对抗训练pytorch
时间: 2023-07-08 20:02:26 浏览: 102
对抗训练(Adversarial Training)是一种在机器学习领域中使用的一种技术方法,目的是提高模型在面对对抗性样本时的鲁棒性和泛化能力。在pytorch中,我们可以使用一些技术来进行对抗训练。
首先,对抗训练中最常用的方法是生成对抗网络(GAN)。GAN包括一个生成器网络和一个判别器网络。生成器网络用于生成对抗性样本,而判别器网络则根据输入判断样本是真实样本还是生成器产生的样本。通过对生成器和判别器进行交替训练,生成器可以不断优化产生更具对抗性的样本,而判别器也可以提高对抗样本的识别能力。
其次,对抗训练中还可以使用对抗样本生成方法,如生成对抗网络(GAN)的变种,或者使用优化算法,如快速梯度符号方法(FGSM)。这些方法可以通过对原始样本进行微小的扰动来生成对抗样本,从而欺骗模型并提高其鲁棒性。
另外,对抗训练中还可以使用预训练的模型进行迁移学习。预训练的模型可以提供更好的初始权重,并且具有更强的泛化能力。通过在预训练的模型上进行对抗训练可以让模型更好地适应对抗性样本。
总之,对抗训练是一种提高模型泛化能力和鲁棒性的重要技术。在pytorch中,我们可以使用生成对抗网络、对抗样本生成方法和迁移学习等技术来进行对抗训练。通过对模型进行对抗训练,我们可以提高模型在面对对抗性样本时的表现,从而使模型更具实用价值。
相关问题
BERT对抗训练pytorch代码
以下是一个BERT对抗训练的pytorch代码示例:
```
import torch
from transformers import BertTokenizer, BertForSequenceClassification
from transformers import AdamW, get_linear_schedule_with_warmup
from torch.utils.data import DataLoader, RandomSampler, SequentialSampler, TensorDataset
from tqdm import tqdm
import random
# 设置GPU
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# 设置随机种子
random.seed(2022)
np.random.seed(2022)
torch.manual_seed(2022)
torch.cuda.manual_seed_all(2022)
# 加载预训练好的BERT模型和tokenizer
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased', do_lower_case=True)
# 加载数据集
train_texts = ['This is a positive sentence.', 'This is a negative sentence.']
train_labels = [1, 0]
test_texts = ['This is another positive sentence.', 'This is another negative sentence.']
test_labels = [1, 0]
# 将数据集转换为BERT输入格式
train_encodings = tokenizer(train_texts, truncation=True, padding=True)
test_encodings = tokenizer(test_texts, truncation=True, padding=True)
train_labels = torch.tensor(train_labels)
test_labels = torch.tensor(test_labels)
train_dataset = TensorDataset(train_encodings['input_ids'], train_encodings['attention_mask'], train_labels)
test_dataset = TensorDataset(test_encodings['input_ids'], test_encodings['attention_mask'], test_labels)
# 设置训练参数
epochs = 3
batch_size = 8
learning_rate = 2e-5
epsilon = 1e-8
num_adv_steps = 1
adv_learning_rate = 1e-5
# 定义对抗函数,使用FGM对抗训练
def fgsm_attack(input_ids, attention_mask, labels, epsilon):
# 将模型设置为训练模式
model.train()
# 创建对抗样本
input_ids.requires_grad = True
attention_mask.requires_grad = True
loss_func = torch.nn.CrossEntropyLoss()
outputs = model(input_ids, attention_mask=attention_mask, labels=labels)
loss = loss_func(outputs.logits, labels)
loss.backward()
# 对抗样本
input_ids_grad = torch.sign(input_ids.grad)
attention_mask_grad = torch.sign(attention_mask.grad)
input_ids = input_ids + epsilon * input_ids_grad
attention_mask = attention_mask + epsilon * attention_mask_grad
# 清除梯度
model.zero_grad()
input_ids.grad = None
attention_mask.grad = None
return input_ids, attention_mask
