c++ std::vector<uint8_t> AA = {72, 101, 108, 108, 111};将AA内容输出一个是字符串“72,101,108,108,111”
时间: 2023-09-20 17:03:08 浏览: 44
可以使用以下代码将`std::vector<uint8_t>`类型的`AA`转换为字符串输出:
```c++
#include <iostream>
#include <vector>
#include <cstdint>
#include <string>
int main() {
std::vector<uint8_t> AA = {72, 101, 108, 108, 111}; // Hello
std::string str;
for (int i = 0; i < AA.size(); i++) {
str += std::to_string(AA[i]);
if (i < AA.size() - 1) {
str += ",";
}
}
std::cout << str << std::endl;
return 0;
}
```
输出结果为:
```
72,101,108,108,111
```
这里使用了一个for循环,遍历`AA`中的每一个元素,并将其转换为字符串类型,然后加入到一个字符串`str`中。在每个元素的后面加入一个逗号,除了最后一个元素之外。这里的`std::to_string()`函数可以将整数类型转换为字符串类型。
相关问题
c++ std::vector<uint8_t> AA;将AA的内容以一个字符串的形式输出
可以使用以下代码将`std::vector<uint8_t>`类型的`AA`转换为字符串输出:
```c++
#include <iostream>
#include <vector>
#include <cstdint>
#include <string>
int main() {
std::vector<uint8_t> AA = {72, 101, 108, 108, 111}; // Hello
std::string str(AA.begin(), AA.end());
std::cout << str << std::endl;
return 0;
}
```
输出结果为:
```
Hello
```
这里利用了`std::string`的构造函数,该构造函数接受两个迭代器作为参数,第一个迭代器指向字符串的起始位置,第二个迭代器指向字符串的结束位置(不包括结束位置本身),这里的`AA.begin()`和`AA.end()`分别表示`AA`的起始位置和结束位置。由于`std::vector<uint8_t>`中存储的是`uint8_t`类型的数据,所以需要将其转换为字符类型,这里使用的是字符的ASCII码,即72表示字符'H',101表示字符'e',以此类推。
c++如何从“std::vector<uint8_t,std::allocator<uint8_t>>”转换为“uint8_t”
在C++中,你可以使用下标运算符([])或者at()函数来访问std::vector容器中的元素。如果你想将一个std::vector<uint8_t,std::allocator<uint8_t>>类型的向量转换为uint8_t类型,你可以通过以下方式实现:
1. 使用下标运算符([]):
```cpp
std::vector<uint8_t,std::allocator<uint8_t>> vec;
// 假设vec已经被填充了一些元素
uint8_t element = vec[0]; // 获取第一个元素
```
2. 使用at()函数:
```cpp
std::vector<uint8_t,std::allocator<uint8_t>> vec;
// 假设vec已经被填充了一些元素
uint8_t element = vec.at(0); // 获取第一个元素
```
这样就可以将std::vector<uint8_t,std::allocator<uint8_t>>类型的向量中的元素转换为uint8_t类型。
相关推荐
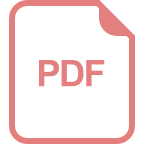
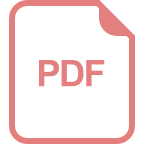
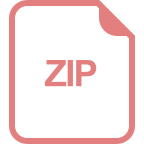












