对一幅添加了噪声的图像,用高斯滤波器在频率域分别实现一 个平滑和锐化的操作。使用Python代码
时间: 2024-05-01 07:20:03 浏览: 16
首先,我们需要导入一些必要的库:numpy、opencv-python和matplotlib。
```python
import numpy as np
import cv2
import matplotlib.pyplot as plt
```
接下来,我们可以加载一张图像并添加一些高斯噪声。
```python
# 加载图像
img = cv2.imread('test.jpg', 0)
# 添加高斯噪声
mean = 0
var = 100
sigma = var ** 0.5
gaussian = np.random.normal(mean, sigma, img.shape)
noisy_img = img + gaussian
```
接下来,我们可以将这个图像转换为频率域。为了做到这一点,我们可以使用傅里叶变换(`np.fft.fft2`)和将零频率分量移到频率域中心的函数(`np.fft.fftshift`)。
```python
# 傅里叶变换
f = np.fft.fft2(noisy_img)
# 将零频率分量移到频率域中心
fshift = np.fft.fftshift(f)
```
现在我们可以对频率域图像进行平滑和锐化操作了。对于平滑操作,我们可以使用一个低通滤波器,例如高斯滤波器。对于锐化操作,我们可以使用一个高通滤波器,例如拉普拉斯滤波器。在这里,我们将使用高斯滤波器来做平滑操作,使用拉普拉斯滤波器来做锐化操作。
```python
# 高斯滤波
kernel_size = 5
sigma = 1.5
kernel = cv2.getGaussianKernel(kernel_size, sigma)
kernel = kernel * kernel.T
smoothed_f = np.copy(fshift)
smoothed_f[:, :] = cv2.filter2D(np.real(smoothed_f), -1, kernel) + 1j * cv2.filter2D(np.imag(smoothed_f), -1, kernel)
# 拉普拉斯滤波
laplacian_kernel = np.array([[0, 1, 0], [1, -4, 1], [0, 1, 0]])
sharpened_f = np.copy(fshift)
sharpened_f[:, :] = cv2.filter2D(np.real(sharpened_f), -1, laplacian_kernel) + 1j * cv2.filter2D(np.imag(sharpened_f), -1, laplacian_kernel)
```
最后,我们可以将平滑和锐化操作后的频率域图像转换回空间域,并显示它们。
```python
# 将平滑和锐化操作后的频率域图像转换回空间域
smoothed_img = np.fft.ifft2(np.fft.ifftshift(smoothed_f))
smoothed_img = np.real(smoothed_img).astype(np.uint8)
sharpened_img = np.fft.ifft2(np.fft.ifftshift(sharpened_f))
sharpened_img = np.real(sharpened_img).astype(np.uint8)
# 显示结果
plt.figure(figsize=(10, 8))
plt.subplot(2, 2, 1)
plt.imshow(img, cmap='gray')
plt.title('Original Image')
plt.subplot(2, 2, 2)
plt.imshow(noisy_img, cmap='gray')
plt.title('Noisy Image')
plt.subplot(2, 2, 3)
plt.imshow(smoothed_img, cmap='gray')
plt.title('Smoothed Image')
plt.subplot(2, 2, 4)
plt.imshow(sharpened_img, cmap='gray')
plt.title('Sharpened Image')
plt.show()
```
完整的代码如下:
```python
import numpy as np
import cv2
import matplotlib.pyplot as plt
# 加载图像
img = cv2.imread('test.jpg', 0)
# 添加高斯噪声
mean = 0
var = 100
sigma = var ** 0.5
gaussian = np.random.normal(mean, sigma, img.shape)
noisy_img = img + gaussian
# 傅里叶变换
f = np.fft.fft2(noisy_img)
# 将零频率分量移到频率域中心
fshift = np.fft.fftshift(f)
# 高斯滤波
kernel_size = 5
sigma = 1.5
kernel = cv2.getGaussianKernel(kernel_size, sigma)
kernel = kernel * kernel.T
smoothed_f = np.copy(fshift)
smoothed_f[:, :] = cv2.filter2D(np.real(smoothed_f), -1, kernel) + 1j * cv2.filter2D(np.imag(smoothed_f), -1, kernel)
# 拉普拉斯滤波
laplacian_kernel = np.array([[0, 1, 0], [1, -4, 1], [0, 1, 0]])
sharpened_f = np.copy(fshift)
sharpened_f[:, :] = cv2.filter2D(np.real(sharpened_f), -1, laplacian_kernel) + 1j * cv2.filter2D(np.imag(sharpened_f), -1, laplacian_kernel)
# 将平滑和锐化操作后的频率域图像转换回空间域
smoothed_img = np.fft.ifft2(np.fft.ifftshift(smoothed_f))
smoothed_img = np.real(smoothed_img).astype(np.uint8)
sharpened_img = np.fft.ifft2(np.fft.ifftshift(sharpened_f))
sharpened_img = np.real(sharpened_img).astype(np.uint8)
# 显示结果
plt.figure(figsize=(10, 8))
plt.subplot(2, 2, 1)
plt.imshow(img, cmap='gray')
plt.title('Original Image')
plt.subplot(2, 2, 2)
plt.imshow(noisy_img, cmap='gray')
plt.title('Noisy Image')
plt.subplot(2, 2, 3)
plt.imshow(smoothed_img, cmap='gray')
plt.title('Smoothed Image')
plt.subplot(2, 2, 4)
plt.imshow(sharpened_img, cmap='gray')
plt.title('Sharpened Image')
plt.show()
```
相关推荐
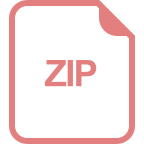
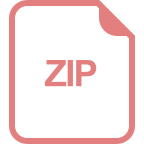
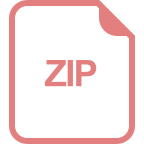














