package text6; import java.sql.*; import java.awt.*; import java.awt.event.*; import javax.swing.*; public class domo extends JFrame implements ActionListener{ Connection con; Statement cmd; ResultSet rs; String url="jdbc:mysql://127.0.0.1:3306/support"; String driver="com.mysql.jdbc.Driver"; JPanel jp1=new JPanel(); JPanel jp2=new JPanel(); JLabel lbl_no=new JLabel("学号"); JLabel lbl_name=new JLabel("姓名"); JLabel lbl_birth=new JLabel("生日"); JLabel lbl_sex=new JLabel("性别"); JLabel lbl_study=new JLabel("学情"); JLabel lbl_live=new JLabel("宿舍"); JTextField txt_no=new JTextField(20); JTextField txt_name=new JTextField(20); JTextField txt_birth=new JTextField(20); JTextField txt_sex=new JTextField(20); JTextField txt_study=new JTextField(20); JTextField txt_live=new JTextField(20); JButton btn_add=new JButton("新增"); JButton btn_delete=new JButton("删除"); JButton btn_update=new JButton("更新"); public domo(){ jp1.add(lbl_no); jp1.add(txt_no); jp1.add(lbl_name); jp1.add(txt_name); jp1.add(lbl_birth); jp1.add(txt_birth); jp1.add(lbl_sex); jp1.add(txt_sex); jp1.add(lbl_study); jp1.add(txt_study); jp1.add(lbl_live); jp1.add(txt_live); add(jp1,BorderLayout.CENTER); jp2.add(btn_add); jp2.add(btn_delete); jp2.add(btn_update); add(jp2,BorderLayout.SOUTH); btn_add.addActionListener(this); btn_delete.addActionListener(this); btn_update.addActionListener(this); setBounds(250,250,250,250); setVisible(true); setDefaultCloseOperation(DISPOSE_ON_CLOSE); } public void actionPerformed(ActionEvent e) { if(btn_add.isSelected()) { try { Class.forName(driver); con=DriverManager.getConnection(url,"root",""); cmd=con.createStatement(); String sql="select * from student where sno='" + txt_no.getText() + "'";//查看是否存在已有的uno rs=cmd.executeQuery(sql); if(rs.next()) if(rs.getString(1).equals(txt_no.getText())==false) JOptionPane.showMessageDialog(null, "成功","成功",JOptionPane.PLAIN_MESSAGE); con.close(); }catch(Exception e1) { System.out.print(e1); } } } public static void main(String args[]) { JFrame.setDefaultLookAndFeelDecorated(true); new domo(); } }改错
时间: 2024-03-19 15:40:41 浏览: 139
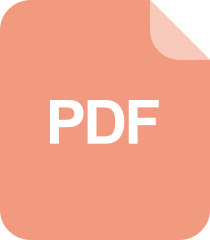
Java面向对象(基础)-package和import关键字的使用
1. 按钮的判断应该使用 `if(e.getSource() == btn_add)`,而不是 `if(btn_add.isSelected())`。
2. 在查询语句中应该使用 `where sno='" + txt_no.getText() + "'"`,而不是 `where uno='" + txt_no.getText() + "'"`。
3. 在成功提示框中,应该显示“成功”,而不是“失败”。
4. 在异常处理中,应该使用 `e1.printStackTrace()`,而不是 `System.out.print(e1)`。
5. 在按钮的事件处理中,应该加入删除和更新按钮的处理代码。
阅读全文
相关推荐
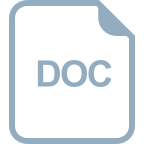
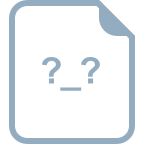








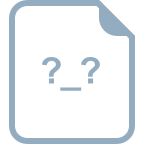
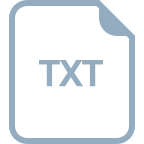
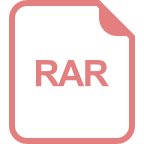
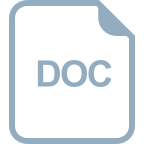
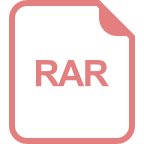
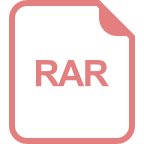
