可不可以帮我写一串代码实现前端操作后端进行数据库增删改查,要求前后端分离
时间: 2024-09-06 14:01:44 浏览: 44
当然可以,这里我给出一个简化版的前后端分离示例,假设我们正在使用JavaScript (通过Fetch API) 进行前端操作,并使用Node.js + Express作为后端进行简单的数据库操作(例如MongoDB)。这是一个基本的增删改查操作的例子:
**后端 (Node.js with Express and Mongoose) - 服务器**
```javascript
const express = require('express');
const mongoose = require('mongoose');
// 连接数据库
mongoose.connect('mongodb://localhost/test', { useNewUrlParser: true });
// 假设有一个User模型
const UserSchema = new mongoose.Schema({ name: String });
const User = mongoose.model('User', UserSchema);
const app = express();
// CRUD操作
app.post('/users', async (req, res) => {
try {
const newUser = new User(req.body);
await newUser.save();
res.status(201).json(newUser);
} catch (error) {
res.status(400).json({ error: error.message });
}
});
// ...类似地,为其他CRUD操作创建相应路由
// 启动服务器
app.listen(3000, () => console.log('Server is running on port 3000'));
```
**前端 (HTML/JS with Fetch API) - 客户端**
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>CRUD Example</title>
</head>
<body>
<button id="addUser">Add User</button>
<script>
document.getElementById('addUser').addEventListener('click', async () => {
const newUser = {
name: 'John Doe'
};
// 发送POST请求
try {
const response = await fetch('http://localhost:3000/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(newUser)
});
if (!response.ok) throw new Error(response.statusText);
const data = await response.json();
console.log('User added:', data);
} catch (error) {
console.error('Error:', error);
}
});
</script>
</body>
</html>
```
这只是一个基础示例,实际应用中还需要考虑更多的细节,如错误处理、数据验证、状态管理等。同时,根据你的后端框架和技术栈,上述代码可能会有所不同。
阅读全文
相关推荐
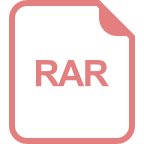
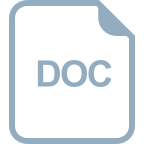
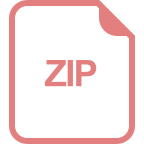
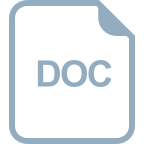
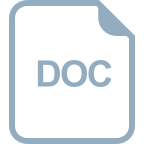
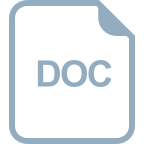
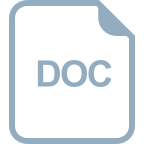
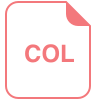
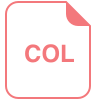
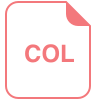
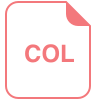
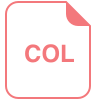
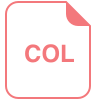
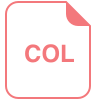
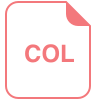
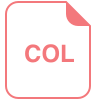
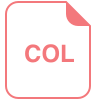
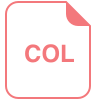
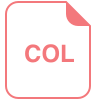