如何代码实现教职员工管理系统
时间: 2023-12-30 09:02:58 浏览: 28
教职员工管理系统可以采用面向对象编程思想,使用 Python 编程语言来实现。以下是一个简单的示例代码:
```python
class Person:
def __init__(self, name, age, gender):
self.name = name
self.age = age
self.gender = gender
class Employee(Person):
def __init__(self, name, age, gender, id, department, salary):
super().__init__(name, age, gender)
self.id = id
self.department = department
self.salary = salary
class Teacher(Employee):
def __init__(self, name, age, gender, id, department, salary, subjects):
super().__init__(name, age, gender, id, department, salary)
self.subjects = subjects
class Staff(Employee):
def __init__(self, name, age, gender, id, department, salary, duties):
super().__init__(name, age, gender, id, department, salary)
self.duties = duties
class ManagementSystem:
def __init__(self):
self.employees = []
def add_employee(self, employee):
self.employees.append(employee)
def remove_employee(self, employee):
self.employees.remove(employee)
def search_employee(self, id):
for employee in self.employees:
if employee.id == id:
return employee
return None
def list_employees(self):
for employee in self.employees:
print(f"{employee.name} ({employee.gender}), {employee.age} years old, ID: {employee.id}, Department: {employee.department}, Salary: {employee.salary}")
# 示例使用
ms = ManagementSystem()
# 添加员工
t1 = Teacher("Tom", 35, "Male", "T001", "Math", 5000, ["Algebra", "Geometry"])
t2 = Teacher("Mary", 30, "Female", "T002", "English", 4500, ["Literature", "Writing"])
s1 = Staff("John", 25, "Male", "S001", "Security", 3000, "Guarding")
s2 = Staff("Lisa", 28, "Female", "S002", "Cafeteria", 3500, "Cooking")
ms.add_employee(t1)
ms.add_employee(t2)
ms.add_employee(s1)
ms.add_employee(s2)
# 查询员工
e = ms.search_employee("T002")
if e:
print(f"Employee found: {e.name}")
else:
print("Employee not found")
# 列举员工
ms.list_employees()
# 删除员工
ms.remove_employee(s2)
# 列举员工
ms.list_employees()
```
这个示例代码中,通过定义 `Person`、`Employee`、`Teacher` 和 `Staff` 等类来表示不同类型的人员,通过 `ManagementSystem` 类来管理员工信息,包括添加、删除、查询和列举等功能。在实际使用时,还可以按照需要添加更多的功能或细节。
相关推荐
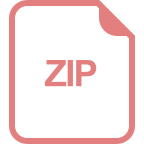
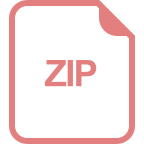
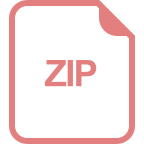















