c++ powerpoint 获取文本框中所有的公式 实例
时间: 2024-03-10 21:51:21 浏览: 124
在 C++ Powerpoint 中获取文本框中的所有公式,可以使用 Microsoft Office 提供的 COM 接口来实现。具体步骤如下:
1. 首先需要在 C++ 代码中引入 Office 相关的头文件和库文件,比如:
```c++
#include <Windows.h>
#include <ole2.h>
#include <comutil.h>
#include <comdef.h>
#include <atlbase.h>
#pragma comment(lib, "ole32.lib")
#pragma comment(lib, "comsupp.lib")
```
2. 接着需要创建一个 PowerPoint 应用程序对象,代码如下:
```c++
HRESULT hr;
CLSID clsid;
hr = CLSIDFromProgID(L"PowerPoint.Application", &clsid);
if (FAILED(hr)) {
// 创建对象失败
return -1;
}
CComPtr<IDispatch> pAppDisp;
hr = CoCreateInstance(clsid, NULL, CLSCTX_LOCAL_SERVER, IID_PPV_ARGS(&pAppDisp));
if (FAILED(hr)) {
// 创建对象失败
return -1;
}
CComQIPtr<_Application> pPPTApp(pAppDisp);
if (pPPTApp == nullptr) {
// 转换对象类型失败
return -1;
}
// 设置 PowerPoint 应用程序的 Visible 属性为 True,以便在界面上显示
pPPTApp->put_Visible(VARIANT_TRUE);
```
3. 打开一个已有的演示文稿,代码如下:
```c++
CComPtr<_Presentation> pPPTPresentation;
hr = pPPTApp->Presentations->Open(L"path/to/your/presentation.pptx", VARIANT_FALSE, VARIANT_FALSE, VARIANT_FALSE, &pPPTPresentation);
if (FAILED(hr)) {
// 打开演示文稿失败
return -1;
}
```
4. 遍历演示文稿中的所有幻灯片,查找其中的文本框,并获取文本框中的所有公式,代码如下:
```c++
// 遍历演示文稿中的所有幻灯片
int slideCount = pPPTPresentation->Slides->Count;
for (int i = 1; i <= slideCount; i++) {
CComPtr<_Slide> pSlide;
hr = pPPTPresentation->Slides->Item(i, &pSlide);
if (FAILED(hr)) {
// 获取幻灯片失败
continue;
}
// 遍历幻灯片中的所有 Shapes 对象,查找文本框
CComPtr<Shapes> pShapes;
hr = pSlide->get_Shapes(&pShapes);
if (FAILED(hr)) {
// 获取幻灯片上的 Shapes 对象失败
continue;
}
int shapeCount = pShapes->Count;
for (int j = 1; j <= shapeCount; j++) {
CComPtr<Shape> pShape;
hr = pShapes->Item(j, &pShape);
if (FAILED(hr)) {
// 获取 Shape 对象失败
continue;
}
// 判断当前 Shape 是否是文本框
MsoShapeType shapeType;
hr = pShape->get_Type(&shapeType);
if (FAILED(hr) || shapeType != msoTextBox) {
// 当前 Shape 不是文本框
continue;
}
// 获取文本框中的 TextFrame 对象
CComPtr<TextFrame> pTextFrame;
hr = pShape->get_TextFrame(&pTextFrame);
if (FAILED(hr)) {
// 获取 TextFrame 对象失败
continue;
}
// 获取 TextFrame 中的 TextRange 对象
CComPtr<TextRange> pTextRange;
hr = pTextFrame->get_TextRange(&pTextRange);
if (FAILED(hr)) {
// 获取 TextRange 对象失败
continue;
}
// 遍历 TextRange 中的所有 TextRange2 对象,查找公式
CComPtr<TextRange2> pTextRange2;
hr = pTextRange->QueryInterface(IID_PPV_ARGS(&pTextRange2));
if (FAILED(hr)) {
// 获取 TextRange2 对象失败
continue;
}
long textRange2Count;
hr = pTextRange2->get_Characters()->get_Count(&textRange2Count);
if (FAILED(hr)) {
// 获取 TextRange2 中 TextRange 数量失败
continue;
}
for (long k = 1; k <= textRange2Count; k++) {
CComPtr<TextRange2> pSubTextRange2;
hr = pTextRange2->get_Characters(k, 1)->QueryInterface(IID_PPV_ARGS(&pSubTextRange2));
if (FAILED(hr)) {
// 获取 TextRange2 对象失败
continue;
}
MsoTextRange2Type textRange2Type;
hr = pSubTextRange2->get_TextRange2Type(&textRange2Type);
if (FAILED(hr) || textRange2Type != msoTextRange2TypeMath) {
// 当前 TextRange2 不是公式
continue;
}
// 获取公式字符串
BSTR formula;
hr = pSubTextRange2->get_Text(&formula);
if (FAILED(hr)) {
// 获取公式字符串失败
continue;
}
// TODO: 处理公式字符串
// ...
SysFreeString(formula);
}
}
}
```
需要注意的是,在使用 COM 接口时,需要手动管理对象的生命周期和释放资源,否则容易导致内存泄漏和程序崩溃等问题。
阅读全文
相关推荐
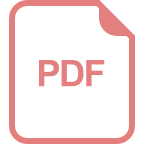
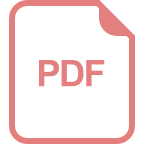
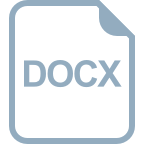





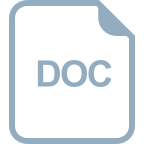
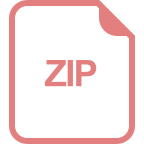
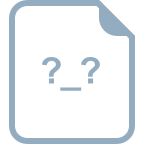
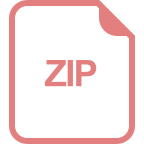
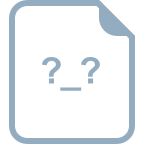
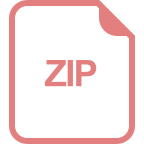