使用stream流对List<String>进行排序
时间: 2024-03-13 19:20:04 浏览: 66
可以使用以下代码对 List<String> 进行排序:
```java
List<String> list = Arrays.asList("apple", "banana", "orange", "grape");
List<String> sortedList = list.stream().sorted().collect(Collectors.toList());
```
上面的代码将 List<String> 中的元素按字母顺序进行排序,并将结果存储在 sortedList 中。使用 stream 流的 sorted() 方法进行排序,最后使用 collect() 方法将排序后的元素收集到一个新的 List 中。
相关问题
使用stream流对 Map<String,List<SafetyQuestionVo>> list.size 进行排序
可以使用Java 8的Stream API对Map<String,List<SafetyQuestionVo>>中的List的size进行排序,代码如下:
```
Map<String, List<SafetyQuestionVo>> map = ...; // 假设已经有一个Map对象
List<Map.Entry<String, List<SafetyQuestionVo>>> sortedList = map.entrySet().stream()
.sorted(Comparator.comparingInt(entry -> entry.getValue().size()))
.collect(Collectors.toList());
// sortedList中就是按照List的size从小到大排序后的Map.Entry对象列表
```
代码解析:
- 首先调用Map的entrySet()方法,将Map转换为Set<Map.Entry>对象。
- 然后调用Stream的sorted()方法,传入一个Comparator对象,该对象根据List的size进行比较。Comparator.comparingInt()方法是Java 8新增的方法,用于返回一个基于整数的比较器,更加高效。
- 最后调用Stream的collect()方法,将排序后的Map.Entry对象收集到一个List中。在这里使用了Collectors.toList()方法,可以将Stream中的元素收集到一个List对象中。
使用stream流对 Map<String,List<Object>> list.size 进行排序
首先需要明确一点,Map<String,List<Object>> 中的value是List类型,而List是有大小的,所以不能直接使用size对Map进行排序。您可能想要对Map中value的List的大小进行排序,如果是这样的话,可以使用下面的代码:
```
Map<String, List<Object>> map = new HashMap<>();
// 添加数据到map中
List<Map.Entry<String, List<Object>>> list = new ArrayList<>(map.entrySet());
list.stream()
.sorted(Comparator.comparingInt(e -> e.getValue().size()))
.collect(Collectors.toList());
```
在上面的代码中,我们首先将Map转换为List类型,然后使用stream方法对List进行排序。Comparator.comparingInt方法用于指定排序的规则,这里是根据每个Map.Entry的value的List大小进行排序,然后使用collect方法将排序后的结果转换为List类型。
需要注意的是,这里的排序是升序排序,如果需要降序排序,可以将comparingInt改为comparingInt().reversed()。
阅读全文
相关推荐
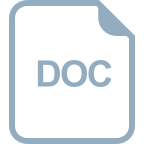
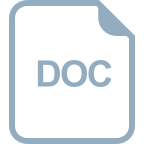
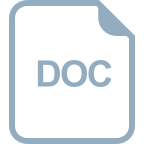










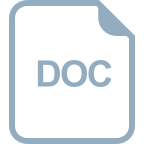
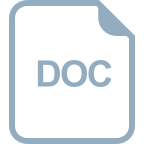
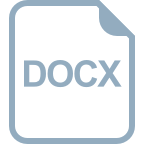