linux shared内存释放
时间: 2023-10-31 16:15:08 浏览: 91
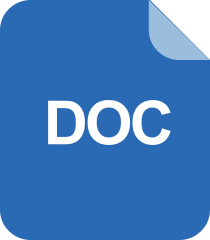
Linux 共享内存
在Linux中,可以使用shmctl函数释放共享内存。shmctl函数的原型如下:
```c
#include <sys/shm.h>
int shmctl(int shmid, int cmd, struct shmid_ds *buf);
```
其中,shmid是共享内存的标识符,cmd是要执行的操作(例如IPC_RMID,表示删除共享内存),buf是一个指向共享内存数据结构的指针。
具体步骤如下:
1. 获取共享内存的标识符(shmid)。
2. 调用shmctl函数,传入IPC_RMID命令,释放共享内存。
3. 若共享内存已经没有进程使用,则释放其占用的内存空间。
以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
int main()
{
int shmid;
key_t key;
char *shm;
/* 创建共享内存 */
key = ftok(".", 'a');
shmid = shmget(key, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(1);
}
/* 获取共享内存的指针 */
shm = shmat(shmid, NULL, 0);
if (shm == (char *)-1) {
perror("shmat");
exit(1);
}
/* 向共享内存中写入数据 */
sprintf(shm, "Hello, world!");
/* 删除共享内存 */
if (shmctl(shmid, IPC_RMID, NULL) == -1) {
perror("shmctl");
exit(1);
}
/* 输出共享内存中的数据 */
printf("Shared memory contains: %s\n", shm);
/* 分离共享内存 */
if (shmdt(shm) == -1) {
perror("shmdt");
exit(1);
}
return 0;
}
```
在上面的代码中,我们创建了一个大小为SHM_SIZE的共享内存,写入了一些数据,然后释放了该共享内存,最后输出共享内存中的数据。注意,释放共享内存后,不能再使用shmat函数获得共享内存的指针。
阅读全文
相关推荐
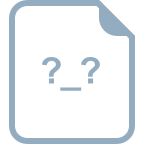

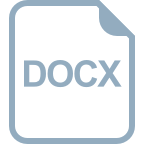
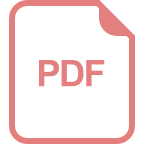
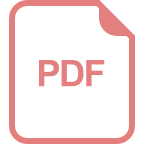
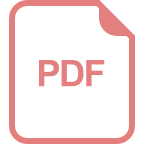
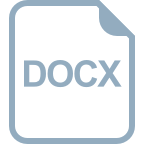
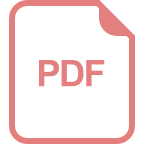
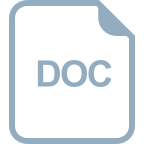
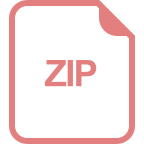
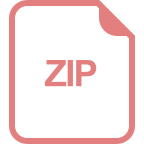
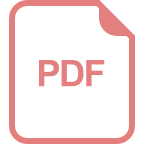
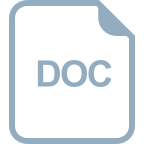
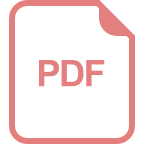
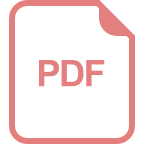
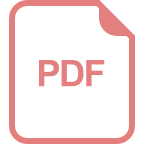