stm32f10x蓝牙串口通信例程
时间: 2023-10-07 20:07:56 浏览: 100
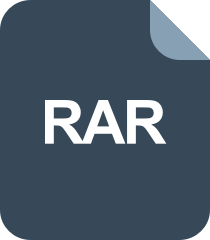
stm32 串口通讯案例
以下是一个简单的STM32F10x蓝牙串口通信例程,使用USART1和HC-05蓝牙模块。在此示例中,MCU将接收到的数据发送回蓝牙模块,并将蓝牙模块发送的数据发送到串口1。
```c
#include "stm32f10x.h"
void USART1_Init(void);
void Delay_ms(uint32_t nCount);
int main(void)
{
USART1_Init();
GPIO_InitTypeDef GPIO_InitStructure;
// 使能GPIOA时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// 配置PA9为复用推挽输出
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置PA10为浮空输入
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 使能USART1
USART_Cmd(USART1, ENABLE);
while(1)
{
// 接收蓝牙模块发送的数据
if(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) != RESET)
{
USART_SendData(USART1, USART_ReceiveData(USART1));
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
}
// 发送从串口接收到的数据到蓝牙模块
if(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) != RESET)
{
USART_SendData(USART1, USART_ReceiveData(USART1));
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
}
Delay_ms(10);
}
}
void USART1_Init(void)
{
// 使能USART1时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
// 配置USART1引脚
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1参数
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 使能USART1
USART_Cmd(USART1, ENABLE);
}
void Delay_ms(uint32_t nCount)
{
for(uint32_t i=0; i<nCount*1000; i++);
}
```
注意:这只是一个简单的示例,实际应用中可能需要添加更多的代码来处理接收到的数据、检查错误等。
阅读全文
相关推荐
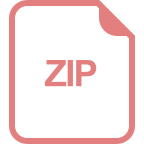
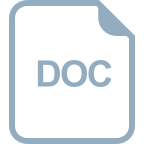

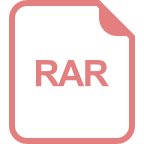
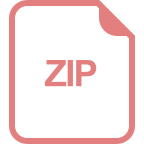
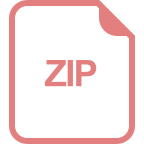
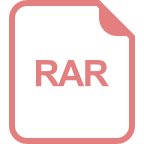
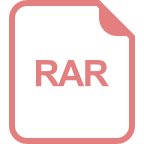
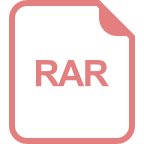
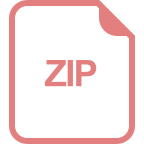
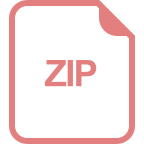
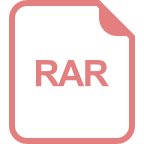
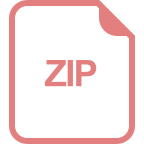
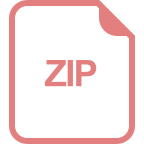
