python bs4中解析html
时间: 2023-10-23 09:31:48 浏览: 178
在Python中使用BeautifulSoup库(通常简称为bs4)解析HTML,可以按照以下步骤进行操作:
1. 安装BeautifulSoup库:使用pip命令安装bs4库,可以在命令行中执行以下命令:
```
pip install bs4
```
2. 导入BeautifulSoup库:在Python脚本的开头添加以下代码:
```python
from bs4 import BeautifulSoup
```
3. 读取HTML文件或字符串:有两种方式可以读取HTML内容,你可以选择其中之一:
- 从本地文件读取HTML内容:
```python
with open('example.html', 'r') as f:
html_content = f.read()
```
- 直接使用HTML字符串:
```python
html_content = """
<html>
<head>
<title>Example</title>
</head>
<body>
<h1>Hello, world!</h1>
</body>
</html>
"""
```
4. 创建BeautifulSoup对象:使用BeautifulSoup类将HTML内容解析为BeautifulSoup对象:
```python
soup = BeautifulSoup(html_content, 'html.parser')
```
5. 使用BeautifulSoup对象进行解析:通过BeautifulSoup对象可以获取HTML中的各种元素、标签、属性等信息。以下是几个常用的操作示例:
- 查找元素:
```python
# 根据标签名查找第一个匹配的元素
element = soup.find('div')
# 根据标签名查找所有匹配的元素
elements = soup.find_all('a')
# 根据CSS选择器查找元素
elements = soup.select('.class-name')
```
- 获取元素内容:
```python
# 获取元素的文本内容
text = element.text
# 获取元素的属性值
attr_value = element['attribute']
```
- 遍历元素:
```python
# 遍历所有匹配的元素
for element in elements:
print(element.text)
```
这些是使用BeautifulSoup库解析HTML的基本步骤和操作示例。你可以根据具体的需求进行更多的操作,可以参考BeautifulSoup官方文档获取更多用法和示例。
阅读全文
相关推荐
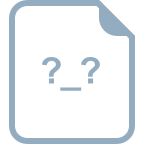
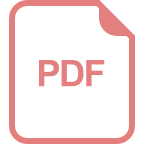
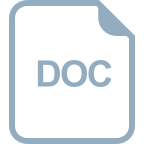
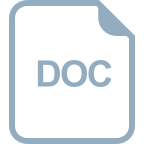
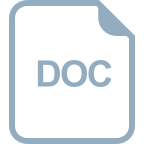
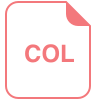









