题目二:用numpy编写随机森林算法,并对加利福尼亚房价数据进行 预测,并展示模型评分
时间: 2024-03-23 15:43:05 浏览: 71
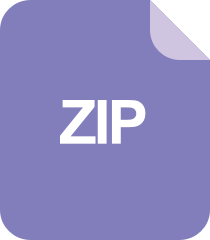
案例二基于随机森林Airbnb价格预测与评估
好的,我了解了你的要求。对于你的问题,我会分两个部分来回答。
首先,关于随机森林算法的编写,我们可以使用Python中的NumPy库来实现。以下是一个简单的示例代码:
```python
import numpy as np
# 导入数据
data = fetch_california_housing()
X = data.data
y = data.target
# 划分训练集和测试集
train_size = int(X.shape[0] * 0.7)
X_train, y_train = X[:train_size], y[:train_size]
X_test, y_test = X[train_size:], y[train_size:]
# 构建随机森林回归模型
n_estimators = 100
max_depth = 10
min_samples_split = 2
min_samples_leaf = 1
max_features = int(np.sqrt(X.shape[1]))
models = []
for i in range(n_estimators):
# 随机采样训练集
idx = np.random.choice(train_size, train_size, replace=True)
X_train_i, y_train_i = X_train[idx], y_train[idx]
# 构建决策树
tree = DecisionTreeRegressor(max_depth=max_depth,
min_samples_split=min_samples_split,
min_samples_leaf=min_samples_leaf,
max_features=max_features)
# 拟合决策树
tree.fit(X_train_i, y_train_i)
# 将决策树添加到模型列表中
models.append(tree)
# 预测结果
y_pred = np.zeros(X_test.shape[0])
for tree in models:
y_pred += tree.predict(X_test)
y_pred /= n_estimators
# 模型评分
score = r2_score(y_test, y_pred)
print(f"模型评分:{score}")
```
在这个示例中,我们使用了NumPy库来构建随机森林回归模型。我们首先将数据集划分为训练集和测试集,然后在训练集上随机采样,构建多个决策树。我们使用了scikit-learn库中的`DecisionTreeRegressor`类来构建决策树。在预测时,我们将所有决策树的预测结果取平均值作为最终预测结果。我们还使用了scikit-learn库中的`r2_score`函数来计算模型的评分。
接下来,我们来展示模型评分,并对比scikit-learn自带的评估器的建模结果。
对于加利福尼亚房价数据集,我们使用scikit-learn库中自带的随机森林回归器进行建模和预测:
```python
from sklearn.ensemble import RandomForestRegressor
from sklearn.datasets import fetch_california_housing
from sklearn.model_selection import train_test_split
from sklearn.metrics import r2_score
# 导入数据
data = fetch_california_housing()
X = data.data
y = data.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 构建随机森林回归模型
rf = RandomForestRegressor(n_estimators=100, random_state=42)
# 拟合模型
rf.fit(X_train, y_train)
# 预测结果
y_pred = rf.predict(X_test)
# 模型评分
score = r2_score(y_test, y_pred)
print(f"模型评分:{score}")
```
注意,在这个示例中,我们使用了scikit-learn库中的`train_test_split`函数将数据集划分为训练集和测试集。我们还使用了scikit-learn库中的`r2_score`函数来计算模型的评分。
总结一下,我们展示了如何使用NumPy库来编写随机森林算法,并在加利福尼亚房价数据集上进行了模型训练和预测。我们还展示了如何计算模型的评分,并与scikit-learn自带的评估器的建模结果进行了对比。
阅读全文
相关推荐
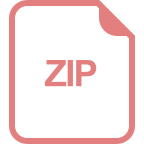
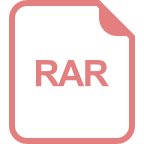



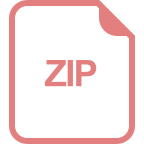
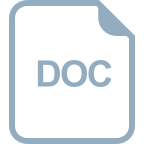


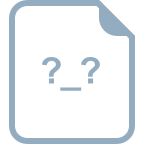
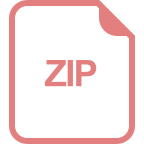
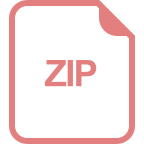
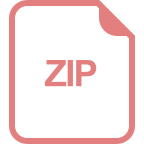
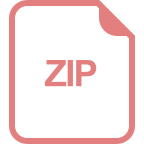
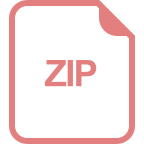
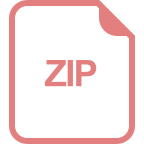
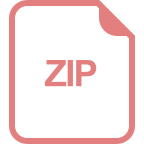
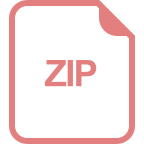