用Python开发植物大战僵尸小游戏
时间: 2023-11-16 18:29:44 浏览: 48
好的,下面是一个简单的植物大战僵尸小游戏的实现,供你参考:
首先,我们需要安装 Pygame:
```
pip install pygame
```
然后,我们来实现游戏的主要逻辑:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小
size = (800, 600)
screen = pygame.display.set_mode(size)
# 设置游戏标题
pygame.display.set_caption("植物大战僵尸小游戏")
# 加载背景图片
bg_image = pygame.image.load("background.png")
# 加载植物图片
plant_image = pygame.image.load("plant.png")
# 加载僵尸图片
zombie_image = pygame.image.load("zombie.png")
# 设置游戏字体
font = pygame.font.SysFont("arial", 30)
# 设置游戏分数
score = 0
# 设置游戏主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 绘制背景
screen.blit(bg_image, (0, 0))
# 绘制植物
screen.blit(plant_image, (50, 500))
# 生成僵尸
if random.randint(1, 100) == 1:
zombie_x = 800
zombie_y = random.randint(50, 550)
else:
zombie_x -= 5
# 绘制僵尸
screen.blit(zombie_image, (zombie_x, zombie_y))
# 检测碰撞
plant_rect = pygame.Rect(50, 500, plant_image.get_width(), plant_image.get_height())
zombie_rect = pygame.Rect(zombie_x, zombie_y, zombie_image.get_width(), zombie_image.get_height())
if plant_rect.colliderect(zombie_rect):
score += 1
zombie_x = -100
# 绘制分数
score_text = font.render("Score: " + str(score), True, (255, 255, 255))
screen.blit(score_text, (10, 10))
# 更新屏幕
pygame.display.flip()
# 退出Pygame
pygame.quit()
```
在这个小游戏中,我们使用 Pygame 实现了如下功能:
- 加载背景、植物、僵尸图片;
- 设置游戏窗口大小和标题;
- 在游戏窗口中绘制背景、植物、僵尸和分数;
- 当植物和僵尸碰撞时,增加分数;
- 检测玩家是否退出游戏。
你可以根据自己的需要,对这个小游戏进行修改和扩展,实现更多的功能。
相关推荐
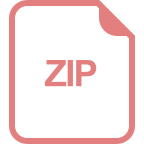
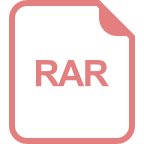














