UCS算法python
时间: 2023-11-18 19:56:28 浏览: 154
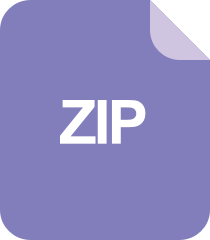
ucs:使用Python语言实现算法统一成本搜索(UCS)
UCS算法是一种使用优先级队列的最佳算法,用于遍历或搜索加权树、树结构或图的树搜索算法。在Python中,可以通过以下步骤实现UCS算法:
1. 定义节点类,包括节点名称、父节点、距离等属性。
2. 定义优先级队列,用于存储待扩展的节点。
3. 定义UCS算法函数,包括起始节点、目标节点和图等参数。
4. 初始化起始节点,并将其加入优先级队列。
5. 从优先级队列中取出距离起始节点最近的节点,并将其标记为已访问。
6. 遍历该节点的所有邻居节点,计算它们到起始节点的距离,并将它们加入优先级队列。
7. 重复步骤5和6,直到找到目标节点或者优先级队列为空。
以下是一个简单的UCS算法Python实现的示例代码:
```
import heapq
class Node:
def __init__(self, name):
self.name = name
self.parent = None
self.distance = float('inf')
self.visited = False
self.adjacent = {}
def __lt__(self, other):
return self.distance < other.distance
def ucs(start, goal, graph):
start.distance = 0
queue = [(0, start)]
while queue:
current_distance, current_node = heapq.heappop(queue)
if current_node == goal:
path = []
while current_node is not None:
path.append(current_node.name)
current_node = current_node.parent
return path[::-1]
current_node.visited = True
for neighbor, weight in current_node.adjacent.items():
if not neighbor.visited:
distance = current_distance + weight
if distance < neighbor.distance:
neighbor.distance = distance
neighbor.parent = current_node
heapq.heappush(queue, (distance, neighbor))
return None
```
阅读全文
相关推荐
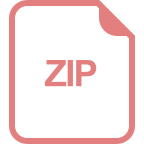


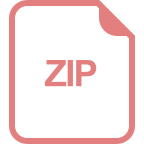
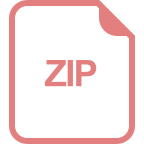
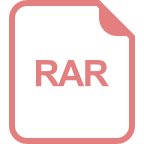
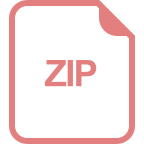
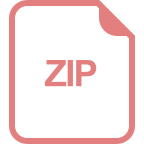
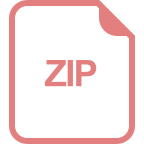
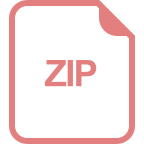
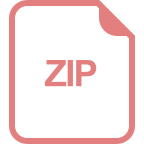
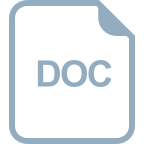
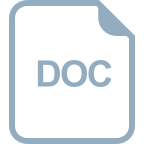


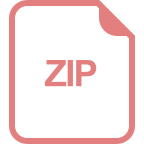