pcl dbscan聚类算法 c++
时间: 2023-06-29 09:14:06 浏览: 492
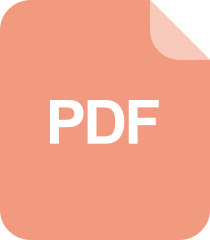
用C++实现DBSCAN聚类算法

PCL(点云库)中的DBSCAN聚类算法是一种基于密度的聚类算法,用于在点云数据中识别聚类。下面是一个简单的C++代码示例,用于在PCL中实现DBSCAN聚类算法:
```c++
#include <iostream>
#include <pcl/point_types.h>
#include <pcl/filters/voxel_grid.h>
#include <pcl/features/normal_3d.h>
#include <pcl/segmentation/extract_clusters.h>
#include <pcl/segmentation/sac_segmentation.h>
#include <pcl/sample_consensus/method_types.h>
#include <pcl/sample_consensus/model_types.h>
#include <pcl/ModelCoefficients.h>
#include <pcl/kdtree/kdtree.h>
#include <pcl/segmentation/extract_clusters.h>
#include <pcl/filters/passthrough.h>
#include <pcl/visualization/cloud_viewer.h>
int main (int argc, char** argv)
{
//创建点云数据对象
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_filtered(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_normals(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_filtered_normals(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZRGB>::Ptr colored_cloud(new pcl::PointCloud<pcl::PointXYZRGB>);
//读入点云数据
pcl::io::loadPCDFile<pcl::PointXYZ>("input_cloud.pcd", *cloud);
//对点云进行下采样
pcl::VoxelGrid<pcl::PointXYZ> sor;
sor.setInputCloud(cloud);
sor.setLeafSize(0.01f, 0.01f, 0.01f);
sor.filter(*cloud_filtered);
//计算点云法线
pcl::NormalEstimation<pcl::PointXYZ, pcl::Normal> ne;
ne.setInputCloud(cloud_filtered);
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>());
ne.setSearchMethod(tree);
pcl::PointCloud<pcl::Normal>::Ptr cloud_normals_ptr(new pcl::PointCloud<pcl::Normal>);
ne.setRadiusSearch(0.03);
ne.compute(*cloud_normals_ptr);
cloud_normals = boost::make_shared<pcl::PointCloud<pcl::PointXYZ> >();
copyPointCloud(*cloud_normals_ptr, *cloud_normals);
//对法线进行下采样
sor.setInputCloud(cloud_normals);
sor.setLeafSize(0.01f, 0.01f, 0.01f);
sor.filter(*cloud_filtered_normals);
//创建DBSCAN聚类算法对象
pcl::EuclideanClusterExtraction<pcl::PointXYZ> ec;
ec.setClusterTolerance(0.02);
ec.setMinClusterSize(100);
ec.setMaxClusterSize(25000);
ec.setInputCloud(cloud_filtered_normals);
std::vector<pcl::PointIndices> cluster_indices;
ec.extract(cluster_indices);
//对聚类结果进行可视化
int j = 0;
for (std::vector<pcl::PointIndices>::const_iterator it = cluster_indices.begin(); it != cluster_indices.end(); ++it)
{
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_cluster(new pcl::PointCloud<pcl::PointXYZ>);
for (std::vector<int>::const_iterator pit = it->indices.begin(); pit != it->indices.end(); ++pit)
cloud_cluster->points.push_back(cloud_filtered_normals->points[*pit]);
cloud_cluster->width = cloud_cluster->points.size();
cloud_cluster->height = 1;
cloud_cluster->is_dense = true;
//为每个聚类赋予不同的颜色
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointXYZ> color_handler(cloud_cluster, 255 - j * 50, 0, j * 50);
j++;
//将聚类结果可视化
pcl::visualization::PCLVisualizer viewer("Cluster viewer");
viewer.setBackgroundColor(1, 1, 1);
viewer.addPointCloud<pcl::PointXYZ>(cloud_cluster, color_handler, "cluster");
viewer.setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 3, "cluster");
viewer.spin();
}
return (0);
}
```
在上面的代码中,我们首先读入点云数据,然后对点云进行下采样,计算点云法线,对法线进行下采样,然后创建DBSCAN聚类算法对象,并设置聚类参数。最后,我们对聚类结果进行可视化,为每个聚类赋予不同的颜色。
需要注意的是,由于DBSCAN聚类算法是基于密度的聚类算法,它对点云数据中的点密度变化比较敏感,因此需要根据实际情况调整聚类参数。
阅读全文
相关推荐
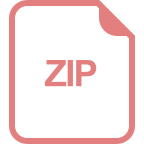

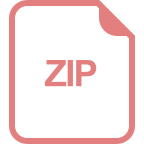



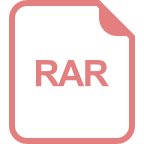
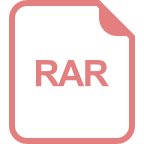
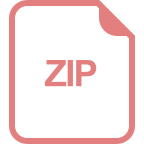
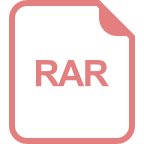
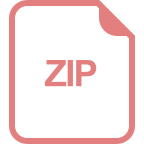
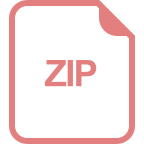
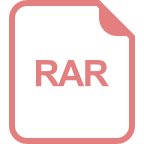
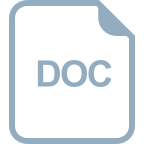
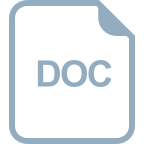
