利用python做一个贪吃蛇游戏
时间: 2023-07-18 20:16:29 浏览: 110
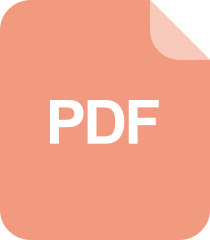
python 使用pygame工具包实现贪吃蛇游戏(多彩版)
好的,下面是一个简单的贪吃蛇游戏的Python代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 游戏界面大小
width = 800
height = 600
# 颜色定义
black = pygame.Color(0, 0, 0)
white = pygame.Color(255, 255, 255)
red = pygame.Color(255, 0, 0)
green = pygame.Color(0, 255, 0)
blue = pygame.Color(0, 0, 255)
# 设置游戏界面大小及标题
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption('贪吃蛇')
# 蛇的初始位置
snake_position = [100, 100]
# 蛇的初始长度
snake_body = [[100, 100], [90, 100], [80, 100]]
# 食物的初始位置
food_position = [random.randrange(1, (width // 10)) * 10, random.randrange(1, (height // 10)) * 10]
# 食物的状态(0:未被吃掉,1:已被吃掉)
food_status = 0
# 初始方向
direction = 'RIGHT'
change_direction = direction
# 游戏结束函数
def game_over():
pygame.quit()
exit()
# 主函数
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
change_direction = 'RIGHT'
if event.key == pygame.K_LEFT:
change_direction = 'LEFT'
if event.key == pygame.K_UP:
change_direction = 'UP'
if event.key == pygame.K_DOWN:
change_direction = 'DOWN'
if event.key == pygame.K_ESCAPE:
game_over()
# 确定蛇头的方向
if change_direction == 'RIGHT' and not direction == 'LEFT':
direction = 'RIGHT'
if change_direction == 'LEFT' and not direction == 'RIGHT':
direction = 'LEFT'
if change_direction == 'UP' and not direction == 'DOWN':
direction = 'UP'
if change_direction == 'DOWN' and not direction == 'UP':
direction = 'DOWN'
# 根据方向移动蛇头的位置
if direction == 'RIGHT':
snake_position[0] += 10
if direction == 'LEFT':
snake_position[0] -= 10
if direction == 'UP':
snake_position[1] -= 10
if direction == 'DOWN':
snake_position[1] += 10
# 增加蛇的长度
snake_body.insert(0, list(snake_position))
if snake_position == food_position:
food_status = 1
else:
snake_body.pop()
# 生成新的食物
if food_status == 1:
food_position = [random.randrange(1, (width // 10)) * 10, random.randrange(1, (height // 10)) * 10]
food_status = 0
# 绘制游戏界面
screen.fill(black)
for position in snake_body:
pygame.draw.rect(screen, white, pygame.Rect(position[0], position[1], 10, 10))
pygame.draw.rect(screen, green, pygame.Rect(food_position[0], food_position[1], 10, 10))
# 判断游戏结束
if snake_position[0] < 0 or snake_position[0] > width - 10:
game_over()
if snake_position[1] < 0 or snake_position[1] > height - 10:
game_over()
for block in snake_body[1:]:
if snake_position == block:
game_over()
# 更新游戏界面
pygame.display.update()
# 控制游戏速度
pygame.time.Clock().tick(20)
```
这段代码用pygame库实现了贪吃蛇游戏。你可以将这段代码保存到一个py文件中,然后在电脑上运行。
阅读全文
相关推荐
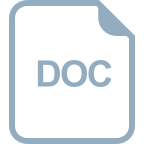
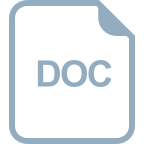




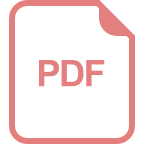
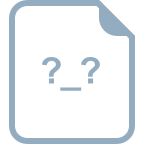
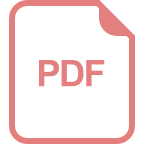
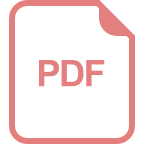
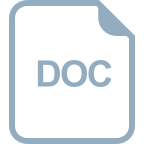





