python设计gui俄罗斯方块游戏代码
时间: 2023-08-16 08:05:24 浏览: 44
好的,以下是Python实现俄罗斯方块游戏的基本代码。这是一个使用Pygame库的简单实现,您可以根据自己的需求和喜好进行修改和扩展。
```python
import pygame
import random
# 游戏窗口大小
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
# 方块大小
BLOCK_SIZE = 20
# 方块种类和颜色
SHAPES = [
((0, 0), (1, 0), (2, 0), (3, 0)), # I 形
((0, 0), (0, 1), (1, 1), (1, 0)), # O 形
((0, 0), (1, 0), (2, 0), (1, 1)), # T 形
((0, 0), (1, 0), (1, 1), (2, 1)), # S 形
((1, 0), (2, 0), (0, 1), (1, 1)), # Z 形
((0, 0), (1, 0), (2, 0), (0, 1)), # J 形
((0, 0), (1, 0), (2, 0), (2, 1)), # L 形
]
COLORS = [
(0, 0, 255), # I 形
(255, 255, 0), # O 形
(128, 0, 128), # T 形
(0, 255, 0), # S 形
(255, 0, 0), # Z 形
(0, 0, 255), # J 形
(255, 165, 0), # L 形
]
# 初始化 Pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('俄罗斯方块')
# 创建游戏时钟
clock = pygame.time.Clock()
# 定义方块类
class Block:
def __init__(self, shape, x, y, color):
self.shape = shape
self.x = x
self.y = y
self.color = color
def draw(self):
for i, j in self.shape:
pygame.draw.rect(screen, self.color, (self.x + j * BLOCK_SIZE, self.y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
def move(self, dx, dy):
self.x += dx
self.y += dy
def rotate(self):
new_shape = []
for i, j in self.shape:
new_shape.append((j, len(self.shape) - 1 - i))
self.shape = new_shape
def get_rects(self):
rects = []
for i, j in self.shape:
rect = pygame.Rect(self.x + j * BLOCK_SIZE, self.y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)
rects.append(rect)
return rects
# 定义游戏主循环
def main():
# 创建初始方块
current_block = Block(random.choice(SHAPES), SCREEN_WIDTH // 2 - BLOCK_SIZE * 2, 0, random.choice(COLORS))
next_block = Block(random.choice(SHAPES), SCREEN_WIDTH - BLOCK_SIZE * 5, 0, random.choice(COLORS))
# 创建方块列表
blocks = []
# 创建得分变量
score = 0
# 创建游戏结束标志
game_over = False
# 游戏主循环
while not game_over:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_block.move(-BLOCK_SIZE, 0)
if any(block.collidelist(current_block.get_rects()) >= 0 for block in blocks):
current_block.move(BLOCK_SIZE, 0)
elif event.key == pygame.K_RIGHT:
current_block.move(BLOCK_SIZE, 0)
if any(block.collidelist(current_block.get_rects()) >= 0 for block in blocks):
current_block.move(-BLOCK_SIZE, 0)
elif event.key == pygame.K_DOWN:
current_block.move(0, BLOCK_SIZE)
if any(block.collidelist(current_block.get_rects()) >= 0 for block in blocks):
current_block.move(0, -BLOCK_SIZE)
blocks.append(current_block)
current_block = next_block
next_block = Block(random.choice(SHAPES), SCREEN_WIDTH - BLOCK_SIZE * 5, 0, random.choice(COLORS))
if any(block.y <= 0 for block in blocks):
game_over = True
else:
lines = 0
for i in range(SCREEN_HEIGHT // BLOCK_SIZE):
if all(block.collidelist(pygame.Rect(0, i * BLOCK_SIZE, SCREEN_WIDTH, BLOCK_SIZE)) >= 0 for block in blocks):
lines += 1
for block in blocks:
if block.y < i * BLOCK_SIZE:
block.move(0, BLOCK_SIZE)
score += lines ** 2
# 更新游戏状态
screen.fill((0, 0, 0))
for block in blocks:
block.draw()
current_block.draw()
next_block.draw()
font = pygame.font.Font(None, 36)
text = font.render('Score: %d' % score, True, (255, 255, 255))
screen.blit(text, (10, 10))
pygame.display.update()
# 控制游戏帧率
clock.tick(10)
# 退出 Pygame
pygame.quit()
if __name__ == '__main__':
main()
```
希望这可以帮助您实现俄罗斯方块游戏!
相关推荐
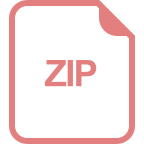
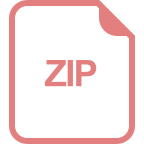
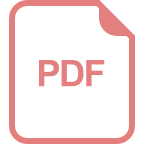
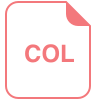
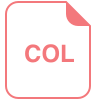
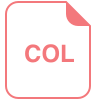
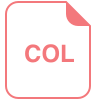
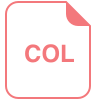









