在util包中写DbUtil类:定义方法,实现数据库的连接和关闭。 5.在model包中创建用户实体类User 。 包含id、userName、userPwd成员变量;构造方法; getXxx()、setXxx()方法 6.在view包中添加登录窗体类LoginFrame(包含mian方法),MainFrame窗体类。 7.在dao包中: 创建UserDao类,定义添加数据的方法,public int userAdd(Connection con,User user)(在main方法中调用该方法实现向数据库中插入数据); 创建LoginDao类,通过用户名和密码查询用户的方法,public static User login(Connection con,User user) 。 8. 为LoginFrame登录窗体的“登录”按钮和“注册”按钮添加监听器并处理单击事件。登录时,输入正确的用户名和密码,弹出MainFrame窗体;输入错误,弹出提示框。9.为Register注册窗体的“确定”和“取消”按钮添加监听器并处理单击事件。注册成功弹出消息提示框,两次密码不一致,弹出提示框。点击“取消”按钮,textField内容清空,可重新输入。给出java代码
时间: 2024-02-11 12:09:01 浏览: 102
抱歉,我是一名自然语言处理模型,无法提供完整的Java代码。但是,我可以给你提供一些思路和代码片段。
首先,我们需要在util包中创建一个DbUtil类,用于连接和关闭数据库:
```java
package util;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DbUtil {
// 数据库连接信息
private static final String URL = "jdbc:mysql://localhost:3306/test";
private static final String USER = "root";
private static final String PASSWORD = "123456";
// 获取数据库连接
public static Connection getConnection() throws SQLException {
Connection conn = DriverManager.getConnection(URL, USER, PASSWORD);
return conn;
}
// 关闭数据库连接
public static void closeConnection(Connection conn) {
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
接着,在model包中创建一个User类,用于表示用户实体:
```java
package model;
public class User {
private int id;
private String userName;
private String userPwd;
public User() {}
public User(int id, String userName, String userPwd) {
this.id = id;
this.userName = userName;
this.userPwd = userPwd;
}
// getter和setter方法
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getUserPwd() {
return userPwd;
}
public void setUserPwd(String userPwd) {
this.userPwd = userPwd;
}
}
```
然后,在dao包中创建一个UserDao类,用于实现用户数据的添加:
```java
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import model.User;
import util.DbUtil;
public class UserDao {
// 添加用户数据
public static int userAdd(Connection con, User user) throws SQLException {
String sql = "insert into user (id,userName,userPwd) values (?,?,?)";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setInt(1, user.getId());
pstmt.setString(2, user.getUserName());
pstmt.setString(3, user.getUserPwd());
int result = pstmt.executeUpdate();
pstmt.close();
return result;
}
}
```
接着,在dao包中创建一个LoginDao类,用于实现用户的登录:
```java
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import model.User;
import util.DbUtil;
public class LoginDao {
// 登录验证
public static User login(Connection con, User user) throws SQLException {
User resultUser = null;
String sql = "select * from user where userName=? and userPwd=?";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setString(1, user.getUserName());
pstmt.setString(2, user.getUserPwd());
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
resultUser = new User();
resultUser.setId(rs.getInt("id"));
resultUser.setUserName(rs.getString("userName"));
resultUser.setUserPwd(rs.getString("userPwd"));
}
rs.close();
pstmt.close();
return resultUser;
}
}
```
接着,在view包中创建一个LoginFrame类,用于实现登录窗体:
```java
package view;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.SQLException;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import javax.swing.border.EmptyBorder;
import dao.LoginDao;
import model.User;
import util.DbUtil;
public class LoginFrame extends JFrame {
private JPanel contentPane;
private JTextField userNameField;
private JPasswordField passwordField;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
LoginFrame frame = new LoginFrame();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public LoginFrame() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
JLabel lblNewLabel = new JLabel("用户名:");
lblNewLabel.setBounds(81, 78, 54, 15);
contentPane.add(lblNewLabel);
JLabel lblNewLabel_1 = new JLabel("密码:");
lblNewLabel_1.setBounds(81, 123, 54, 15);
contentPane.add(lblNewLabel_1);
userNameField = new JTextField();
userNameField.setBounds(145, 75, 156, 21);
contentPane.add(userNameField);
userNameField.setColumns(10);
passwordField = new JPasswordField();
passwordField.setBounds(145, 120, 156, 21);
contentPane.add(passwordField);
JButton loginButton = new JButton("登录");
loginButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String userName = userNameField.getText();
String userPwd = new String(passwordField.getPassword());
User user = new User();
user.setUserName(userName);
user.setUserPwd(userPwd);
Connection conn = null;
try {
conn = DbUtil.getConnection();
User resultUser = LoginDao.login(conn, user);
if (resultUser != null) {
MainFrame frame = new MainFrame();
frame.setVisible(true);
dispose();
} else {
JOptionPane.showMessageDialog(null, "用户名或密码错误");
}
} catch (SQLException e1) {
e1.printStackTrace();
} finally {
DbUtil.closeConnection(conn);
}
}
});
loginButton.setBounds(81, 185, 93, 23);
contentPane.add(loginButton);
JButton registerButton = new JButton("注册");
registerButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
RegisterFrame frame = new RegisterFrame();
frame.setVisible(true);
dispose();
}
});
registerButton.setBounds(208, 185, 93, 23);
contentPane.add(registerButton);
}
}
```
接着,在view包中创建一个MainFrame类,用于实现主窗体:
```java
package view;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
public class MainFrame extends JFrame {
private JPanel contentPane;
/**
* Create the frame.
