c++发送邮件的代码
时间: 2023-09-15 20:05:43 浏览: 137
### 回答1:
在 C 语言中,可以使用系统调用来发送电子邮件。 例如,可以使用 `sendmail` 命令来发送电子邮件。
下面是一个示例代码,展示了如何使用 `system` 函数来调用 `sendmail` 命令:
```
#include <stdio.h>
#include <stdlib.h>
int main() {
// 要发送的电子邮件的信息
char *to = "recipient@example.com";
char *subject = "Hello";
char *body = "This is a test email.";
// 将信息拼接成命令行参数的形式
char command[1024];
sprintf(command, "echo '%s' | sendmail -v -t -f sender@example.com -s '%s' %s", body, subject, to);
// 使用 system 函数来执行命令
int result = system(command);
if (result != 0) {
printf("Failed to send email\n");
return 1;
}
printf("Successfully sent email\n");
return 0;
}
```
这段代码会将一封电子邮件发送到 "recipient@example.com",主题是 "Hello",正文是 "This is a test email."。
需要注意的是,这段代码仅作为示例,并不能保证在所有环境下都能正常工作。
### 回答2:
发送邮件的代码可以使用Python的smtplib库来实现。具体步骤如下:
1. 首先,需要导入smtplib库:
```
import smtplib
```
2. 接下来,创建一个SMTP对象,并连接到邮件服务器:
```
smtp_server = smtplib.SMTP('smtp.example.com', 587) # 邮件服务器地址和端口号
smtp_server.starttls() # 启用TLS加密,如果服务器支持的话
smtp_server.login('your_email@example.com', 'your_password') # 登录邮箱账号和密码
```
3. 然后,创建一个包含邮件内容的消息对象:
```
from email.mime.text import MIMEText
subject = '邮件主题'
body = '邮件内容'
message = MIMEText(body, 'plain')
message['Subject'] = subject
message['From'] = 'your_email@example.com'
message['To'] = 'recipient_email@example.com'
```
4. 最后,发送邮件:
```
smtp_server.send_message(message)
smtp_server.quit()
```
以上代码会连接到指定的邮件服务器,并使用给定的邮箱账号和密码进行登录。创建一个包含邮件主题和内容的消息对象,并指定发件人和收件人的邮箱地址。然后使用SMTP对象发送邮件,并关闭连接。
注意,以上代码仅为示例代码,具体的邮件服务器地址、端口号和邮箱账号信息需要根据实际情况进行替换。
### 回答3:
发送邮件的代码可以使用各种编程语言来实现,例如Python、Java、C#等。下面以Python为例给出一个简单的发送邮件的代码示例:
```python
import smtplib
from email.mime.text import MIMEText
from email.header import Header
def send_email(subject, content, sender, receiver, smtp_server, smtp_port, username, password):
message = MIMEText(content, 'plain', 'utf-8') # 创建邮件正文
message['Subject'] = Header(subject, 'utf-8') # 设置邮件主题
message['From'] = sender # 设置发件人
message['To'] = receiver # 设置收件人
try:
smtp_obj = smtplib.SMTP_SSL(smtp_server, smtp_port) # 创建SMTP对象
smtp_obj.login(username, password) # 登录SMTP服务器
smtp_obj.sendmail(sender, receiver, message.as_string()) # 发送邮件
print("邮件发送成功")
except smtplib.SMTPException as e:
print("邮件发送失败:" + str(e))
# 示例用法
subject = "测试邮件"
content = "这是一封测试邮件。"
sender = "sender@example.com"
receiver = "receiver@example.com"
smtp_server = "smtp.example.com"
smtp_port = 465
username = "your_username"
password = "your_password"
send_email(subject, content, sender, receiver, smtp_server, smtp_port, username, password)
```
以上代码使用了Python内置的smtplib和email库来实现邮件的发送。通过设置发件人、收件人、主题、正文等信息,使用SMTP服务器和账号登录,然后调用`sendmail`方法发送邮件。
注意:实际使用时需要替换掉示例中的smtp_server、smtp_port、username和password等参数,确保它们与你的邮件服务器和账号信息相符。
阅读全文
相关推荐


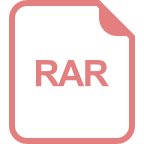






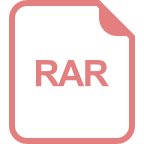





