编写函数,求给定字符串中数字字符的个数,在主函数中输入字符串及输出统计的个数。
时间: 2024-05-14 18:16:02 浏览: 28
以下是 Python 代码实现:
```python
def count_digits(s):
count = 0
for c in s:
if c.isdigit():
count += 1
return count
if __name__ == '__main__':
s = input('请输入字符串:')
print('数字字符的个数为:', count_digits(s))
```
在主函数中,我们可以通过 `input` 函数获取用户输入的字符串,然后调用 `count_digits` 函数统计数字字符的个数并输出。`count_digits` 函数中,我们遍历字符串中的每个字符,如果该字符是数字字符,就将计数器加 1,最后返回计数器的值。
相关问题
编写函数求给定字符串中数字字符的个数在主函数中输入字符串及输出统计的个数
以下是 Python 的示例代码:
```python
def count_digits(s):
count = 0
for c in s:
if c.isdigit():
count += 1
return count
# 示例用法
s = input("请输入一个字符串:")
count = count_digits(s)
print("字符串中数字字符的个数为:", count)
```
解释一下代码:
- `count_digits` 函数接受一个字符串参数 `s`,用 `count` 变量来记录其中数字字符的数量。
- 使用 `for` 循环遍历字符串中的每个字符,对于每个字符,使用 `isdigit()` 方法来判断是否是数字字符,如果是,则将计数器 `count` 加 1。
- 循环结束后,返回数字字符的数量。
- 在主函数中,首先让用户输入一个字符串,然后调用 `count_digits` 函数来统计其中数字字符的数量,并输出结果。
注意,这里的示例代码假设输入的字符串只包含 ASCII 编码下的数字字符。如果要处理 Unicode 编码下的数字字符,需要使用 `isnumeric()` 方法来判断。
编写函数,求给定字符串中数字字符的个数,在主函数中输入字符串及输出统计的个数。c语言
以下是 C 语言的实现:
```c
#include <stdio.h>
int countNumbers(char *str) {
int count = 0;
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] >= '0' && str[i] <= '9') {
count++;
}
}
return count;
}
int main() {
char str[100];
printf("请输入字符串:");
scanf("%s", str);
int count = countNumbers(str);
printf("字符串中数字字符的个数为:%d\n", count);
return 0;
}
```
以上代码中,`countNumbers` 函数统计给定字符串中数字字符的个数,`main` 函数则获取用户输入的字符串并输出统计的结果。如果字符串中的字符是数字字符,即 ASCII 码为 `'0'` 到 `'9'` 之间,则计数器加 1。
阅读全文
相关推荐












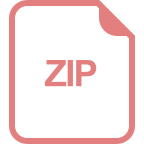