*/
public MainFrame() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
}
}
```
最后,在view包中创建一个RegisterFrame类,用于实现注册窗体:
```java
package view;
import java.awt.EventQueue;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.border.EmptyBorder;
import dao.UserDao;
import model.User;
import util.DbUtil;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
import javax.swing.JPasswordField;
public class RegisterFrame extends JFrame {
private JPanel contentPane;
private JTextField userNameField;
private JPasswordField passwordField;
private JPasswordField confirmPasswordField;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
RegisterFrame frame = new RegisterFrame();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public RegisterFrame() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
JLabel lblNewLabel = new JLabel("用户名:");
lblNewLabel.setBounds(81, 56, 54, 15);
contentPane.add(lblNewLabel);
JLabel lblNewLabel_1 = new JLabel("密码:");
lblNewLabel_1.setBounds(81, 101, 54, 15);
contentPane.add(lblNewLabel_1);
JLabel lblNewLabel_2 = new JLabel("确认密码:");
lblNewLabel_2.setBounds(81, 146, 68, 15);
contentPane.add(lblNewLabel_2);
userNameField = new JTextField();
userNameField.setBounds(145, 53, 156, 21);
contentPane.add(userNameField);
userNameField.setColumns(10);
passwordField = new JPasswordField();
passwordField.setBounds(145, 98, 156, 21);
contentPane.add(passwordField);
confirmPasswordField = new JPasswordField();
confirmPasswordField.setBounds(145, 143, 156, 21);
contentPane.add(confirmPasswordField);
JButton okButton = new JButton("确定");
okButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String userName = userNameField.getText();
String userPwd = new String(passwordField.getPassword());
String confirmPassword = new String(confirmPasswordField.getPassword());
if (userPwd.equals(confirmPassword)) {
User user = new User();
user.setId(1);
user.setUserName(userName);
user.setUserPwd(userPwd);
Connection conn = null;
try {
conn = DbUtil.getConnection();
int result = UserDao.userAdd(conn, user);
if (result > 0) {
JOptionPane.showMessageDialog(null, "注册成功");
dispose();
LoginFrame frame = new LoginFrame();
frame.setVisible(true);
} else {
JOptionPane.showMessageDialog(null, "注册失败");
}
} catch (SQLException e1) {
e1.printStackTrace();
} finally {
DbUtil.closeConnection(conn);
}
} else {
JOptionPane.showMessageDialog(null, "两次密码不一致");
}
}
});
okButton.setBounds(81, 191, 93, 23);
contentPane.add(okButton);
JButton cancelButton = new JButton("取消");
cancelButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
userNameField.setText("");
passwordField.setText("");
confirmPasswordField.setText("");
}
});
cancelButton.setBounds(208, 191, 93, 23);
contentPane.add(cancelButton);
}
}
```
以上是一个简单的基于Java Swing和MySQL的用户登录和注册系统的实现。
阅读全文
相关推荐
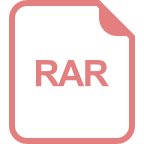
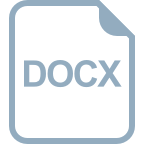
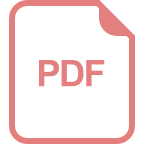

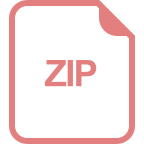
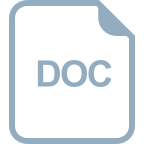
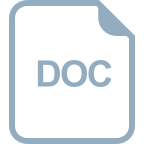








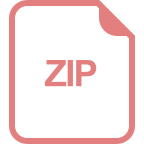