AlertDialog.Builder dialog = null;
时间: 2024-05-01 13:22:17 浏览: 232
这段代码创建了一个用于构建对话框的AlertDialog.Builder对象,但是该对象还未被实例化,需要进一步的代码来实例化并设置对话框的属性。例如,可以使用下面的代码来创建一个简单的对话框:
```
dialog = new AlertDialog.Builder(context);
dialog.setTitle("提示");
dialog.setMessage("确定要删除吗?");
dialog.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// 点击确定按钮后执行的操作
}
});
dialog.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// 点击取消按钮后执行的操作
}
});
dialog.show();
```
这个对话框会显示一个标题为“提示”的对话框,内容为“确定要删除吗?”,并且有两个按钮:“确定”和“取消”。当用户点击确定或取消按钮时,对应的OnClickListener对象中的onClick方法会被调用。
相关问题
private fun showanbuKeyboard() { val builder = AlertDialog.Builder(this) val inflater = LayoutInflater.from(this) val view = inflater.inflate(R.layout.anbu, null, false) builder.setView(view) dialog = builder.create() dialog = Dialog(this, R.style.Transparent) as AlertDialog dialog.show() }怎么改为使用自定义的Dialog
要将上述代码改为使用自定义的 Dialog 类,可以按照以下步骤进行修改:
1. 首先,创建一个名为 CustomDialog 的类,继承自 Dialog 类。在 CustomDialog 类中,可以定义自己需要的属性和方法。
```kotlin
class CustomDialog(context: Context) : Dialog(context) {
// 定义自己需要的属性和方法
// ...
}
```
2. 在 showanbuKeyboard 函数中,使用 CustomDialog 类替换掉 AlertDialog 和 Dialog 类。修改代码如下:
```kotlin
private fun showanbuKeyboard() {
val inflater = LayoutInflater.from(this)
val view = inflater.inflate(R.layout.anbu, null, false)
// 使用自定义的 Dialog 类
val dialog = CustomDialog(this)
dialog.setContentView(view)
dialog.window?.setBackgroundDrawable(ColorDrawable(Color.TRANSPARENT))
dialog.show()
}
```
以上就是将原来使用 AlertDialog 和 Dialog 类的代码改为使用自定义的 Dialog 类的步骤。你可以根据自己的需求在 CustomDialog 类中添加额外的属性和方法。
private fun showanbuKeyboard() { val builder = AlertDialog.Builder(this) val inflater = LayoutInflater.from(this) val view = inflater.inflate(R.layout.anbu, null, false) builder.setView(view) dialog = builder.create() dialog = Dialog(this, R.style.Transparent) as AlertDialog dialog.show() }这段代码有什么问题
在这段代码中,存在两个问题:
1. 对话框的创建方式重复了:您在代码中使用了两种不同的方式创建了对话框实例。首先,您使用`AlertDialog.Builder`创建了一个对话框实例,并将其设置为`Dialog`类型的`dialog`变量。然后,您又创建了一个带有自定义样式的`Dialog`实例,并将其转换为`AlertDialog`类型的`dialog`变量。这导致前一个对话框实例被覆盖,无法使用。
2. `Dialog`和`AlertDialog`的混用:您在代码中混用了`Dialog`和`AlertDialog`,这可能会导致一些问题。如果您只需要显示一个简单的对话框,可以直接使用`AlertDialog.Builder`创建对话框。如果您需要更多的自定义选项,可以考虑使用自定义的`Dialog`类。
以下是修正后的代码:
```kotlin
private fun showAnbuKeyboard() {
val builder = AlertDialog.Builder(this)
val inflater = LayoutInflater.from(this)
val view = inflater.inflate(R.layout.anbu, null, false)
builder.setView(view)
val dialog = builder.create()
dialog.show()
}
```
在修正后的代码中,我们只使用了`AlertDialog.Builder`创建了一个对话框实例,并直接调用了`show()`方法来显示对话框。这样就避免了重复创建对话框的问题,并且统一使用了`AlertDialog`类来处理对话框。
希望能帮助到您!如果还有其他问题,请随时提问。
阅读全文
相关推荐







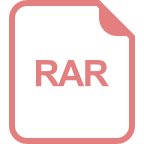





