场景预设:创建一个手机通讯录,该通讯录具有以下功能: 1.添加联系人 2.查看通讯录 3.删除联系人 4.修改联系人信息 5.查找联系人 6.退出 要求编写程序实现上述功能
时间: 2024-11-10 09:14:27 浏览: 5
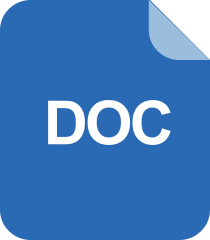
通讯录实现添加,删除,查找联系人,密码锁功能.doc
场景预设的手机通讯录程序可以采用经典的结构化设计思路,通常会包含以下几个步骤:
```python
class Contact:
def __init__(self, name, phone_number):
self.name = name
self.phone_number = phone_number
class AddressBook:
def __init__(self):
self.contacts = []
# 添加联系人
def add_contact(self, name, phone_number):
new_contact = Contact(name, phone_number)
self.contacts.append(new_contact)
# 查看通讯录
def view_contacts(self):
for contact in self.contacts:
print(f"Name: {contact.name}, Phone Number: {contact.phone_number}")
# 删除联系人
def delete_contact(self, name):
for index, contact in enumerate(self.contacts):
if contact.name == name:
del self.contacts[index]
break
# 修改联系人信息
def update_contact_info(self, old_name, new_phone_number):
for contact in self.contacts:
if contact.name == old_name:
contact.phone_number = new_phone_number
break
# 查找联系人
def find_contact(self, name):
found_contact = None
for contact in self.contacts:
if contact.name == name:
found_contact = contact
break
return found_contact if found_contact else "Contact not found"
# 退出
def exit(self):
print("Exiting the address book...")
# 示例使用
address_book = AddressBook()
address_book.add_contact("Alice", "1234567890")
address_book.view_contacts()
address_book.update_contact_info("Alice", "0987654321")
address_book.find_contact("Alice")
address_book.delete_contact("Alice")
address_book.exit()
```
这个简单的例子展示了如何通过Python实现一个基础的手机通讯录应用,包括基本操作的功能。实际应用可能会需要考虑更多的细节,比如数据持久化、错误处理等。
阅读全文
相关推荐
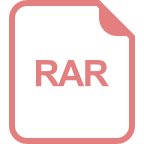
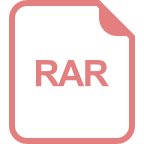



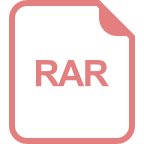
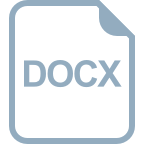
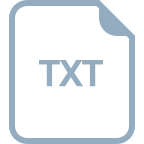
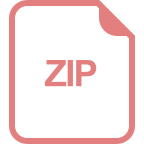
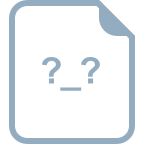
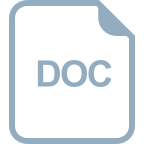
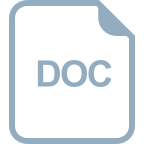
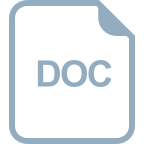
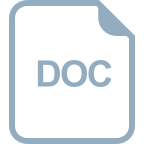
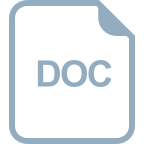
