c语言直接调用libcurl库,实现http ping服务端和客户端功能,支持探测次数、探测周期配置
时间: 2024-06-08 21:11:15 浏览: 14
要实现C语言调用libcurl库实现HTTP ping服务端和客户端功能,需要进行以下步骤:
1. 安装libcurl库
首先需要安装libcurl库,可以在终端中使用以下命令进行安装:
```
sudo apt-get install libcurl4-openssl-dev
```
2. 编写服务端程序
服务端程序需要监听HTTP请求并返回响应,可以使用libcurl库中提供的curl_easy_setopt函数设置参数,如下所示:
```c
#include <curl/curl.h>
int main(void)
{
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://localhost:8080/ping");
curl_easy_setopt(curl, CURLOPT_RETURNTRANSFER, 1L);
curl_easy_setopt(curl, CURLOPT_HTTPGET, 1L);
res = curl_easy_perform(curl);
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
curl_easy_cleanup(curl);
}
return 0;
}
```
上述代码中,使用curl_easy_setopt函数设置了URL、返回响应的选项和使用HTTP GET方法发送请求的选项。在使用curl_easy_perform函数执行请求后,可以通过返回值res判断请求是否成功。
3. 编写客户端程序
客户端程序需要定时向服务端发送HTTP请求,并记录响应时间。可以使用C语言中的time函数获取当前时间,并使用curl_easy_setopt函数设置超时时间,如下所示:
```c
#include <curl/curl.h>
#include <time.h>
int main(void)
{
CURL *curl;
CURLcode res;
long response_code;
double elapsed_time;
time_t start_time, end_time;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://localhost:8080/ping");
curl_easy_setopt(curl, CURLOPT_RETURNTRANSFER, 1L);
curl_easy_setopt(curl, CURLOPT_HTTPGET, 1L);
curl_easy_setopt(curl, CURLOPT_TIMEOUT_MS, 1000L);
while(1) {
start_time = time(NULL);
res = curl_easy_perform(curl);
end_time = time(NULL);
elapsed_time = difftime(end_time, start_time);
if(res == CURLE_OK) {
curl_easy_getinfo(curl, CURLINFO_RESPONSE_CODE, &response_code);
printf("Response code: %ld\n", response_code);
printf("Elapsed time: %f\n", elapsed_time);
} else {
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
}
sleep(1);
}
curl_easy_cleanup(curl);
}
return 0;
}
```
上述代码中,使用curl_easy_setopt函数设置了URL、返回响应的选项、使用HTTP GET方法发送请求的选项和超时时间。在使用curl_easy_perform函数执行请求后,可以通过curl_easy_getinfo函数获取响应码和响应时间,并使用printf函数输出。
4. 配置探测次数和探测周期
为了支持探测次数和探测周期的配置,可以使用C语言中的命令行参数进行传递。修改客户端程序如下:
```c
#include <curl/curl.h>
#include <time.h>
#include <stdlib.h>
int main(int argc, char *argv[])
{
CURL *curl;
CURLcode res;
long response_code;
double elapsed_time;
time_t start_time, end_time;
int num_pings = 10;
int ping_interval = 1;
if(argc >= 2) {
num_pings = atoi(argv[1]);
}
if(argc >= 3) {
ping_interval = atoi(argv[2]);
}
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://localhost:8080/ping");
curl_easy_setopt(curl, CURLOPT_RETURNTRANSFER, 1L);
curl_easy_setopt(curl, CURLOPT_HTTPGET, 1L);
curl_easy_setopt(curl, CURLOPT_TIMEOUT_MS, 1000L);
for(int i = 0; i < num_pings; i++) {
start_time = time(NULL);
res = curl_easy_perform(curl);
end_time = time(NULL);
elapsed_time = difftime(end_time, start_time);
if(res == CURLE_OK) {
curl_easy_getinfo(curl, CURLINFO_RESPONSE_CODE, &response_code);
printf("Response code: %ld\n", response_code);
printf("Elapsed time: %f\n", elapsed_time);
} else {
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
}
sleep(ping_interval);
}
curl_easy_cleanup(curl);
}
return 0;
}
```
上述代码中,使用命令行参数设置探测次数和探测周期,并在循环中使用sleep函数等待下一次探测。
使用以下命令编译客户端程序:
```
gcc -o ping_client ping_client.c -lcurl
```
使用以下命令运行客户端程序:
```
./ping_client 10 2
```
上述命令将会进行10次探测,每次间隔2秒。
相关推荐
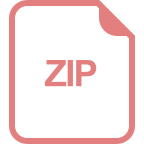














