用c写一个函数,生成256位、64位、42位、16位四个16进制随机数的函数并且不使用printf
时间: 2023-11-28 09:48:22 浏览: 72
以下是生成四个不同长度的随机16进制数的C函数代码,其中使用了rand()函数来生成随机数:
```c
#include <stdlib.h>
#include <time.h>
// 生成256位16进制随机数
void generate256(char *result) {
srand(time(NULL));
for (int i = 0; i < 64; i++) {
int rand_num = rand() % 16;
if (rand_num < 10) {
result[i] = rand_num + '0';
} else {
result[i] = rand_num - 10 + 'a';
}
}
}
// 生成64位16进制随机数
void generate64(char *result) {
srand(time(NULL));
for (int i = 0; i < 16; i++) {
int rand_num = rand() % 16;
if (rand_num < 10) {
result[i] = rand_num + '0';
} else {
result[i] = rand_num - 10 + 'a';
}
}
}
// 生成42位16进制随机数
void generate42(char *result) {
srand(time(NULL));
for (int i = 0; i < 11; i++) {
int rand_num = rand() % 16;
if (rand_num < 10) {
result[i] = rand_num + '0';
} else {
result[i] = rand_num - 10 + 'a';
}
}
}
// 生成16位16进制随机数
void generate16(char *result) {
srand(time(NULL));
for (int i = 0; i < 4; i++) {
int rand_num = rand() % 16;
if (rand_num < 10) {
result[i] = rand_num + '0';
} else {
result[i] = rand_num - 10 + 'a';
}
}
}
```
调用方法如下:
```c
char result[65]; // 存放256位16进制随机数
generate256(result);
result[64] = '\0'; // 字符串末尾加上'\0',变成字符串形式
char result2[17]; // 存放16位16进制随机数
generate16(result2);
result2[16] = '\0'; // 字符串末尾加上'\0',变成字符串形式
// 其余两个长度的随机数同理
```
阅读全文
相关推荐
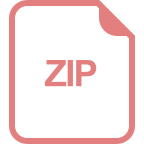
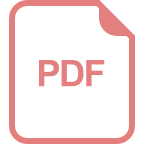
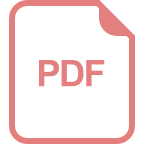







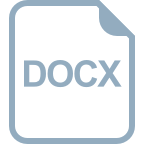
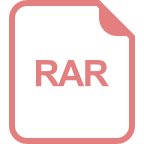
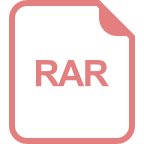
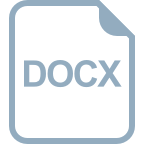
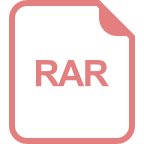
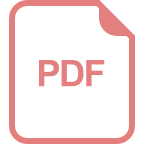
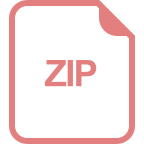