移动应用开发写出相应的activity和xml文件1.布局简易多媒体界面,包括上半部分音频播放功能和下半部分的视频播放功能。 a)音频播放部分包括:音频路径输入控件、文件选择按钮、播放按钮、暂停按钮、重播按钮和停止按钮。 b)视频播放部分包括:视频路径输入控件、文件选择按钮、播放按钮和视频播放区的SurfaceView(适合大小)。分别建立三个activity,实现音频和视频播放功能,要求实现重播、暂停、停止、播放功能
时间: 2024-03-07 13:48:58 浏览: 58
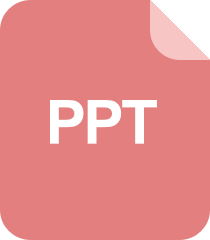
包含activity的java源文件-Android_Application_Presentation
好的,以下是相应的activity和xml文件,分别实现了音频播放、视频播放和音视频播放界面。
音频播放界面:
activity:
```java
public class AudioPlayerActivity extends AppCompatActivity {
private MediaPlayer mediaPlayer;
private Button playButton;
private Button pauseButton;
private Button stopButton;
private Button replayButton;
private EditText pathEditText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_audio_player);
pathEditText = findViewById(R.id.path_edit_text);
playButton = findViewById(R.id.play_button);
pauseButton = findViewById(R.id.pause_button);
stopButton = findViewById(R.id.stop_button);
replayButton = findViewById(R.id.replay_button);
playButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
play();
}
});
pauseButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
pause();
}
});
stopButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
stop();
}
});
replayButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
replay();
}
});
}
private void play() {
try {
if (mediaPlayer == null) {
mediaPlayer = new MediaPlayer();
}
mediaPlayer.reset();
mediaPlayer.setDataSource(pathEditText.getText().toString());
mediaPlayer.prepare();
mediaPlayer.start();
} catch (Exception e) {
e.printStackTrace();
}
}
private void pause() {
if (mediaPlayer != null && mediaPlayer.isPlaying()) {
mediaPlayer.pause();
}
}
private void stop() {
if (mediaPlayer != null) {
mediaPlayer.stop();
mediaPlayer.release();
mediaPlayer = null;
}
}
private void replay() {
if (mediaPlayer != null) {
mediaPlayer.seekTo(0);
mediaPlayer.start();
}
}
}
```
xml:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="20dp">
<EditText
android:id="@+id/path_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入音频文件路径" />
<Button
android:id="@+id/play_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="播放" />
<Button
android:id="@+id/pause_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="暂停" />
<Button
android:id="@+id/stop_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="停止" />
<Button
android:id="@+id/replay_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="重播" />
</LinearLayout>
```
视频播放界面:
activity:
```java
public class VideoPlayerActivity extends AppCompatActivity {
private MediaPlayer mediaPlayer;
private VideoView videoView;
private EditText pathEditText;
private Button playButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_video_player);
pathEditText = findViewById(R.id.path_edit_text);
playButton = findViewById(R.id.play_button);
videoView = findViewById(R.id.video_view);
playButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
play();
}
});
}
private void play() {
videoView.setVideoPath(pathEditText.getText().toString());
videoView.start();
}
}
```
xml:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="20dp">
<EditText
android:id="@+id/path_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入视频文件路径" />
<Button
android:id="@+id/play_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="播放" />
<VideoView
android:id="@+id/video_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
```
音视频播放界面:
activity:
```java
public class MediaPlayerActivity extends AppCompatActivity {
private MediaPlayer mediaPlayer;
private Button audioPlayButton;
private Button audioPauseButton;
private Button audioStopButton;
