c++ std::vector<uint8_t> AA = {0x11, 0x22, 0x33,};将AA内容输出一个是字符串“0x11,0x22,0x33”
时间: 2024-03-28 14:42:20 浏览: 134
可以使用循环遍历 `AA` 中的元素,将其转换成字符串后拼接起来,最后输出即可。以下是示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cstdint>
#include <sstream>
int main() {
std::vector<uint8_t> AA = {0x11, 0x22, 0x33};
std::stringstream ss;
for (auto it = AA.begin(); it != AA.end(); ++it) {
ss << "0x" << std::hex << static_cast<int>(*it);
if (it != AA.end() - 1) {
ss << ",";
}
}
std::cout << ss.str() << std::endl; // 输出:0x11,0x22,0x33
return 0;
}
```
代码中使用了 `std::stringstream` 类来拼接字符串,`std::hex` 是用来指定输出的数字以十六进制形式显示的格式控制符。
相关问题
c++ std::vector<uint8_t> AA = {0x11, 0x22, 0x33,};不使用std::stringstream的方式,将AA内容输出一个是字符串“0x11,0x22,0x33”
可以使用循环遍历 `AA` 中的元素,将其转换成字符串后拼接起来,最后输出即可。以下是示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cstdint>
#include <string>
int main() {
std::vector<uint8_t> AA = {0x11, 0x22, 0x33};
std::string s;
for (auto it = AA.begin(); it != AA.end(); ++it) {
char buf[5];
sprintf(buf, "0x%02x", static_cast<int>(*it));
s += buf;
if (it != AA.end() - 1) {
s += ",";
}
}
std::cout << s << std::endl; // 输出:0x11,0x22,0x33
return 0;
}
```
代码中使用了 `sprintf` 函数来将数字转换成字符串,`"0x%02x"` 是指定输出的数字以十六进制形式显示,且宽度为2位,不足的前面补0。
std::vector<uint8_t>数据写入文件
可以使用文件流和迭代器来实现将 std::vector<uint8_t> 写入文件的操作,示例如下:
```c++
#include <iostream>
#include <fstream>
#include <vector>
int main() {
std::vector<uint8_t> data = {0x12, 0x34, 0x56, 0x78};
std::ofstream fout("data.bin", std::ios::binary);
if (!fout.is_open()) {
std::cerr << "Failed to open file!" << std::endl;
return -1;
}
std::copy(data.begin(), data.end(), std::ostreambuf_iterator<char>(fout));
fout.close();
return 0;
}
```
在上述代码中,使用 `std::ofstream` 打开文件,并指定以二进制模式打开,然后使用 `std::copy` 将 vector 中的数据复制到文件中。最后关闭文件。
阅读全文
相关推荐
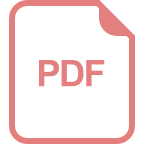
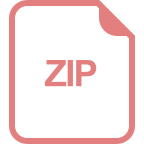














