websocket: close 1000 (normal)
时间: 2024-06-05 16:06:33 浏览: 172
As an AI language model, I don't have the context to provide a specific answer. However, in general, WebSocket is a communication protocol that allows real-time, two-way communication between a client and a server over a single, long-lived connection. The "close 1000 (normal)" message indicates that the WebSocket connection has been closed normally, without any error or abnormal termination. The number "1000" represents the WebSocket status code for a normal closure. When a WebSocket connection is closed, both the client and the server receive the close message, and any subsequent messages sent over the connection will not be delivered.
相关问题
C++ websocket
要在 C++ 中实现 WebSocket,您可以使用现有的 WebSocket 库,如 Boost.Beast 或 uWebSockets。这些库提供了 WebSocket 协议的实现,并允许您创建 WebSocket 客户端和服务器。
以下是使用 Boost.Beast 创建 WebSocket 客户端的示例代码:
```c++
#include <boost/beast/core.hpp>
#include <boost/beast/websocket.hpp>
#include <boost/asio/ip/tcp.hpp>
#include <iostream>
#include <cstdlib>
#include <string>
namespace beast = boost::beast; // from <boost/beast.hpp>
namespace http = beast::http; // from <boost/beast/http.hpp>
namespace websocket = beast::websocket; // from <boost/beast/websocket.hpp>
namespace net = boost::asio; // from <boost/asio.hpp>
using tcp = boost::asio::ip::tcp; // from <boost/asio/ip/tcp.hpp>
int main(int argc, char** argv)
{
try
{
// Check command line arguments.
if (argc != 4)
{
std::cerr <<
"Usage: websocket-client-sync <host> <port> <text>\n" <<
"Example:\n" <<
" websocket-client-sync echo.websocket.org 80 \"Hello, world!\"\n";
return EXIT_FAILURE;
}
auto const host = argv[1];
auto const port = argv[2];
auto const text = argv[3];
// The io_context is required for all I/O
net::io_context ioc;
// These objects perform our I/O
tcp::resolver resolver{ioc};
websocket::stream<tcp::socket> ws{ioc};
// Look up the domain name
auto const results = resolver.resolve(host, port);
// Make the connection on the IP address we get from a lookup
auto ep = net::connect(ws.next_layer(), results);
// Set up the WebSocket handshake
ws.handshake(host, "/", beast::multi_buffer());
// Send the message
ws.write(net::buffer(std::string{text}));
// This buffer will hold the incoming message
beast::flat_buffer buffer;
// Read a message into our buffer
ws.read(buffer);
// Close the WebSocket connection
ws.close(websocket::close_code::normal);
// If we get here then the connection is closed gracefully
// The buffers() function helps print a ConstBufferSequence
std::cout << beast::buffers(buffer.data()) << std::endl;
// Exit with success
return EXIT_SUCCESS;
}
catch (std::exception const& e)
{
std::cerr << "Error: " << e.what() << std::endl;
return EXIT_FAILURE;
}
}
```
该示例使用 Boost.Beast 创建 WebSocket 客户端,连接到指定的 WebSocket 服务器并发送一条消息。接收到服务器的响应后,关闭 WebSocket 连接并打印出响应消息。
c++ websocket
要在 C++ 中实现 WebSocket,您可以使用现有的 WebSocket 库,如 Boost.Beast 或 uWebSockets。这些库提供了 WebSocket 协议的实现,并允许您创建 WebSocket 客户端和服务器。
以下是使用 Boost.Beast 创建 WebSocket 客户端的示例代码:
```c++
#include <boost/beast/core.hpp>
#include <boost/beast/websocket.hpp>
#include <boost/asio/ip/tcp.hpp>
#include <iostream>
#include <cstdlib>
#include <string>
namespace beast = boost::beast; // from <boost/beast.hpp>
namespace http = beast::http; // from <boost/beast/http.hpp>
namespace websocket = beast::websocket; // from <boost/beast/websocket.hpp>
namespace net = boost::asio; // from <boost/asio.hpp>
using tcp = boost::asio::ip::tcp; // from <boost/asio/ip/tcp.hpp>
int main(int argc, char** argv)
{
try
{
// Check command line arguments.
if (argc != 4)
{
std::cerr <<
"Usage: websocket-client-sync <host> <port> <text>\n" <<
"Example:\n" <<
" websocket-client-sync echo.websocket.org 80 \"Hello, world!\"\n";
return EXIT_FAILURE;
}
auto const host = argv[1];
auto const port = argv[2];
auto const text = argv[3];
// The io_context is required for all I/O
net::io_context ioc;
// These objects perform our I/O
tcp::resolver resolver{ioc};
websocket::stream<tcp::socket> ws{ioc};
// Look up the domain name
auto const results = resolver.resolve(host, port);
// Make the connection on the IP address we get from a lookup
auto ep = net::connect(ws.next_layer(), results);
// Set up the WebSocket handshake
ws.handshake(host, "/", beast::multi_buffer());
// Send the message
ws.write(net::buffer(std::string{text}));
// This buffer will hold the incoming message
beast::flat_buffer buffer;
// Read a message into our buffer
ws.read(buffer);
// Close the WebSocket connection
ws.close(websocket::close_code::normal);
// If we get here then the connection is closed gracefully
// The buffers() function helps print a ConstBufferSequence
std::cout << beast::buffers(buffer.data()) << std::endl;
// Exit with success
return EXIT_SUCCESS;
}
catch (std::exception const& e)
{
std::cerr << "Error: " << e.what() << std::endl;
return EXIT_FAILURE;
}
}
```
该示例使用 Boost.Beast 创建 WebSocket 客户端,连接到指定的 WebSocket 服务器并发送一条消息。接收到服务器的响应后,关闭 WebSocket 连接并打印出响应消息。
阅读全文
相关推荐
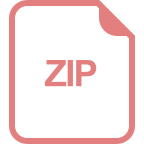
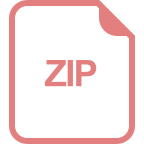
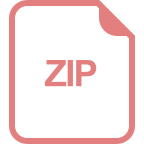
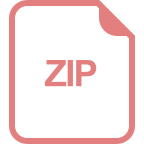
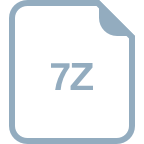
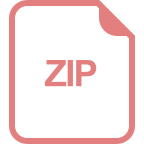
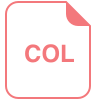
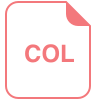
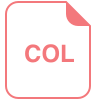







