android textview 滚动颜色渐变
时间: 2023-07-28 10:17:57 浏览: 46
要实现`TextView`文字滚动时颜色渐变的效果,可以使用`LinearGradient`和`Matrix`来实现。
具体实现步骤如下:
1. 定义一个`LinearGradient`对象,用于存储渐变颜色信息。
```java
int[] colors = {Color.BLACK, Color.WHITE, Color.BLACK}; // 定义渐变颜色
float[] positions = {0f, 0.5f, 1f}; // 定义渐变颜色位置
LinearGradient gradient = new LinearGradient(0, 0, textView.getWidth(), 0, colors, positions, Shader.TileMode.CLAMP); // 创建线性渐变对象
```
2. 定义一个`Matrix`对象,用于控制文字的绘制。
```java
Matrix matrix = new Matrix();
matrix.setTranslate(textView.getWidth(), 0); // 设置初始位置
```
3. 在`TextView`的`onDraw()`方法中,使用`Paint`对象设置渐变和矩阵,并调用`canvas.drawText()`方法绘制文字。
```java
Paint paint = textView.getPaint();
paint.setShader(gradient);
paint.getShader().setLocalMatrix(matrix);
canvas.drawText(textView.getText().toString(), 0, textView.getHeight() / 2, paint);
```
4. 在`TextView`的`onSizeChanged()`方法中,重新设置`LinearGradient`和`Matrix`对象的参数。
```java
@Override
protected void onSizeChanged(int w, int h, int oldw, int oldh) {
super.onSizeChanged(w, h, oldw, oldh);
int[] colors = {Color.BLACK, Color.WHITE, Color.BLACK};
float[] positions = {0f, 0.5f, 1f};
gradient = new LinearGradient(0, 0, w, 0, colors, positions, Shader.TileMode.CLAMP);
matrix = new Matrix();
matrix.setTranslate(w, 0);
}
```
完整代码示例:
```java
public class GradientTextView extends androidx.appcompat.widget.AppCompatTextView {
private LinearGradient gradient;
private Matrix matrix;
public GradientTextView(Context context) {
super(context);
}
public GradientTextView(Context context, AttributeSet attrs) {
super(context, attrs);
}
public GradientTextView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
}
@Override
protected void onDraw(Canvas canvas) {
Paint paint = getPaint();
paint.setShader(gradient);
paint.getShader().setLocalMatrix(matrix);
canvas.drawText(getText().toString(), 0, getHeight() / 2, paint);
invalidate();
}
@Override
protected void onSizeChanged(int w, int h, int oldw, int oldh) {
int[] colors = {Color.BLACK, Color.WHITE, Color.BLACK};
float[] positions = {0f, 0.5f, 1f};
gradient = new LinearGradient(0, 0, w, 0, colors, positions, Shader.TileMode.CLAMP);
matrix = new Matrix();
matrix.setTranslate(w, 0);
}
}
```
使用方法:
```xml
<com.example.GradientTextView
android:id="@+id/gradient_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/lorem_ipsum"
android:textColor="@android:color/black"
android:textSize="24sp" />
```
相关推荐
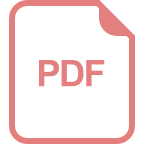