# 定义优化器和学习率调度器
optimizer = AdamW(model.parameters(), lr=learning_rate, eps=epsilon)
total_steps = len(train_dataset) * epochs
scheduler = get_linear_schedule_with_warmup(optimizer, num_warmup_steps=0, num_training_steps=total_steps)
# 训练模型
train_dataloader = DataLoader(train_dataset, sampler=RandomSampler(train_dataset), batch_size=batch_size)
for epoch in range(epochs):
model.train()
total_loss = 0
for batch in tqdm(train_dataloader, desc="Training"):
input_ids = batch[0].to(device)
attention_mask = batch[1].to(device)
labels = batch[2].to(device)
# 对抗训练
for i in range(num_adv_steps):
adv_input_ids, adv_attention_mask = fgsm_attack(input_ids, attention_mask, labels, epsilon)
adv_outputs = model(adv_input_ids, attention_mask=adv_attention_mask, labels=labels)
adv_loss = adv_outputs.loss
adv_loss.backward()
# 更新模型参数
optimizer.step()
scheduler.step()
model.zero_grad()
# 计算损失函数值
outputs = model(input_ids, attention_mask=attention_mask, labels=labels)
loss = outputs.loss
total_loss += loss.item()
avg_loss = total_loss / len(train_dataloader)
print("Epoch:", epoch+1, "Train loss:", avg_loss)
# 在测试集上测试模型
test_dataloader = DataLoader(test_dataset, sampler=SequentialSampler(test_dataset), batch_size=batch_size)
model.eval()
total_accuracy = 0
for batch in tqdm(test_dataloader, desc="Testing"):
input_ids = batch[0].to(device)
attention_mask = batch[1].to(device)
labels = batch[2].to(device)
with torch.no_grad():
outputs = model(input_ids, attention_mask=attention_mask)
logits = outputs.logits
preds = torch.argmax(logits, axis=1)
accuracy = torch.sum(preds == labels).item() / len(labels)
total_accuracy += accuracy
avg_accuracy = total_accuracy / len(test_dataloader)
print("Test accuracy:", avg_accuracy)
```
此代码使用FGM对抗训练方法,在每个训练步骤中对输入数据进行一次对抗样本生成,并使用对抗样本进行模型训练。在测试阶段,模型将在未经过对抗样本生成的测试数据上进行测试。
对抗学习pytorch
对抗学习(Adversarial Learning)是一种机器学习的方法,旨在通过训练一个生成模型和一个判别模型来使它们相互对抗并提高性能。在PyTorch中,可以使用以下步骤进行对抗学习:
1. 首先,定义生成模型(Generator)和判别模型(Discriminator)。生成模型用于生成合成样本,而判别模型用于区分真实样本和合成样本。
2. 实现生成模型和判别模型的网络结构。在PyTorch中,可以使用`torch.nn.Module`类创建自定义的神经网络模型,并定义各层的结构和参数。
3. 定义损失函数。对于对抗学习,通常使用对抗损失函数,如生成模型希望最小化判别模型的判断错误,而判别模型希望最小化真实样本和合成样本的区分度。常见的对抗损失函数包括GAN(生成对抗网络)中的生成器损失和判别器损失。
4. 定义优化器。在PyTorch中,可以使用`torch.optim`模块中的优化器(如Adam、SGD等)来更新模型的参数。
5. 在训练过程中,交替地训练生成模型和判别模型。首先,通过生成模型生成一批合成样本,然后使用判别模型对真实样本和合成样本进行判断,并计算损失函数。接着,根据损失函数的梯度,使用优化器更新生成模型和判别模型的参数。
6. 重复以上步骤,直到生成模型和判别模型收敛或达到预定的训练轮数。
需要注意的是,对抗学习是一个复杂的任务,可能需要进行调试和调优。可以根据具体问题的需求和数据集的特点,对生成模型和判别模型的网络结构、损失函数和优化器进行适当的调整和优化。
阅读全文
相关推荐
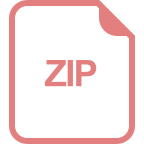
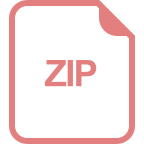
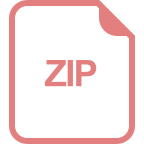
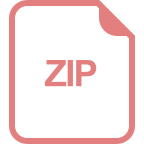








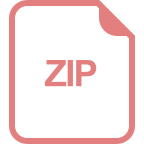