用Java写一段代码,要求定义一个Team类型的ArrayList集合 并往里面添加1234四个元素,并随机选取两个比较大小,确保比较6次
时间: 2024-02-05 10:11:40 浏览: 80
以下是Java代码:
```java
import java.util.ArrayList;
import java.util.Collections;
public class TeamComparison {
public static void main(String[] args) {
ArrayList<Team> teamList = new ArrayList<>();
teamList.add(new Team("Team 1", 10));
teamList.add(new Team("Team 2", 8));
teamList.add(new Team("Team 3", 12));
teamList.add(new Team("Team 4", 9));
int count = 0;
while (count < 6) {
int index1 = (int) (Math.random() * teamList.size());
int index2 = (int) (Math.random() * teamList.size());
if (index1 != index2) {
Team team1 = teamList.get(index1);
Team team2 = teamList.get(index2);
int result = team1.compareTo(team2);
System.out.println(team1.getName() + " vs " + team2.getName() + ": " + result);
count++;
}
}
}
static class Team implements Comparable<Team> {
private String name;
private int score;
public Team(String name, int score) {
this.name = name;
this.score = score;
}
public String getName() {
return name;
}
public int getScore() {
return score;
}
@Override
public int compareTo(Team o) {
return Integer.compare(score, o.score);
}
}
}
```
解释一下代码:
首先,我们定义了一个静态内部类 `Team`,它有两个属性,分别是队名和分数。`Team` 实现了 `Comparable` 接口,这样我们就可以使用 `Collections.sort()` 方法对 `ArrayList` 进行排序。
在 `main` 方法中,我们创建了一个 `ArrayList` 对象 `teamList`,向其中添加了四个 `Team` 对象。接着,我们使用一个循环来比较两支队伍的分数大小。在循环中,我们先生成两个随机数作为索引,然后检查这两个索引是否相等。如果相等,说明选中了同一支队伍,需要重新选取。否则,我们从 `teamList` 中取出两支队伍进行比较,得到比较结果后打印出来。
值得一提的是,我们在 `compareTo` 方法中使用了 `Integer.compare()` 方法,这样可以更简洁地实现比较操作。如果我们直接使用 `score - o.score`,会有可能出现整型溢出的情况。
阅读全文
相关推荐
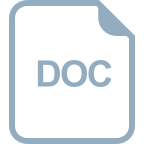
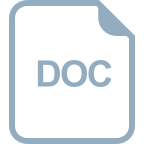
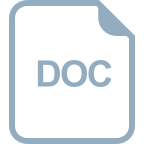
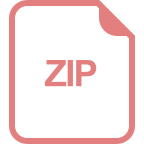
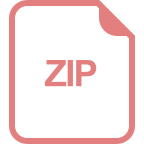
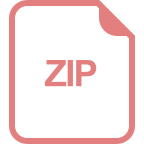
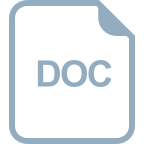
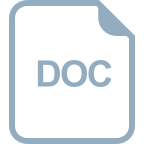
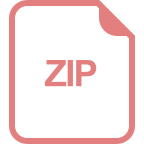
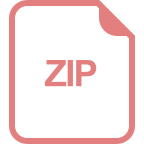
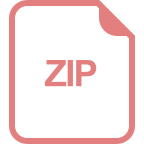
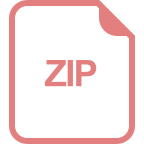
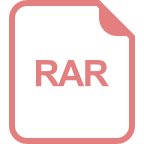
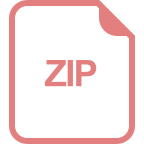
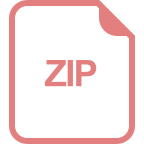
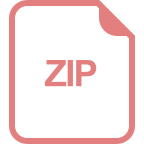
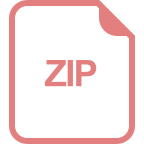