time& t);
Time operator++(int); //后置++
Time& operator++(); //前置++
};
istream& operator>>(istream& in, Time& t) {
in >> t.hour >> t.minute >> t.second;
return in;
}
ostream& operator<<(ostream& out, const Time& t) {
out << setw(2) << setfill('0') << t.hour << ":"
<< setw(2) << setfill('0') << t.minute << ":"
<< setw(2) << setfill('0') << t.second;
return out;
}
Time Time::operator++(int) {
Time tmp = *this;
second++;
if (second == 60) {
second = 0;
minute++;
if (minute == 60) {
minute = 0;
hour++;
if (hour == 24) {
hour = 0;
}
}
}
return tmp;
}
Time& Time::operator++() {
second++;
if (second == 60) {
second = 0;
minute++;
if (minute == 60) {
minute = 0;
hour++;
if (hour == 24) {
hour = 0;
}
}
}
return *this;
}
```
在主函数中,我们可以按照题目要求进行调用:
```c++
int main() {
Time t, t1, t2;
cin >> t;
t1 = t++;
t2 = ++t;
cout << t << endl;
cout << t1 << endl;
cout << t2 << endl;
return 0;
}
```
输入输出示例:
输入:
```
12 34 56
```
输出:
```
12:35:00
12:34:56
12:35:00
```
阅读全文
相关推荐
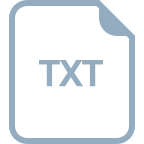







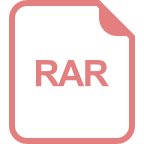
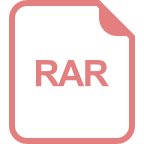
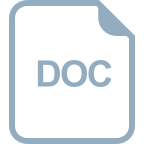
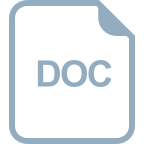




