pcl点云聚类反光柱c++
时间: 2024-10-15 21:04:56 浏览: 14
PCL(Point Cloud Library),是一个开源的用于处理3D点云数据的库,主要用于计算机视觉和机器人技术领域。如果你提到的“反光柱”可能是特定类型的点云特征,比如在环境中识别出类似支柱、杆状物体等。
对于PCL中点云聚类反光柱的C++实现,通常涉及以下几个步骤:
1. **数据预处理**:首先,你需要读取包含反光柱点云的数据,可能会通过如激光雷达扫描、RGB-D相机或其他传感器获取。使用PCL提供的I/O函数(如`pcl::io::loadCloud()`)加载数据。
2. **滤波和分割**:去除噪声和非目标点,可以使用诸如`pcl::PassThroughFilter` 或 `pcl::RANSAC Plane Segmentation` 等滤波器。对于反光柱,可能需要基于高度或者垂直方向进行筛选。
3. **特征提取**:针对点云特征,例如形状或纹理,选择适合的特征描述符,如PCA、FPFH或者SHOT。
4. **聚类算法**:应用聚类算法对相似的反光柱进行分组。PCL提供了多种聚类选项,如K-means (`pcl::KMeansClustering`)、DBSCAN (`pcl::DBSCAN`) 或层次聚类(`pcl::AgglomerativeClustering`)。
5. **结果分析**:最后,根据聚类结果对反光柱进行进一步的分析,如测量尺寸、定位等。
相关问题
pcl 点云聚类算法
点云聚类算法(Point Cloud clustering algorithm,PCL)是一种用于处理三维点云数据的算法,主要用于将一组离散的点云对象聚类到不同的群集中。
该算法的主要思想是通过计算点云之间的距离和相似性来找出彼此聚集在一起的点。它可以被用来识别同一物体的不同部分或者相似形状的物体。点云聚类算法通常包含以下几个步骤:
1. 数据预处理:首先对原始点云数据进行预处理,包括去除杂散噪声、滤波处理等,以提高后续聚类的准确性。
2. 特征提取:根据点的属性,如位置、颜色、法向量等提取点云的特征。这些特征将用于计算点与点之间的相似性。
3. 距离计算:根据提取的特征计算点与点之间的距离。通常使用的距离计算方法包括欧氏距离、马哈拉诺比斯距离等。
4. 聚类运算:根据计算的距离和相似性,将点云对象分配到不同的聚类中。常用的聚类算法有基于密度的DBSCAN算法、基于连通性的分割算法等。
5. 聚类结果分析:对聚类结果进行分析和验证。可以根据聚类结果确定不同群集的属性、样式和几何特征。
点云聚类算法在多个领域中有广泛应用,如三维物体识别、环境分析、医学图像处理等。它可以帮助我们有效处理大量的三维点云数据,并从中提取出有用的信息。
dbscan实现pcd文件中的点云聚类,使用c++、pcl完成
DBSCAN是一种基于密度的聚类算法,可以用于点云数据的聚类。PCL(Point Cloud Library)是一个常用的点云处理库,提供了很多点云处理算法的实现,包括DBSCAN。下面是使用PCL实现DBSCAN点云聚类的C++代码:
```c++
#include <iostream>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
#include <pcl/segmentation/extract_clusters.h>
#include <pcl/filters/voxel_grid.h>
#include <pcl/filters/passthrough.h>
#include <pcl/visualization/cloud_viewer.h>
using namespace std;
int main(int argc, char** argv) {
if (argc < 2) {
cout << "Usage: " << argv[0] << " <pcd_file>" << endl;
return -1;
}
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
if (pcl::io::loadPCDFile<pcl::PointXYZ>(argv[1], *cloud) == -1) {
cout << "Failed to load PCD file" << endl;
return -1;
}
// 下采样
pcl::VoxelGrid<pcl::PointXYZ> vg;
vg.setInputCloud(cloud);
vg.setLeafSize(0.01f, 0.01f, 0.01f);
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_filtered(new pcl::PointCloud<pcl::PointXYZ>);
vg.filter(*cloud_filtered);
// 过滤
pcl::PassThrough<pcl::PointXYZ> pass;
pass.setInputCloud(cloud_filtered);
pass.setFilterFieldName("z");
pass.setFilterLimits(0.0, 1.0);
pass.filter(*cloud_filtered);
