如何用PHP代码实现教职员工管理系统
时间: 2024-02-19 10:03:24 浏览: 29
教职员工管理系统可以用PHP编写,以下是一个基本实现示例:
```php
class Person {
public $name;
public $age;
public $gender;
public function __construct($name, $age, $gender) {
$this->name = $name;
$this->age = $age;
$this->gender = $gender;
}
}
class Employee extends Person {
public $id;
public $department;
public $salary;
public function __construct($name, $age, $gender, $id, $department, $salary) {
parent::__construct($name, $age, $gender);
$this->id = $id;
$this->department = $department;
$this->salary = $salary;
}
}
class Teacher extends Employee {
public $subjects;
public function __construct($name, $age, $gender, $id, $department, $salary, $subjects) {
parent::__construct($name, $age, $gender, $id, $department, $salary);
$this->subjects = $subjects;
}
}
class Staff extends Employee {
public $duties;
public function __construct($name, $age, $gender, $id, $department, $salary, $duties) {
parent::__construct($name, $age, $gender, $id, $department, $salary);
$this->duties = $duties;
}
}
class ManagementSystem {
public $employees = [];
public function add_employee($employee) {
$this->employees[] = $employee;
}
public function remove_employee($employee) {
$key = array_search($employee, $this->employees);
if ($key !== false) {
unset($this->employees[$key]);
}
}
public function search_employee($id) {
foreach ($this->employees as $employee) {
if ($employee->id == $id) {
return $employee;
}
}
return null;
}
public function list_employees() {
foreach ($this->employees as $employee) {
echo "{$employee->name} ({$employee->gender}), {$employee->age} years old, ID: {$employee->id}, Department: {$employee->department}, Salary: {$employee->salary}<br>";
}
}
}
// 示例使用
$ms = new ManagementSystem();
// 添加员工
$t1 = new Teacher("Tom", 35, "Male", "T001", "Math", 5000, ["Algebra", "Geometry"]);
$t2 = new Teacher("Mary", 30, "Female", "T002", "English", 4500, ["Literature", "Writing"]);
$s1 = new Staff("John", 25, "Male", "S001", "Security", 3000, "Guarding");
$s2 = new Staff("Lisa", 28, "Female", "S002", "Cafeteria", 3500, "Cooking");
$ms->add_employee($t1);
$ms->add_employee($t2);
$ms->add_employee($s1);
$ms->add_employee($s2);
// 查询员工
$e = $ms->search_employee("T002");
if ($e) {
echo "Employee found: {$e->name}<br>";
} else {
echo "Employee not found<br>";
}
// 列举员工
$ms->list_employees();
// 删除员工
$ms->remove_employee($s2);
// 列举员工
$ms->list_employees();
```
在上面的示例代码中,我们使用PHP定义了一个`Person`类作为基类,包含了姓名、年龄和性别等基本属性。然后我们定义了`Employee`类,作为一个员工类,继承了`Person`类的属性,并添加了员工的工号、部门和工资等属性。
之后我们又定义了`Teacher`类和`Staff`类,分别表示教师和职员,它们都是`Employee`类的子类,并添加了各自特有的属性。
最后我们定义了一个`ManagementSystem`类,作为员工管理系统,包含了添加、删除、查询和列举员工等方法。
我们在示例代码中通过实例化这些类,并调用相关方法,来演示了这个基本的教职员工管理系统的使用。您可以在此基础上进行扩展和完善,以满足自己的实际需求。
相关推荐
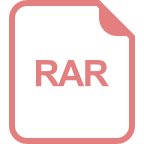
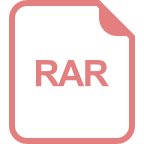
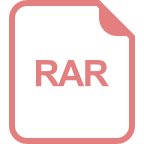
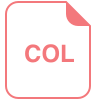
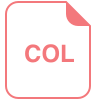
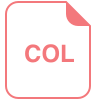
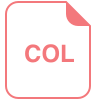
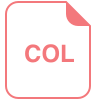









