std::map<int,std::string>g_mapDeviceIndex;的使用方法,使用已知的string字符串得到对应的int值。
时间: 2024-09-10 07:02:55 浏览: 72
`std::map` 是 C++ 标准库中的一个关联容器,它存储的元素是键值对,其中每个键都是唯一的,并且与一个值相关联。在给定的例子中,`g_mapDeviceIndex` 是一个从 `int` 到 `std::string` 的映射。这意味着你可以使用一个整数(`int`)作为键来获取对应的字符串(`std::string`)。
为了使用 `g_mapDeviceIndex` 来获取一个已知的字符串值对应的整数,你需要首先确保这个字符串确实存在于映射中。如果存在,你可以通过以下方法来获取对应的整数值:
```cpp
#include <iostream>
#include <string>
#include <map>
int main() {
std::map<int, std::string> g_mapDeviceIndex;
// 假设映射已经被填充了键值对
g_mapDeviceIndex[1] = "设备1";
g_mapDeviceIndex[2] = "设备2";
// ... 其他键值对
// 已知的字符串值
std::string deviceName = "设备2";
// 使用 find 方法查找字符串值对应的键
auto it = g_mapDeviceIndex.begin();
while (it != g_mapDeviceIndex.end()) {
if (it->second == deviceName) {
std::cout << "找到设备,对应的键值为: " << it->first << std::endl;
break;
}
++it;
}
// 如果没有找到,可以输出未找到的信息
if (it == g_mapDeviceIndex.end()) {
std::cout << "设备名未找到对应的键值。" << std::endl;
}
return 0;
}
```
在这个例子中,我们通过遍历 `std::map` 的所有元素来查找与给定字符串相匹配的键值对。如果找到了匹配的字符串,我们就打印出对应的键值(`int`)。如果没有找到,我们打印出一个未找到的信息。
请注意,这个方法不是最高效的查找方法,因为它的查找时间复杂度为 O(n)。如果需要频繁查找并且对性能有要求,可以考虑使用 `std::map` 的 `find` 方法,它可以提供 O(log n) 的查找效率。下面是一个使用 `find` 方法的例子:
```cpp
// 使用 find 方法查找字符串值对应的键
auto it = g_mapDeviceIndex.find(deviceName);
if (it != g_mapDeviceIndex.end()) {
std::cout << "找到设备,对应的键值为: " << it->first << std::endl;
} else {
std::cout << "设备名未找到对应的键值。" << std::endl;
}
```
阅读全文
相关推荐
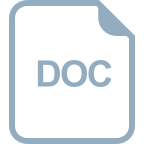
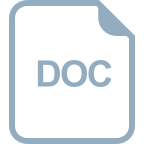
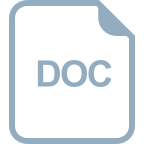
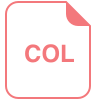
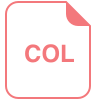
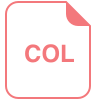
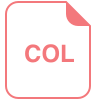
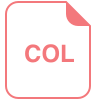
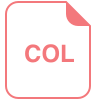
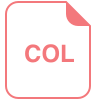
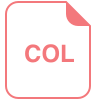
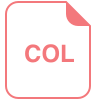
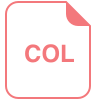
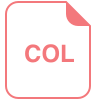




