vue中使用RabbitMQ,请给出示例代码
时间: 2023-09-06 22:04:01 浏览: 87
### 回答1:
在 Vue 项目中使用 RabbitMQ 的示例代码如下:
1. 安装 amqplib
```
npm install amqplib
```
2. 在 main.js 中引入 amqplib
```javascript
import amqp from 'amqplib/callback_api'
```
3. 在 Vue 实例中声明一个连接 RabbitMQ 的方法
```javascript
methods: {
connectToRabbitMQ() {
amqp.connect('amqp://localhost', (error0, connection) => {
if (error0) {
throw error0;
}
connection.createChannel((error1, channel) => {
if (error1) {
throw error1;
}
var queue = 'hello';
var msg = 'Hello World!';
channel.assertQueue(queue, {
durable: false
});
channel.sendToQueue(queue, Buffer.from(msg));
console.log(" [x] Sent %s", msg);
});
setTimeout(() => {
connection.close();
process.exit(0);
}, 500);
});
}
}
```
4. 在 Vue 组件中调用该方法
```javascript
mounted() {
this.connectToRabbitMQ();
}
```
请注意,以上代码只是示例,您可能需要根据实际需求进行修改。
### 回答2:
Vue中使用RabbitMQ需要结合后端框架进行实现,例如使用Node.js和Express来搭建后端服务。以下是一个使用Vue和RabbitMQ的示例代码:
后端代码(使用Node.js和Express):
```
const express = require('express');
const amqp = require('amqplib');
const app = express();
const port = 3000;
app.use(express.json());
// 定义RabbitMQ连接信息
const mqHost = 'localhost';
const mqUser = 'guest';
const mqPassword = 'guest';
const mqExchange = 'myExchange';
// 创建消息队列连接
async function createConnection() {
const connection = await amqp.connect(`amqp://${mqUser}:${mqPassword}@${mqHost}`);
const channel = await connection.createChannel();
// 创建一个名为myQueue的队列
await channel.assertQueue('myQueue');
// 绑定消息队列到指定的exchange
await channel.bindQueue('myQueue', mqExchange, '');
return channel;
}
app.post('/send', async (req, res) => {
try {
const message = req.body.message;
// 创建一个RabbitMQ连接
const channel = await createConnection();
// 发送消息到myQueue队列
channel.sendToQueue('myQueue', Buffer.from(message));
res.status(200).send('Message sent successfully');
} catch (error) {
res.status(500).send('Failed to send message');
}
});
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
```
前端代码(使用Vue):
```
<template>
<div>
<input v-model="message" placeholder="Message" />
<button @click="sendMessage">Send</button>
</div>
</template>
<script>
export default {
data() {
return {
message: '',
};
},
methods: {
sendMessage() {
const data = { message: this.message };
// 发送POST请求到后端服务
fetch('/send', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(data),
})
.then((response) => response.text())
.then((result) => {
console.log(result);
// 处理发送成功的逻辑
})
.catch((error) => {
console.error(error);
// 处理发送失败的逻辑
});
},
},
};
</script>
```
在上述示例中,前端通过点击按钮发送消息到后端的`/send`接口。后端会创建一个RabbitMQ连接,并将消息发送到名为`myQueue`的队列中。
### 回答3:
在Vue中使用RabbitMQ,可以使用RabbitMQ.js库来实现与RabbitMQ的通信。下面是一个简单的Vue示例代码,演示了如何使用RabbitMQ.js库来发送和接收消息:
```javascript
<template>
<div>
<button @click="sendMessage">发送消息</button>
<div v-for="message in receivedMessages" :key="message">
{{ message }}
</div>
</div>
</template>
<script>
import amqp from 'amqplib';
export default {
data() {
return {
receivedMessages: []
}
},
methods: {
async sendMessage() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'my_queue';
const message = 'Hello RabbitMQ!';
await channel.assertQueue(queue);
channel.sendToQueue(queue, Buffer.from(message));
await channel.close();
await connection.close();
}
},
mounted() {
this.receiveMessage();
},
async receiveMessage() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'my_queue';
await channel.assertQueue(queue);
channel.consume(queue, (msg) => {
this.receivedMessages.push(msg.content.toString());
});
await channel.close();
await connection.close();
}
}
</script>
```
上面的代码中,我们首先导入了amqplib库,并创建了一个Vue组件。组件内部定义了一个用于存储接收到的消息的数据属性receivedMessages,以及两个方法sendMessage和receiveMessage。
sendMessage方法用于发送消息,首先通过connect方法连接到RabbitMQ服务器并创建一个消息通道。然后声明了一个队列名为'my_queue',并将消息'Hello RabbitMQ!'发送到该队列。最后关闭消息通道和连接。
receiveMessage方法用于接收消息,同样地通过connect方法连接到RabbitMQ服务器并创建一个消息通道。然后声明了一个名为'my_queue'的队列,并通过channel.consume方法注册了一个消费者函数,用于接收队列中的消息。当接收到消息后,将其转化为字符串并存储到receivedMessages数组中。最后关闭消息通道和连接。
在mounted生命周期钩子中调用receiveMessage方法,以便组件挂载后能够开始接收消息。
需要在项目中安装amqplib库,可以通过npm install amqplib命令进行安装。请注意,上述代码仅为示例,在实际应用中,您需要根据自己的需求进行修改和适配。
相关推荐
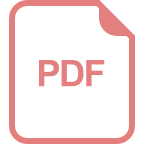