private Button audioReplayButton;
private EditText audioPathEditText;
private VideoView videoView;
private EditText videoPathEditText;
private Button videoPlayButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_media_player);
audioPathEditText = findViewById(R.id.audio_path_edit_text);
audioPlayButton = findViewById(R.id.audio_play_button);
audioPauseButton = findViewById(R.id.audio_pause_button);
audioStopButton = findViewById(R.id.audio_stop_button);
audioReplayButton = findViewById(R.id.audio_replay_button);
videoPathEditText = findViewById(R.id.video_path_edit_text);
videoPlayButton = findViewById(R.id.video_play_button);
videoView = findViewById(R.id.video_view);
audioPlayButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
playAudio();
}
});
audioPauseButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
pauseAudio();
}
});
audioStopButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
stopAudio();
}
});
audioReplayButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
replayAudio();
}
});
videoPlayButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
playVideo();
}
});
}
private void playAudio() {
try {
if (mediaPlayer == null) {
mediaPlayer = new MediaPlayer();
}
mediaPlayer.reset();
mediaPlayer.setDataSource(audioPathEditText.getText().toString());
mediaPlayer.prepare();
mediaPlayer.start();
} catch (Exception e) {
e.printStackTrace();
}
}
private void pauseAudio() {
if (mediaPlayer != null && mediaPlayer.isPlaying()) {
mediaPlayer.pause();
}
}
private void stopAudio() {
if (mediaPlayer != null) {
mediaPlayer.stop();
mediaPlayer.release();
mediaPlayer = null;
}
}
private void replayAudio() {
if (mediaPlayer != null) {
mediaPlayer.seekTo(0);
mediaPlayer.start();
}
}
private void playVideo() {
videoView.setVideoPath(videoPathEditText.getText().toString());
videoView.start();
}
}
```
xml:
```xml
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="20dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="音频播放" />
<EditText
android:id="@+id/audio_path_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入音频文件路径" />
<Button
android:id="@+id/audio_play_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="播放" />
<Button
android:id="@+id/audio_pause_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="暂停" />
<Button
android:id="@+id/audio_stop_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="停止" />
<Button
android:id="@+id/audio_replay_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="重播" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="视频播放" />
<EditText
android:id="@+id/video_path_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入视频文件路径" />
<Button
android:id="@+id/video_play_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="播放" />
<VideoView
android:id="@+id/video_view"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
</ScrollView>
```
阅读全文
相关推荐
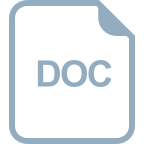
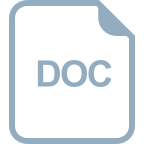
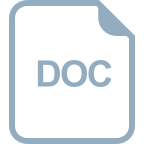
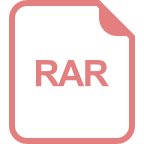
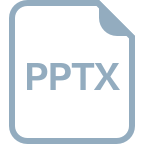
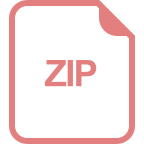
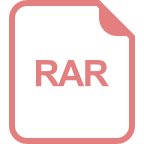
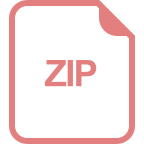
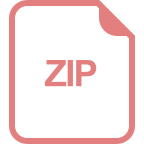
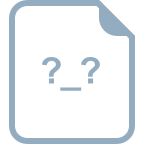
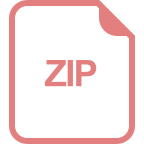
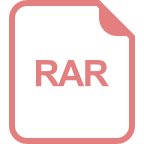
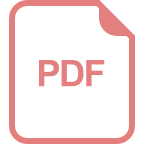
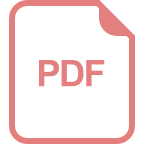
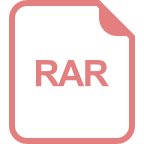
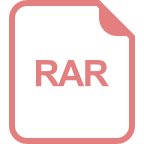
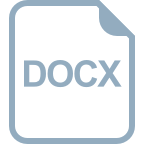
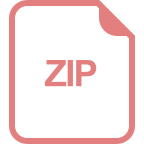