Java中,现在有一组List<BoAttribute>类型的数据,其中,我需要取出BoAttribute实体的某个字段,叫:CharLen,改字段为数字类型,我需要求出所有CharLen的总数
时间: 2023-09-03 19:27:22 浏览: 55
### 回答1:
你好,根据你的问题描述,我可以给出以下Java代码实现:
```java
List<BoAttribute> list = new ArrayList<>(); // 假设数据已经初始化
int totalCharLen = 0; // 存储CharLen总数
for (BoAttribute boAttribute : list) {
totalCharLen += boAttribute.getCharLen();
}
System.out.println("CharLen总数为:" + totalCharLen);
```
以上代码中,假设数据已经初始化,我们遍历List中的每个BoAttribute实体,调用该实体的getCharLen()方法获取CharLen值,并累加到totalCharLen变量中。最后输出totalCharLen即可。
希望能够帮到你,如果有其他问题,随时提出哦~
### 回答2:
要求取出List<BoAttribute>中所有BoAttribute实体的CharLen字段的数字类型值,并求出这些值的总和。
首先,我们可以通过遍历List<BoAttribute>,逐个访问每个BoAttribute实体。可以使用for循环来实现:
```java
List<BoAttribute> boAttributes = ... ; // 初始化List<BoAttribute>数据
int totalCharLen = 0;
for (BoAttribute boAttribute : boAttributes) {
int charLen = boAttribute.getCharLen(); // 假设获取CharLen字段的方法为getCharLen()
totalCharLen += charLen;
}
System.out.println("CharLen总数为:" + totalCharLen);
```
在循环中,通过使用getCharLen()方法获取每个BoAttribute实体的CharLen字段的值,并累加到totalCharLen变量中。
注意:前提是BoAttribute实体类中必须存在getCharLen()方法,并将CharLen字段转换为数字类型。
此外,还可以使用Java 8的流(Stream)操作来实现求和:
```java
int totalCharLen = boAttributes.stream()
.mapToInt(BoAttribute::getCharLen) // 假设获取CharLen字段的方法为getCharLen()
.sum();
System.out.println("CharLen总数为:" + totalCharLen);
```
这种方式通过将List<BoAttribute>转换为流,然后使用mapToInt()方法将BoAttribute实体转换为CharLen字段的值,并使用sum()方法求和。
无论哪种方式,最终的结果都是求出所有BoAttribute实体的CharLen字段的数字类型值的总和。
### 回答3:
在Java中,我们可以使用循环遍历List<BoAttribute>,并通过调用BoAttribute实体的getter方法获取CharLen字段的值,将这些值累加得到所有CharLen的总数。
具体代码如下:
```java
List<BoAttribute> boAttributeList = // 初始化List<BoAttribute>数据
int totalCharLen = 0;
for (BoAttribute boAttribute : boAttributeList) {
totalCharLen += boAttribute.getCharLen();
}
System.out.println("所有CharLen的总数为:" + totalCharLen);
```
在上述代码中,假设我们已经初始化了List<BoAttribute>的数据。然后通过循环遍历,对于每一个BoAttribute实体,调用其getCharLen()方法获取CharLen字段的值,并累加到totalCharLen变量中。最后,打印出所有CharLen的总数。
需要注意的是,BoAttribute类中必须提供一个公有的getter方法来获取CharLen字段的值。
相关推荐
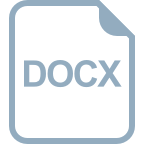
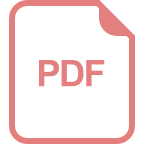
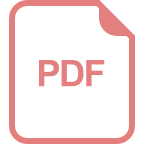




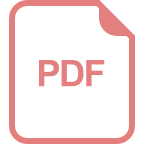
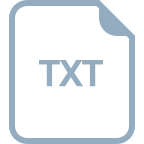
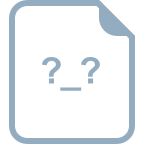
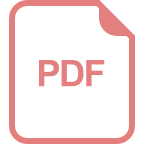
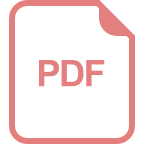
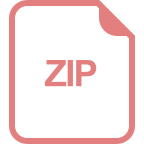
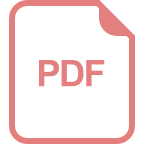
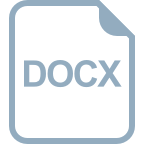
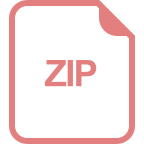