C++编程实现:模拟计算机进行进制转换的过程。从键盘输入一个二进制整数(不超过10位),将其转换成十进制整数并输出结果。要求使用while语句
时间: 2024-10-28 08:07:18 浏览: 37
在C++中,我们可以使用`cin`从键盘读取用户输入的二进制整数,并利用`while`循环结合计算来完成进制转换。以下是一个简单的示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
// Function to convert a binary number into decimal
int binaryToDecimal(string binary) {
int decimal = 0;
int power = 0; // Power of 2 starting from rightmost digit
while (!binary.empty()) {
char currentChar = binary.back();
binary.pop_back();
if (currentChar == '1') {
decimal += pow(2, power);
}
power++;
}
return decimal;
}
int main() {
string binaryInput;
cout << "Enter a binary number (up to 10 digits): ";
cin >> binaryInput;
// Ensure the input is valid
while (binaryInput.length() > 10 || (binaryInput.size() == 10 && binaryInput[0] != '1')) {
cout << "Invalid binary number! Please enter up to 10 digits, starting with '1': ";
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Clear the invalid input
getline(cin, binaryInput);
}
// Convert binary to decimal and output
int decimalValue = binaryToDecimal(binaryInput);
cout << "The decimal equivalent of " << binaryInput << " is: " << decimalValue << endl;
return 0;
}
```
这个程序首先提示用户输入一个二进制数,如果输入不符合规定(超过10位或非纯二进制数),则会清除无效输入并重新请求。一旦输入有效,就调用`binaryToDecimal`函数将二进制转换成十进制。
阅读全文
相关推荐
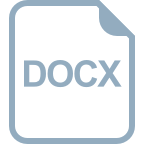
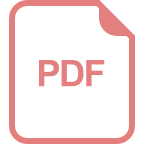
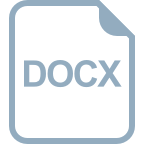

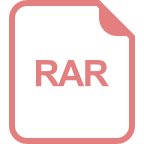
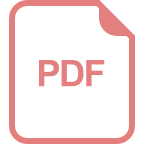
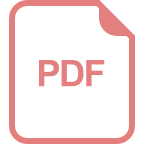
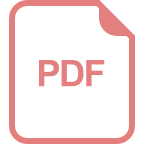
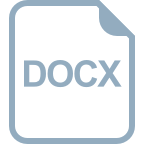
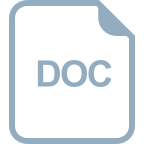
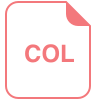
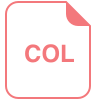
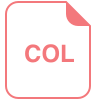
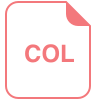
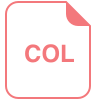
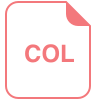
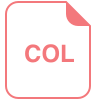
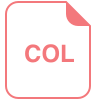
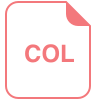