dbscan算法聚类C++代码
时间: 2024-12-26 09:09:47 浏览: 2
DBSCAN(Density-Based Spatial Clustering of Applications with Noise,基于密度的空间聚类应用算法)是一种非监督学习的聚类算法,它不需要预先指定聚类的数量,而是根据数据点周围的密度来发现聚类。以下是使用C++实现DBSCAN的一个简化版伪代码:
```cpp
#include <vector>
#include <cmath>
// 定义数据结构Point,包含x,y坐标
struct Point {
double x, y;
};
// 计算两个点之间的欧氏距离
double distance(Point p1, Point p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2));
}
class DBSCAN {
public:
// 初始化函数
DBSCAN(double eps, int minPts, const std::vector<Point>& data);
// 主要聚类操作
void cluster();
private:
std::vector<Point> points; // 数据集
double eps; // 密度邻域半径
int minPts; // 最小邻居数
std::vector<std::vector<int>> clusters; // 存储每个簇的索引
bool isCorePoint(int idx); // 判断某个点是否为核心点
bool isNeighbor(int idx1, int idx2); // 判断两点是否临近
};
DBSCAN::DBSCAN(double eps, int minPts, const std::vector<Point>& data) {
// 初始化
this->eps = eps;
this->minPts = minPts;
this->points = data;
}
void DBSCAN::cluster() {
for (int i = 0; i < points.size(); ++i) {
if (!isCorePoint(i)) continue;
// 开始扩展簇
expandCluster(i);
}
}
bool DBSCAN::isCorePoint(int idx) {
int neighbors = 0;
for (int j = 0; j < points.size(); ++j) {
if (distance(points[idx], points[j]) <= eps && !visited[j]) {
++neighbors;
visited[j] = true;
}
}
return neighbors >= minPts;
}
bool DBSCAN::isNeighbor(int idx1, int idx2) {
return distance(points[idx1], points[idx2]) <= eps;
}
void DBSCAN::expandCluster(int seed) {
visited[seed] = true;
clusters.push_back(std::vector<int>({seed}));
std::queue<int> frontier;
frontier.push(seed);
while (!frontier.empty()) {
int current = frontier.front();
frontier.pop();
for (int neighbor_idx = 0; neighbor_idx < points.size(); ++neighbor_idx) {
if (!visited[neighbor_idx] && isNeighbor(current, neighbor_idx)) {
visited[neighbor_idx] = true;
frontier.push(neighbor_idx);
clusters.back().push_back(neighbor_idx);
}
}
}
}
// 使用示例
int main() {
std::vector<Point> data = ...; // 实际的数据集合
DBSCAN dbScan(eps, minPts, data);
dbScan.cluster();
// 输出聚类结果
for (const auto& cluster : dbScan.clusters) {
std::cout << "Cluster: ";
for (int point_id : cluster) {
std::cout << "[" << points[point_id].x << ", " << points[point_id].y << "] ";
}
std::cout << "\n";
}
return 0;
}
```
这只是一个简化的版本,实际应用中可能需要添加错误处理、优化等。注意,这个代码没有包括噪声点的处理,通常会在最后一步移除被认为是噪声的孤立点。
阅读全文
相关推荐
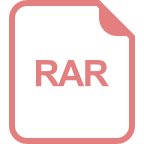
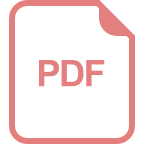
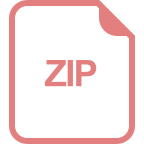


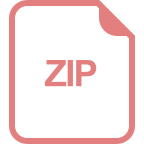
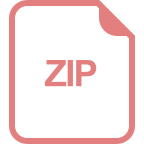
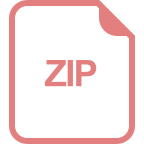
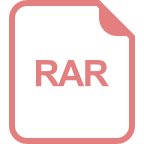
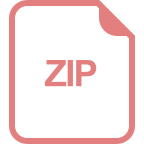
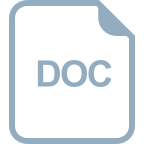
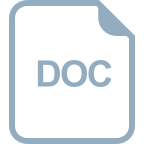




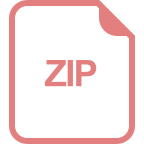
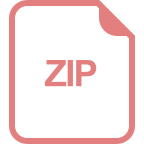