// DBSCAN聚类
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>);
tree->setInputCloud(cloud_filtered);
std::vector<pcl::PointIndices> cluster_indices;
pcl::EuclideanClusterExtraction<pcl::PointXYZ> ec;
ec.setClusterTolerance(0.02);
ec.setMinClusterSize(50);
ec.setMaxClusterSize(1000);
ec.setSearchMethod(tree);
ec.setInputCloud(cloud_filtered);
ec.extract(cluster_indices);
// 可视化
pcl::visualization::PCLVisualizer viewer("DBSCAN Clustering");
int color_idx = 0;
for (auto it = cluster_indices.begin(); it != cluster_indices.end(); ++it) {
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_cluster(new pcl::PointCloud<pcl::PointXYZ>);
for (auto pit = it->indices.begin(); pit != it->indices.end(); ++pit) {
cloud_cluster->points.push_back(cloud_filtered->points[*pit]);
}
cloud_cluster->width = cloud_cluster->points.size();
cloud_cluster->height = 1;
cloud_cluster->is_dense = true;
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointXYZ> color_handler(cloud_cluster, color_idx % 255, (color_idx+128) % 255, (color_idx+192) % 255);
viewer.addPointCloud<pcl::PointXYZ>(cloud_cluster, color_handler, "cluster_" + to_string(color_idx));
viewer.setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 3, "cluster_" + to_string(color_idx));
color_idx++;
}
viewer.addPointCloud<pcl::PointXYZ>(cloud_filtered, "input_cloud");
viewer.setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 1, "input_cloud");
viewer.spin();
return 0;
}
```
这里的输入是一个PCD文件,程序首先使用PCL的loadPCDFile函数加载点云数据。然后对点云数据进行下采样和过滤,以减少噪声和加速聚类的速度。下采样使用VoxelGrid滤波器,过滤使用PassThrough滤波器。接着,使用DBSCAN算法进行聚类,其中设置了聚类的参数,如聚类半径、最小点数等。最后将聚类结果可视化,不同的聚类使用不同的颜色显示。
需要注意的是,需要在编译时链接PCL库,可以使用以下命令进行编译:
```
g++ dbscan_pcl.cpp -o dbscan_pcl -I/usr/include/pcl-1.8 -lpcl_common -lpcl_io -lpcl_segmentation -lpcl_filters -lpcl_search -lpcl_visualization
```
其中-I选项指定PCL库的头文件路径,-l选项指定需要链接的库。
阅读全文
相关推荐
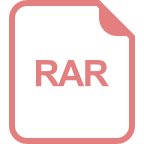
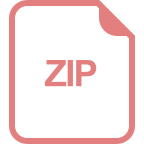
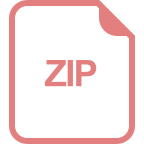
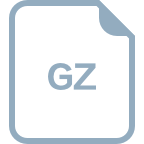
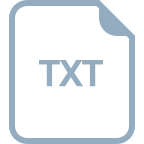
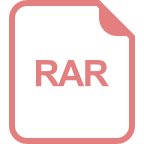
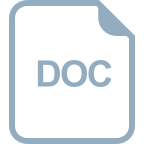
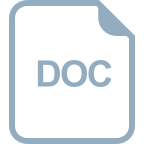
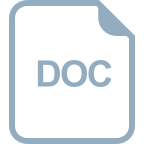








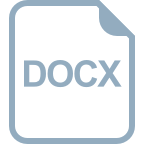