this.$notify onClick
时间: 2024-07-13 12:01:11 浏览: 57
在 Vue.js 中,`this.$notify` 是一个通知组件或通知提示功能,通常用于向用户显示临时的消息或警告,而 `onClick` 是点击事件处理程序。当你设置 `onClick` 属性时,可以在用户点击通知时执行自定义的操作。
例如:
```javascript
this.$notify({
title: '警告',
message: '这是一个通知',
type: 'error', // 可选类型如 'success', 'warning', 'info'
duration: 3000, // 显示时间,单位为毫秒
position: 'top-center', // 提示的位置
onClick: function (event) {
// 当用户点击通知时,执行这个回调函数
console.log('点击了通知');
// 或者在这里触发其他业务操作
}
});
```
在这个例子中,当用户点击通知时,控制台会打印 "点击了通知",并且可以根据需要执行额外的代码。
相关问题--
1. 在Vue中,$notify通常用于什么场景?
2. 如何在VUE的$notify中添加点击事件处理?
3. $notify中的onClick属性能否阻止默认行为?
相关问题
org.openqa.selenium.ElementClickInterceptedException: element click intercepted: Element <input class="codeno" name="Currency1" id="Currency1" style="background: url(../common/images/select--bg_03.png) no-repeat center right; " onclick="return showCodeList('currency',[this,CurrencyName1],[0,1]);" ondblclick="return showCodeList('currency',[this,CurrencyName1],[0,1]);" onkeyup="return showCodeListKey('currency',[this,CurrencyName1],[0,1]);" autocomplete="off"> is not clickable at point (157, 600). Other element would receive the click: <option value="2">...</option> (Session info: chrome=114.0.5735.110) Build info: version: '3.141.59', revision: 'e82be7d358', time: '2018-11-14T08:17:03' System info: host: 'NB-CD-237', ip: '10.10.14.2', os.name: 'Windows 10', os.arch: 'amd64', os.version: '10.0', java.version: '1.8.0_351' Driver info: org.openqa.selenium.chrome.ChromeDriver Capabilities {acceptInsecureCerts: false, browserName: chrome, browserVersion: 114.0.5735.110, chrome: {chromedriverVersion: 114.0.5735.90 (386bc09e8f4f..., userDataDir: C:\Users\HZ2211~1\AppData\L...}, goog:chromeOptions: {debuggerAddress: localhost:50094}, javascriptEnabled: true, networkConnectionEnabled: false, pageLoadStrategy: normal, platform: WINDOWS, platformName: WINDOWS, proxy: Proxy(), setWindowRect: true, strictFileInteractability: false, timeouts: {implicit: 0, pageLoad: 300000, script: 30000}, unhandledPromptBehavior: dismiss and notify, webauthn:extension:credBlob: true, webauthn:extension:largeBlob: true, webauthn:extension:minPinLength: true, webauthn:extension:prf: true, webauthn:virtualAuthenticators: true} Session ID: 573972bafc2464b48b20cd585953448e
这是一个 Selenium 的异常,错误信息是元素无法被点击。具体原因是该元素被其他元素遮挡了,无法直接点击。您可以尝试使用 Selenium 的 Actions 类来模拟鼠标点击,或者使用 JavaScript 脚本来执行点击操作。另外,您也可以尝试等待页面加载完全后再进行点击操作,或者调整页面布局使得该元素可见并可点击。
android推送弹窗,android – 在推送通知中显示活动/弹出窗口,而不是状态栏中的消息...
您可以使用Android系统提供的弹出窗口或者Dialog来实现推送通知中显示活动/弹出窗口。
在您的应用程序中,您可以使用NotificationCompat.Builder来创建通知,并使用setFullScreenIntent方法将Intent设置为显示全屏通知。这将在用户单击通知时显示全屏通知。示例代码如下:
```
// Create an Intent for the activity you want to display
Intent intent = new Intent(this, YourActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT);
// Build the notification
NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID)
.setSmallIcon(R.drawable.notification_icon)
.setContentTitle("My notification")
.setContentText("Hello World!")
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.setContentIntent(pendingIntent)
.setAutoCancel(true)
.setFullScreenIntent(pendingIntent, true);
// Show the notification
NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this);
notificationManager.notify(notificationId, builder.build());
```
您也可以使用Dialog来实现弹窗效果。在您的应用程序中,您可以使用AlertDialog.Builder来创建对话框,并使用setView方法将您的布局设置为对话框的视图。示例代码如下:
```
// Create a builder for the dialog
AlertDialog.Builder builder = new AlertDialog.Builder(this);
// Set the title and message for the dialog
builder.setTitle("My Dialog");
builder.setMessage("Hello World!");
// Inflate your custom layout into the dialog's view
LayoutInflater inflater = getLayoutInflater();
View dialogView = inflater.inflate(R.layout.my_dialog_layout, null);
builder.setView(dialogView);
// Add buttons to the dialog
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// Do something when the user clicks OK
}
});
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// Do something when the user clicks Cancel
}
});
// Show the dialog
AlertDialog dialog = builder.create();
dialog.show();
```
希望这可以帮到您!
相关推荐
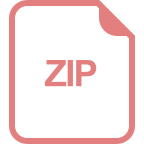
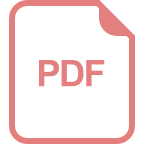
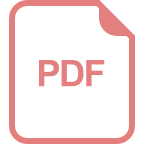













