#第四部分 旋转图片 from PIL import Image, ImageDraw # 将图片平移并旋转 gray2 = Image.fromarray(src) width, height = gray2.size # 计算中心点和X轴角度 center = (max_point[0], max_point[1]) angle = np.arctan2(point2[1] - max_point[1], point2[0] - max_point[0]) * 180 / np.pi img_translated = gray2.transform((width, height), Image.AFFINE, (1, 0, center[0] - width/2, 0, 1, center[1] - height/2), resample=Image.BICUBIC) img_translated_rotated = img_translated.rotate(angle, resample=Image.BICUBIC, expand=True) #img_translated_rotated.show() import cv2 GRID_STEP = distance/2 # 设置10*10栅格(暂时尝试) grid_num_x = 10 grid_num_y = 10 def transform_point_set(points, max_point, distance, angle): # 平移向量 translation_vector = np.array([distance * np.cos(angle*np.pi/180), distance * np.sin(angle*np.pi/180)]) # 旋转矩阵 rotation_matrix = np.array([[np.cos(angle*np.pi/180), -np.sin(angle*np.pi/180)], [np.sin(angle*np.pi/180), np.cos(angle*np.pi/180)]]) # 将点集转换为 numpy 数组 point_array = np.array(points) max_point_array = np.array(max_point) # 对点集进行平移和旋转 point_array = (point_array - max_point_array) @ rotation_matrix + max_point_array + translation_vector # 将 numpy 数组转换为列表 points2 = point_array.tolist() return points2 操作之后点和再图上原本的位置不再重合,请分析原因
时间: 2023-06-18 22:08:27 浏览: 66
经过平移和旋转操作后,图像的像素位置发生了变化,导致点集与原本的位置不再重合。这是因为平移和旋转操作会改变图像的坐标系和像素位置,因此点集也需要进行相应的坐标变换才能与图像对应。在代码中,函数 transform_point_set 实现了对点集进行平移和旋转变换的操作,但可能存在一些细节问题导致变换不准确,需要进一步检查和调试。
相关问题
from PIL import Image, ImageDraw # 将图片平移并旋转 gray2 = Image.fromarray(src) width, height = gray2.size # 计算中心点和X轴角度 center = (max_point[0], max_point[1]) angle = np.arctan2(point2[1] - max_point[1], point2[0] - max_point[0]) * 180 / np.pi img_translated = gr
ay2.transform((width, height), Image.AFFINE, (1, 0, -center[0], 0, 1, -center[1])) # 平移图片
img_rotated = img_translated.rotate(angle) # 旋转图片
draw = ImageDraw.Draw(img_rotated) # 获取绘图对象
draw.line([max_point, point2], fill='red', width=2) # 绘制直线
img_rotated.show() # 显示处理后的图片
以上代码是对一张图片进行平移和旋转,并在图片上绘制一条直线。首先利用PIL库中的Image类将数组转换成图片对象,然后计算中心点和旋转角度,利用transform()方法平移图片,利用rotate()方法旋转图片,最后利用Draw类绘制直线。最后通过show()方法显示处理后的图片。
from PIL import Image, ImageDraw, ImageFont
这是Python中使用Pillow库来操作图像的代码。它可以让你打开、处理和保存图像。
首先,我们需要导入Pillow库中的Image、ImageDraw和ImageFont模块。
```python
from PIL import Image, ImageDraw, ImageFont
```
然后,我们可以使用Image模块的open()方法来打开一张图片。
```python
im = Image.open("example.png")
```
接下来,我们可以使用ImageDraw模块创建一个可以绘制到图像上的对象。
```python
draw = ImageDraw.Draw(im)
```
我们可以使用这个对象来绘制各种形状和文本。例如,我们可以使用它的line()方法来绘制一条直线。
```python
draw.line((0, 0, im.width, im.height), fill=128)
```
最后,我们可以使用ImageFont模块创建一个字体对象,并将其传递给draw对象的text()方法来绘制文本。
```python
font = ImageFont.truetype("arial.ttf", 16)
draw.text((10, 10), "Hello, world!", font=font, fill=128)
```
最后,我们可以使用Image对象的save()方法将修改后的图像保存到文件中。
```python
im.save("output.png")
```
相关推荐
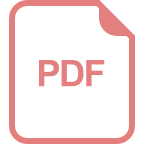
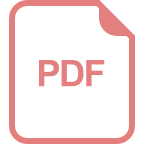
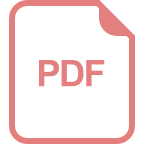












