C# 从某个进程的内存中获取控件中的图片
时间: 2024-10-06 08:01:23 浏览: 28
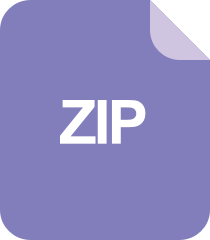
C# 进程,内存,文件管理详细解答
在C#中,如果你想要从另一个进程的内存中获取控件中的图片,通常情况下这涉及到远程过程调用(RPC)、进程间通信(IPC),或者通过某些底层API(如PInvoke)。但需要注意的是,这种操作可能会涉及权限问题、安全限制,并且不是常规的应用程序设计实践。
下面是一个基本的示例,展示了如何使用`System.Drawing.Imaging`库和`Marshal`类来读取另一个进程的内存中存储的图像数据:
```csharp
using System;
using System.Runtime.InteropServices;
using System.Drawing;
class Program
{
[DllImport("user32.dll", SetLastError = true)]
static extern IntPtr GetWindowDC(IntPtr hWnd);
[DllImport("gdi32.dll")]
static extern int GetDIBits(IntPtr hdc, IntPtr hBitmap, uint nStartScan, uint nScanCount,
byte[] lpBits, IntRect lprcBounds, DibAttributes dwFlags);
// 假设你已经获得了需要进程ID和窗口句柄
private const int PROCESS_ID = YourProcessId;
private IntPtr hwnd = YourTargetWindowHandle;
public static void Main(string[] args)
{
IntPtr handle = Process.GetProcessById(PROCESS_ID).MainWindowHandle; // 获取目标进程的主线程窗口
if (handle == IntPtr.Zero) throw new Exception("Failed to get window handle");
try
{
// 获取目标窗口的设备上下文(DC)
IntPtr hdc = GetWindowDC(handle);
if (hdc == IntPtr.Zero) throw new Exception("Failed to get device context");
// 假设图片存储在窗体的一个位图控件中,例如pictureBox1.Image
Image image = LoadImageFromMemory(hdc);
// 现在你可以处理image对象了
}
finally
{
ReleaseDC(hwnd, hdc); // 释放资源
}
}
// 使用GetDIBits从DC中加载图像数据到byte数组
private static Image LoadImageFromMemory(IntPtr hdc)
{
// 假设我们有位图宽度和高度
int width = YourBitmapWidth;
int height = YourBitmapHeight;
byte[] imageData = new byte[width * height * 4]; // 位深度为32bit的Bgra格式
var rect = new Int32Rect(0, 0, width, height);
GetDIBits(hdc, IntPtr.Zero, 0, height, imageData, rect, DibAccess.DibReadPixels);
// 将字节数组转换为Image对象
return Image.FromHbitmap(Marshal.AllocHbitmap(width, height, Imaging.ColorDepth.Depth32Bit, IntPtr.Zero, imageData, IntPtr.Zero));
}
}
```
注意这个例子假设你在目标进程中找到了一个位图控件并且它已经被绘制到了DC上。实际操作可能需要更复杂的交互逻辑和错误处理。同时,这个示例仅适用于Windows环境下的.NET应用。
阅读全文
相关推荐
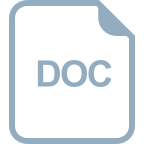
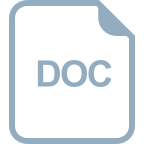
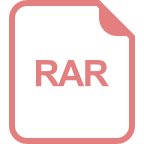
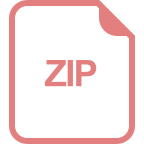
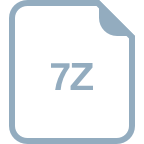
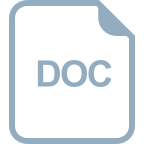
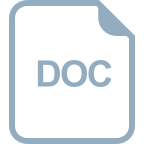
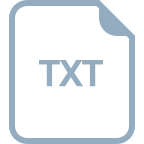
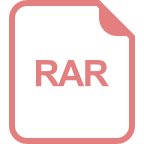
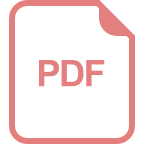
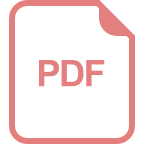
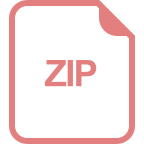
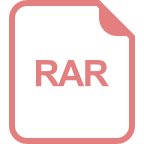
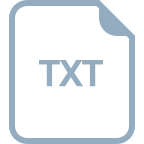
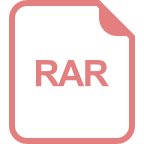
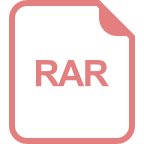
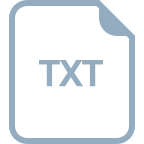
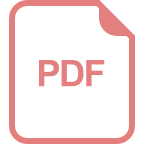