利用python实现植物大战僵尸游戏
时间: 2023-08-24 10:07:04 浏览: 258
实现植物大战僵尸游戏需要一定的游戏开发经验和技术,需要使用一些游戏引擎和库。在Python中可以使用Pygame等游戏引擎来实现这个游戏。
以下是一个简单的实现植物大战僵尸游戏的示例代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 游戏窗口大小
window_width = 800
window_height = 600
# 设置窗口标题
pygame.display.set_caption("植物大战僵尸")
# 加载背景音乐
pygame.mixer.music.load("background_music.mp3")
pygame.mixer.music.set_volume(0.5)
pygame.mixer.music.play(-1)
# 加载音效
sun_sound = pygame.mixer.Sound("sun_sound.wav")
zombie_sound = pygame.mixer.Sound("zombie_sound.wav")
plant_sound = pygame.mixer.Sound("plant_sound.wav")
# 加载图片
background_image = pygame.image.load("background_image.jpg")
sunflower_image = pygame.image.load("sunflower_image.png")
peashooter_image = pygame.image.load("peashooter_image.png")
sun_image = pygame.image.load("sun_image.png")
zombie_image = pygame.image.load("zombie_image.png")
pea_image = pygame.image.load("pea_image.png")
# 设置字体
font = pygame.font.Font(None, 36)
# 定义植物类
class Plant(pygame.sprite.Sprite):
def __init__(self, x, y, image):
super().__init__()
self.image = image
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
self.health = 100
def update(self):
pass
# 定义太阳花类
class Sunflower(Plant):
def __init__(self, x, y):
super().__init__(x, y, sunflower_image)
self.sun_timer = pygame.time.get_ticks()
def update(self):
current_time = pygame.time.get_ticks()
if current_time - self.sun_timer >= 10000:
self.sun_timer = current_time
sun = Sun(random.randint(self.rect.x, self.rect.x + self.rect.width), self.rect.y + self.rect.height)
all_sprites.add(sun)
suns.add(sun)
# 定义豌豆射手类
class Peashooter(Plant):
def __init__(self, x, y):
super().__init__(x, y, peashooter_image)
self.shoot_timer = pygame.time.get_ticks()
def update(self):
current_time = pygame.time.get_ticks()
if current_time - self.shoot_timer >= 1000:
self.shoot_timer = current_time
pea = Pea(self.rect.x + self.rect.width, self.rect.y + self.rect.height // 2)
all_sprites.add(pea)
peas.add(pea)
# 定义阳光类
class Sun(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.image = sun_image
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
def update(self):
self.rect.y += 2
if self.rect.y >= window_height:
self.kill()
# 定义豌豆类
class Pea(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.image = pea_image
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
def update(self):
self.rect.x += 5
for zombie in zombies:
if pygame.sprite.collide_rect(self, zombie):
zombie.health -= 10
self.kill()
if zombie.health <= 0:
zombie.kill()
zombie_sound.play()
# 定义僵尸类
class Zombie(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.image = zombie_image
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
self.health = 100
def update(self):
self.rect.x -= 2
if self.rect.x <= 0:
self.kill()
game_over()
# 定义游戏结束函数
def game_over():
pygame.mixer.music.stop()
zombie_sound.stop()
plant_sound.stop()
game_over_text = font.render("Game Over", True, (255, 0, 0))
game_over_rect = game_over_text.get_rect(center=(window_width // 2, window_height // 2))
screen.blit(game_over_text, game_over_rect)
pygame.display.update()
pygame.time.wait(3000)
pygame.quit()
exit()
# 创建屏幕
screen = pygame.display.set_mode((window_width, window_height))
# 定义精灵组
all_sprites = pygame.sprite.Group()
sunflowers = pygame.sprite.Group()
peashooters = pygame.sprite.Group()
suns = pygame.sprite.Group()
peas = pygame.sprite.Group()
zombies = pygame.sprite.Group()
# 创建植物
sunflower1 = Sunflower(100, 100)
all_sprites.add(sunflower1)
sunflowers.add(sunflower1)
peashooter1 = Peashooter(200, 200)
all_sprites.add(peashooter1)
peashooters.add(peashooter1)
# 游戏循环
clock = pygame.time.Clock()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
pos = pygame.mouse.get_pos()
if pos[1] < 100:
continue
x = pos[0] // 100 * 100
y = pos[1] // 100 * 100
if random.randint(1, 10) == 1:
sun = Sun(random.randint(x, x + 100), y)
all_sprites.add(sun)
suns.add(sun)
sun_sound.play()
else:
peashooter = Peashooter(x, y)
all_sprites.add(peashooter)
peashooters.add(peashooter)
plant_sound.play()
# 更新精灵组
all_sprites.update()
# 绘制背景
screen.blit(background_image, (0, 0))
# 绘制阳光值
sun_value = font.render("阳光值: " + str(len(suns)), True, (255, 255, 255))
screen.blit(sun_value, (10, 10))
# 绘制精灵组
all_sprites.draw(screen)
# 生成僵尸
if len(zombies) < 5 and random.randint(1, 100) == 1:
zombie = Zombie(window_width, random.randint(100, window_height - 100))
all_sprites.add(zombie)
zombies.add(zombie)
# 更新屏幕
pygame.display.update()
# 控制帧率
clock.tick(60)
```
这个示例代码实现了一个简单的植物大战僵尸游戏,包括植物、阳光、豌豆、僵尸等元素,并使用了Pygame游戏引擎来实现游戏逻辑和界面。
相关推荐
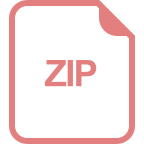
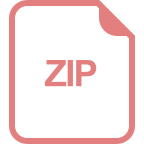
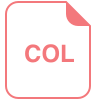
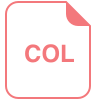
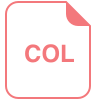
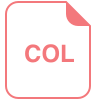
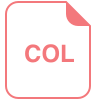









