__declspec(dllexport)使用例子
时间: 2023-09-14 19:09:47 浏览: 44
好的,以下是一个使用__declspec(dllexport)导出函数的例子:
首先,我们需要创建一个C++源文件,例如test.cpp,包含以下函数的实现:
```c++
#include <iostream>
extern "C" __declspec(dllexport) int add(int a, int b) {
return a + b;
}
extern "C" __declspec(dllexport) void print_name() {
std::cout << "My name is ChitGPT!" << std::endl;
}
```
这里使用了extern "C"修饰函数,以确保函数以C语言的命名约定导出。然后,使用__declspec(dllexport)修饰函数,以将其导出为DLL的导出项。
然后,我们可以使用编译器将其编译为动态链接库,例如在Windows上使用Visual Studio编译器,我们可以使用以下命令:
```
cl /LD test.cpp
```
这将生成一个名为test.dll的动态链接库文件。
最后,我们可以在另一个C++程序中使用test.dll中的函数,例如:
```c++
#include <iostream>
#include <Windows.h>
typedef int (*AddFunc)(int, int);
typedef void (*PrintNameFunc)();
int main() {
HMODULE testDll = LoadLibrary("test.dll");
if (testDll == NULL) {
std::cout << "Failed to load test.dll" << std::endl;
return 1;
}
AddFunc add = (AddFunc)GetProcAddress(testDll, "add");
if (add == NULL) {
std::cout << "Failed to get address of add function" << std::endl;
return 1;
}
std::cout << "1 + 2 = " << add(1, 2) << std::endl;
PrintNameFunc printName = (PrintNameFunc)GetProcAddress(testDll, "print_name");
if (printName == NULL) {
std::cout << "Failed to get address of print_name function" << std::endl;
return 1;
}
printName();
FreeLibrary(testDll);
return 0;
}
```
这个程序首先使用LoadLibrary函数加载test.dll动态链接库,然后使用GetProcAddress函数获取test.dll中的add和print_name函数的地址,并调用它们。最后,使用FreeLibrary函数卸载动态链接库。
这就是一个使用__declspec(dllexport)导出函数的例子。
相关推荐
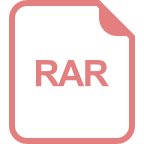
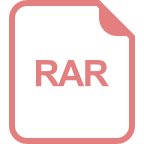














